by Lazy Sloth
Gmail Email Sender App
import smtplib
import os
from email.mime.multipart import MIMEMultipart
from email.mime.text import MIMEText
def send_email(subject, message, sender_email, sender_password, recipient_email):
msg = MIMEMultipart()
msg['From'] = sender_email
msg['To'] = recipient_email
msg['Subject'] = subject
msg.attach(MIMEText(message, 'plain'))
server = smtplib.SMTP('smtp.gmail.com', 587)
server.starttls()
server.login(sender_email, sender_password)
text = msg.as_string()
server.sendmail(sender_email, recipient_email, text)
server.quit()
def main():
subject = "test"
message = "this is a first test"
sender_email = os.environ['SENDER_EMAIL']
Frequently Asked Questions
What are some business applications for this Gmail Email Sender App?
The Gmail Email Sender App can be utilized in various business scenarios, such as: - Automated customer notifications for order confirmations or shipping updates - Sending scheduled reports to team members or stakeholders - Implementing a basic email marketing system for newsletters or promotional content - Automating follow-up emails to leads or clients - Sending internal alerts or notifications for critical business events
How can this app improve business communication efficiency?
The Gmail Email Sender App can significantly enhance business communication efficiency by: - Reducing manual email sending tasks, saving time for employees - Ensuring consistent and timely communication with customers and partners - Enabling automated responses to common inquiries or events - Facilitating bulk email sending for announcements or updates - Integrating email functionality into existing business processes or applications
Is this Gmail Email Sender App suitable for small businesses with limited technical resources?
Yes, the Gmail Email Sender App is well-suited for small businesses with limited technical resources because: - It uses Python, a beginner-friendly programming language - The code is concise and easy to understand - It leverages Gmail's SMTP server, which is widely accessible and reliable - The app can be easily customized and expanded to meet specific business needs - It provides a foundation for building more complex email automation systems as the business grows
How can I modify the Gmail Email Sender App to send HTML emails instead of plain text?
To send HTML emails, you can modify the send_email
function in the Gmail Email Sender App. Replace the plain text attachment with an HTML attachment:
```python from email.mime.text import MIMEText
def send_email(subject, message, sender_email, sender_password, recipient_email): msg = MIMEMultipart() msg['From'] = sender_email msg['To'] = recipient_email msg['Subject'] = subject
# Replace plain text with HTML
html_message = f"<html><body><p>{message}</p></body></html>"
msg.attach(MIMEText(html_message, 'html'))
# Rest of the function remains the same
...
```
This modification allows you to send emails with HTML formatting, enabling rich text, images, and other HTML elements in your emails.
Can the Gmail Email Sender App be adapted to use a different email service provider?
Yes, the Gmail Email Sender App can be adapted to use different email service providers. You'll need to modify the SMTP server settings and potentially the authentication method. Here's an example of how you might modify the app to use Outlook's SMTP server:
```python def send_email(subject, message, sender_email, sender_password, recipient_email): # ... (previous code remains the same)
# Replace Gmail's SMTP server with Outlook's
server = smtplib.SMTP('smtp-mail.outlook.com', 587)
server.starttls()
server.login(sender_email, sender_password)
# ... (rest of the function remains the same)
```
Remember to update the sender email and password to match your Outlook account. Similar modifications can be made for other email service providers by changing the SMTP server address and port.
Created: Wed, Jul 31, 2024, 3:43 PM | Last Updated: Invalid Date
Here's a step-by-step guide for using the Gmail Email Sender App template:
Introduction
The Gmail Email Sender App is a simple yet powerful tool that allows you to send emails through Gmail using SMTP. This template provides a foundation for building more complex email-sending applications and can be easily customized to suit your specific needs.
Getting Started
- Click "Start with this Template" to begin using the Gmail Email Sender App template in the Lazy Builder interface.
Initial Setup
Before using the app, you need to set up the following environment secrets in the Environment Secrets tab within the Lazy Builder:
SENDER_EMAIL
: Your Gmail email addressSENDER_EMAIL_PASSWORD
: Your Gmail app password (not your regular Gmail password)RECIPIENT_EMAIL
: The email address of the recipient
To obtain the Gmail app password:
- Go to your Google Account settings
- Navigate to the Security section
- Enable 2-Step Verification if not already enabled
- Under "Signing in to Google," select "App passwords"
- Generate a new app password for "Mail" and "Other (Custom name)"
- Use this generated password as your
SENDER_EMAIL_PASSWORD
Test
Once you've set up the environment secrets, click the "Test" button to deploy the app and launch the Lazy CLI.
