Dashboard interfaces have been my favorite experience with Django. A great dashboard can make complex data into actionable insights, and I'm looking forward to sharing my hands-on learning in constructing these interfaces.
Essential Dashboard Components
Building a successful dashboard interface involves a well-planned, cohesive implementation of key components. Having built a number of admin panels & analytics dashboards in my career, I can tell you that data visualization, real-time updates, and a clear navigation structure, are the actual building blocks for creating a dashboard that works. The secret to this success is sequencing these elements to create a narrative with your data. The users should be able to catch important information at a glance, but at the same time be able to see more details if desired. The Organization of your layout should lead (or guide) your users through the data in a logical manner, where the most relevant information is most prominent while supporting data is available but less prominent than more critical info.
Setting Up the Dashboard Structure
# dashboard/views.py
from django.views.generic import TemplateView
from django.contrib.auth.mixins import LoginRequiredMixin
class DashboardView(LoginRequiredMixin, TemplateView):
template_name = 'dashboard/main.html'
def get_context_data(self, **kwargs):
context = super().get_context_data(**kwargs)
context['analytics'] = self.get_analytics_data()
context['recent_activities'] = self.get_recent_activities()
context['performance_metrics'] = self.get_performance_data()
return context
def get_analytics_data(self):
return {
'total_users': User.objects.count(),
'active_users': User.objects.filter(is_active=True).count(),
'monthly_revenue': Order.objects.this_month().aggregate(
total=Sum('amount')
)['total'] or 0,
}
Implementing Data Visualizations
{% extends 'base.html' %}
{% block content %}
{% block extra_scripts %}
{% endblock %}
Creating Interactive Widgets
{{ widget.title }}
{{ widget.current_value }}
{{ widget.trend }}%
Data Processing and Analytics Integration
Creating effective dashboards is much more than just showing data it is adding meaning and actionable insight. One important lesson I learned during my dashboard development is that data should be preprocessed before going to a template, as this allows for maintainability and good performance. Developing less processor-intensive tasks in the frontend and backend, responsive UI, or loading indicators can be tricky because they are visible to users to provide a better experience. The key is to strike the right balance between live updates and system resource stability.
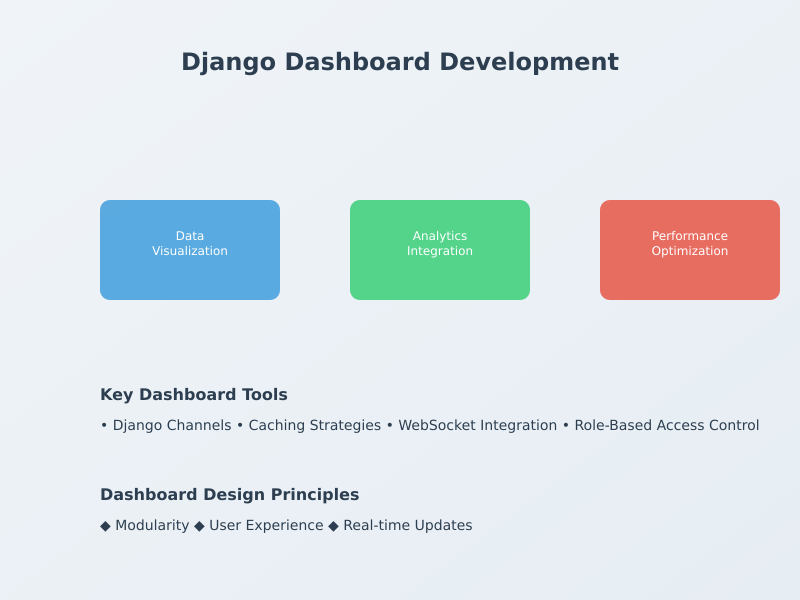
User Experience Optimization
They need to understand how the users are going to interact with the design. After years of collecting user feedback and improving along the way, I found that great dashboards need to find a balance between function and simplicity. That involves meticulously reviewing the placement of each element, verifying a unified movement flow, and offering an obvious visual hierarchy that leads people through intricate data sets. Make responsive and interactive elements seamless, and handle the loading and error states gracefully to keep the users level of trust in the system.
Personal Experience Note: In my experience with different dashboard layouts, I've noticed that elegant best-in-class dashboard creation flourishes with flexible widgets but still must be contained within a well-designed structure.
