Creating an e-commerce website in Django proves to be both fun and a bit challenging. From creating multiple online stores, I have learned some valuable lessons about utilizing Django templates for e-commerce projects.
Important Features of Successful eCommerce Templates
Template Structure
According to the market, every e-commerce platform quite hugely depends on the template structure. A template hierarchy that is well organized, ensures your online store is scalable, maintainable, and provides a consistent user experience. This includes product cards, navigation menus, shopping cart displays, and checkout forms that will help users through their purchasing journey.
Creating a Homepage That Welcomes Visitors
The homepage is usually the bridge to your e-commerce sites. It must connect your featured products, showcase your ongoing promotions, and give an easy navigation link to the various product categories. Designing with a strong visual hierarchy in mind but also keeping load times down and making it mobile responsive for, again, maximum engagement.
Conversion-focused Optimization of Product Listing Pages
Product listing pages are one of the most important touchpoints in your customer's journey. They must implement useful filtering options, transparent pricing information, and clearly visible call-to-action buttons. Don't let a template clutter your comprehensive product information — fine-tune it so it helps the user make purchase decisions without overwhelming them with unnecessary details.
Essential Template Structure
A clean and neat template structure is the base for every e-commerce site. The basic directory layout that I use in every single project looks like the following:
templates/ |-- base.html |-- shop/ |-- product_list.html |-- product_detail.html |-- cart.html |-- checkout.html |-- accounts/ |-- profile.html |-- orders.html
The Base Template
{% block extra_head %}{% endblock %} Home Products Cart ({{ cart.total_items }}) {% block content %} {% endblock %} © {% now "Y" %} My Shop
Personal Experience Note: During my first e-commerce project, I made the mistake of putting too much logic in the templates. Here's a better approach I now use for the product listing:
{% extends 'base.html' %} {% block content %} {% for product in products %} {{ product.name }} Price: ${{ product.price }} {% if product.in_stock %} {% csrf_token %} Add to Cart {% else %} Out of Stock {% endif %} {% endfor %} {% endblock %}
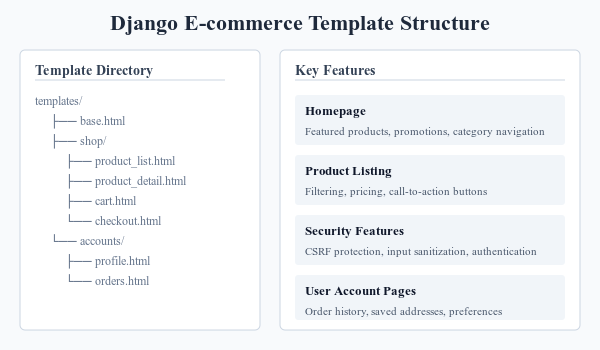
Production Tip: Always handle template inheritance properly and keep your code DRY (Don't Repeat Yourself). Use template blocks effectively to create modular components that can be reused across different pages of your e-commerce site.
Personalizing User Accounts and Order History Pages
Well-structured account pages form a good foundation for personal user experiences. The secret is to provide your users with news in a simple, structured way that allows them to access their order history, saved addresses, and preferences quickly. For order history pages, show order information from most recent to oldest, show order statuses, and make surethe order detail view is easily accessible. You might also think about adding filtering options where users can filter orders by date range or status so that they can easily find specific purchases.
Security Considerations for eCommerce Templates
Security should never be an afterthought in e-commerce development. When designing your templates, always implement CSRF protection for all forms, sanitize any user input before display, and never expose sensitive information in template variables. Ensure that order details and personal information are only accessible to authenticated users with the proper permissions. Additionally, implement proper escaping for all dynamic content to prevent XSS attacks.
Frequently Asked Questions
How do I handle cart templates?
{% extends 'base.html' %} {% block content %} {% if cart_items %} {% for item in cart_items %} {{ item.product.name }} Quantity: {{ item.quantity }} Price: ${{ item.total_price }} {% csrf_token %} Remove {% endfor %} {% else %} Your cart is empty {% endif %} {% endblock %}
The best way for category navigation in templates?
Log structure template is included for maintainability. Store your category navigation in its own template file and use some template tag to highlight the active category. If you have a complex category structure, you might want to think about a breadcrumb approach.
How should templates handle product variations?
Just use template conditionals showing different variation options (size, color, etc) for that product type. Do not make it possible to select variations when one or more are out of stock. Perhaps JavaScript could dynamically refresh prices and availability?
Final Thoughts
In my experience, good Django e-commerce templates come with a balance of features and simplicity. Always keep templates render-focused, move all rich logic to views, and don't forget to be able to scale.
Don't forget to implement proper template inheritance, handle edge cases gracefully, and use a widespread structure for your e-commerce site. Following these practices will save you loads of debugging and maintenance in the long term.