Using the App
The app will automatically send a test email using the credentials you provided in the environment secrets. The email will have the subject "test" and the message "this is a first test".
Template Benefits
-
Automated Customer Communication: Businesses can use this template to set up automated email notifications for order confirmations, shipping updates, or appointment reminders, enhancing customer service and reducing manual workload.
-
Marketing Campaign Automation: The template can be adapted to send bulk marketing emails, newsletters, or promotional content to a list of subscribers, helping businesses maintain regular contact with their audience and drive engagement.
-
Internal Communication Tool: Companies can utilize this script to create an internal notification system for team updates, meeting reminders, or important announcements, improving organizational communication efficiency.
-
Reporting and Analytics Distribution: Businesses can automate the distribution of regular reports, analytics, or performance metrics to stakeholders, ensuring timely and consistent information sharing across the organization.
-
Alert System for Critical Events: The template can be modified to send immediate alerts for critical business events, such as system outages, security breaches, or urgent customer issues, enabling rapid response and minimizing potential damages.
Technologies
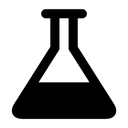
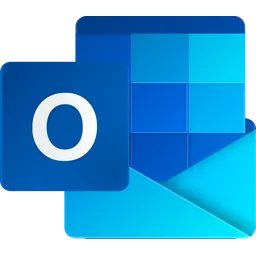
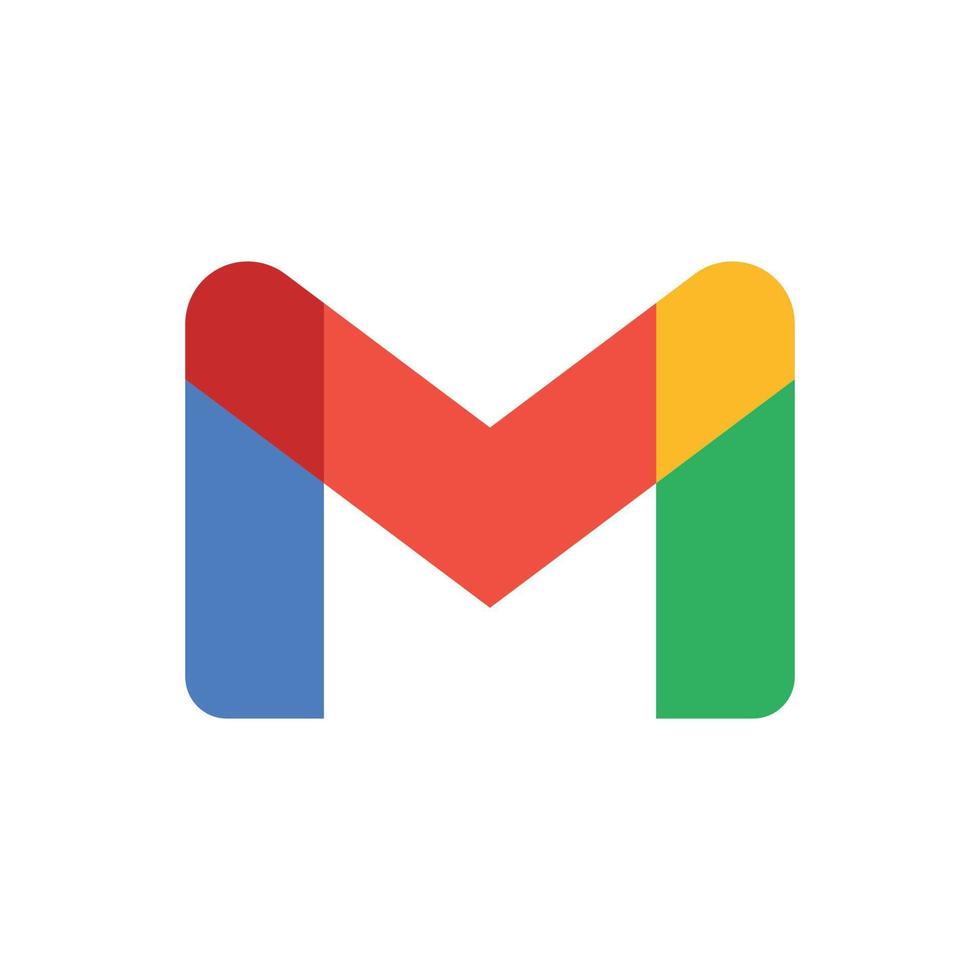