Performance Optimization Strategies
From experience with working on dashboards with millions of data points, performance optimization is critical for user experience. The difficulty is balancing real-time updates of data vs server load vs browser efficiency. By implementing caching strategies, utilizing asynchronous loading, and ensuring proper database query optimization, we can achieve dashboards for which value retrieval appears instantaneous while still being accurate in terms of value representation.
Mobile Responsiveness in Dashboards
There is a unique challenge in the area in data visualization and user interaction when we are building mobile-responsive dashboards. The most important fact is that the goal is not to fit everything into smaller screens, but to find ways to show and interact with the content in a better way on mobile devices. This very often involves rethinking elaborate visualizations for touch-driven interfaces, considering new formats and new ways to display data that, on desktop views, might be exposed as sprawling tables or charts.
Custom Analytics Integration
One of the building blocks of every dashboard is a powerful analytics engine. Having dealt with many analytics needs, I've created a modular, maintainable and extensible approach.
# analytics/dashboard_metrics.py
class DashboardAnalytics:
def __init__(self, user, date_range=30):
self.user = user
self.date_range = date_range
self.today = timezone.now().date()
self.start_date = self.today - timedelta(days=self.date_range)
def get_sales_metrics(self):
return Order.objects.filter(
created_at__gte=self.start_date
).aggregate(
total_sales=Sum('total_amount'),
average_order=Avg('total_amount'),
order_count=Count('id')
)
def get_trending_products(self):
return OrderItem.objects.filter(
order__created_at__gte=self.start_date
).values('product__name').annotate(
sold_count=Count('id'),
revenue=Sum('price_at_time')
).order_by('-sold_count')[:5]
Personal Experience Note: I used to write analytics queries in views, but this led to messy, hard-to-maintain code. We now have a dedicated analytics class that makes it really easy to test and change metrics when the requirements change.
All our metric calculations are encapsulated in the Dashboard Analytics class. It takes a user object and an optional date range, so it can be useful for various time-based analyses. The get_sales_metrics method returns summary data about sales, and get_trending_products finds popular items.
# views.py
class AnalyticsDashboardView(LoginRequiredMixin, TemplateView):
template_name = 'dashboard/analytics.html'
def get_context_data(self, **kwargs):
context = super().get_context_data(**kwargs)
analytics = DashboardAnalytics(self.request.user)
context.update({
'sales_metrics': analytics.get_sales_metrics(),
'trending_products': analytics.get_trending_products(),
'chart_data': self.prepare_chart_data(analytics)
})
return context
So, you keep your view layer clean by only preparing data for the template (which is its responsibility). I've found this separation very beneficial if you need to access the same metrics on different dashboard views.
Production Tip: I cache these analytics queries for better performance, especially for non-real-time metrics. This greatly alleviates database load at peak usage times.
# Template usage
Total Sales
${{ sales_metrics.total_sales|floatformat:2 }}
Past {{ date_range }} days
Trending Products
{% for product in trending_products %}
{{ product.product__name }}
{{ product.sold_count }} units
${{ product.revenue|floatformat:2 }}
{% endfor %}
There is a template code for displaying these metrics in a more clear, organized fashion. From my experience of styling the dashboard interface, using semantic HTML and clear class names makes it so much easier to maintain and style.
The one thing that has stuck with me until now is the importance of error handling in analytics displays. Always plan for edge cases like - what if the sales are 0 for the selected period? What if a product has indeed been deleted but existed in historical data? Such logic — and how it is reflected in templates — should take these considerations into account.
There are various ways that this analytics can be integrated further- Weekly / Monthly Comparisons - User-based filtering - Exporting functionality - Integration with Analytics Providers.
Frequently Asked Questions
How do I handle real-time updates in Django dashboards?
For live updates, I integrate WebSocket connections through Django Channels. This enables an efficient push notification methodology without any expectancy of polling.
What's the best way to structure dashboard templates?
I would suggest taking a modular approach with reusable components. The resident widgets need to be broken into small maintainable and updatable widgets, to be persistent.
How can I optimize dashboard loading times?
Then focus on lazy loading and fetching non-related things on demand, caching them effectively, and paginating (or infinite scroll) through large datasets.
What's the best approach for handling different user roles in dashboards?
Create role-based dashboard views with custom middleware to manage access control and content visibility based on user permissions.
How do I implement custom filters in dashboard views?
Use a combination of class-based views and custom template tags to create flexible filtering systems that can be easily extended.
Final Thoughts
The art of building good dashboards in Django is an iterative process of balancing functionality, performance, and user experience. Along the way, focus on making your code more modular and maintainable while always keeping the end user's needs first in all of your design choices.