by gerson3004mn
Occupational Health and Safety Equipment Management System
from flask import Flask, url_for, request, session
from gunicorn.app.base import BaseApplication
from models.models import db, User
from abilities import apply_sqlite_migrations
import logging
# Setup logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
def create_app():
app = Flask(__name__, static_folder='static')
app.secret_key = 'supersecretkey'
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///database.sqlite'
db.init_app(app)
with app.app_context():
apply_sqlite_migrations(db.engine, db.Model, 'migrations')
# Import blueprints here to avoid circular imports
from routes import routes as routes_blueprint
from authentication import auth, auth_required
app.register_blueprint(routes_blueprint)
Frequently Asked Questions
How can the Occupational Health and Safety Equipment Management System benefit a company's safety program?
The Occupational Health and Safety Equipment Management System can significantly enhance a company's safety program by providing a centralized platform for tracking and managing safety equipment. It allows for efficient inventory management, ensuring that all necessary safety gear is available and in good condition. The system also helps in monitoring equipment loans, which can improve accountability and reduce loss. By maintaining accurate records of equipment purchase dates and status, companies can stay compliant with safety regulations and schedule timely replacements or maintenance, ultimately creating a safer work environment.
Can the Occupational Health and Safety Equipment Management System be customized for different industries?
Yes, the Occupational Health and Safety Equipment Management System is designed to be flexible and can be customized for various industries. While the core functionality of equipment management remains the same, the types of equipment, specific safety regulations, and reporting requirements can be tailored to suit different sectors such as construction, manufacturing, healthcare, or mining. The system's database structure and user interface can be adapted to include industry-specific fields and categories, ensuring that it meets the unique needs of each sector's safety requirements.
How does the Occupational Health and Safety Equipment Management System support compliance with safety regulations?
The Occupational Health and Safety Equipment Management System supports compliance with safety regulations in several ways. It maintains detailed records of all safety equipment, including purchase dates, current status, and maintenance history. This information is crucial for demonstrating compliance during audits or inspections. The system can be configured to send alerts for equipment that needs inspection, replacement, or recertification, helping companies stay proactive in their compliance efforts. Additionally, the reporting features can generate documentation required by regulatory bodies, streamlining the compliance process and reducing the risk of penalties due to non-compliance.
How can I add a new field to the Equipment model in the Occupational Health and Safety Equipment Management System?
To add a new field to the Equipment model, you would need to modify the equipment.py
file in the models directory. For example, if you want to add a "manufacturer" field, you would update the Equipment class as follows:
```python from models.models import db
class Equipment(db.Model): id = db.Column(db.Integer, primary_key=True) name = db.Column(db.String(150), nullable=False) description = db.Column(db.Text) status = db.Column(db.String(20), nullable=False, default='Available') purchase_date = db.Column(db.Date, nullable=False) manufacturer = db.Column(db.String(100)) # New field
def to_dict(self):
return {
'id': self.id,
'name': self.name,
'description': self.description,
'status': self.status,
'purchase_date': self.purchase_date.isoformat() if self.purchase_date else None,
'manufacturer': self.manufacturer # Include new field in dictionary
}
```
After adding the new field, you would need to create a new migration file and apply it to update the database schema.
How can I implement a search function for equipment in the Occupational Health and Safety Equipment Management System?
The Occupational Health and Safety Equipment Management System already includes a basic search function for equipment. To enhance it or implement a more advanced search, you can modify the equipment_route
function in the routes.py
file. Here's an example of how you could implement a more complex search that includes multiple fields:
```python @routes.route("/equipment", methods=['GET']) def equipment_route(): search_query = request.args.get('search', '') status_filter = request.args.get('status', '')
query = Equipment.query
if search_query:
query = query.filter(
(Equipment.name.ilike(f'%{search_query}%')) |
(Equipment.description.ilike(f'%{search_query}%')) |
(Equipment.manufacturer.ilike(f'%{search_query}%'))
)
if status_filter:
query = query.filter(Equipment.status == status_filter)
equipment_list = query.all()
return render_template("equipment.html", with_sidebar=True, equipment_list=equipment_list, search_query=search_query, status_filter=status_filter)
```
This implementation allows searching across multiple fields (name, description, and manufacturer) and includes an optional status filter. You would also need to update the equipment.html
template to include the new search and filter options in the form.
Created: | Last Updated:
Here's a step-by-step guide for using the Occupational Health and Safety Equipment Management System template:
Introduction
This template provides a web-based Occupational Health and Safety Equipment Management System. It allows you to manage safety equipment inventory, employee information, and equipment loans with user authentication.
Getting Started
-
Click "Start with this Template" to begin using the Occupational Health and Safety Equipment Management System template in Lazy.
-
Press the "Test" button to deploy the application and launch the Lazy CLI.
-
Once the deployment is complete, you'll receive a dedicated server link to access the web application.
Using the Application
Logging In
- Open the provided server link in your web browser.
- You'll be presented with a login page. Use your Google account to log in.
Navigating the Dashboard
After logging in, you'll see the main dashboard with the following features:
- Home: Provides an overview of the system
- Equipment: Allows you to manage your equipment inventory
Managing Equipment
To manage equipment:
- Click on the "Equipment" link in the sidebar.
- You'll see a list of all equipment items.
- To add new equipment:
- Click the "Add Equipment" button
- Fill in the required information (name, description, purchase date)
- Click "Save Equipment"
- To edit existing equipment:
- Click the "Edit" button next to the equipment item
- Update the information as needed
- Click "Save Changes"
- To delete equipment:
- Click the "Delete" button next to the equipment item
- Confirm the deletion when prompted
Searching Equipment
To search for specific equipment:
- On the Equipment page, use the search bar at the top.
- Enter your search query and press Enter or click the "Search" button.
- The list will update to show only matching equipment.
Logging Out
To log out of the application:
- Click the "Logout" button in the sidebar or at the top-right corner of the page.
- Confirm that you want to log out when prompted.
Conclusion
You now have a fully functional Occupational Health and Safety Equipment Management System. This web-based application allows you to efficiently manage your safety equipment inventory, track equipment status, and maintain purchase dates. The user-friendly interface makes it easy for your team to keep your safety equipment organized and up-to-date.
Here are the top 5 business benefits of this Occupational Health and Safety Equipment Management System template:
Template Benefits
-
Improved Safety Compliance: Centralized management of safety equipment ensures that all necessary gear is accounted for, properly maintained, and readily available, helping organizations meet occupational health and safety regulations more effectively.
-
Enhanced Inventory Control: The system allows for real-time tracking of equipment status, purchase dates, and availability, reducing the risk of shortages or over-purchasing and optimizing resource allocation.
-
Increased Operational Efficiency: Streamlined processes for equipment management, including easy-to-use interfaces for adding, editing, and deleting equipment records, save time and reduce administrative overhead.
-
Better Decision Making: With comprehensive data on equipment usage, status, and history, managers can make more informed decisions about equipment replacement, maintenance schedules, and budget allocation.
-
Improved Accountability: User authentication and detailed equipment tracking create a clear audit trail, enhancing accountability among employees for the use and care of safety equipment, potentially reducing loss and misuse.
Technologies
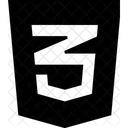
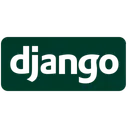
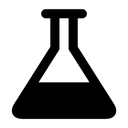
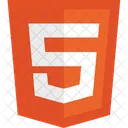

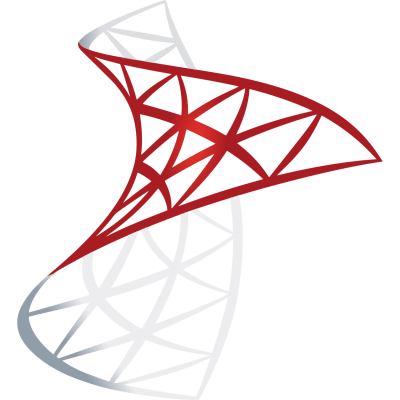