AI Agent
import logging
from gunicorn.app.base import BaseApplication
from app_init import create_initialized_flask_app
# Flask app creation should be done by create_initialized_flask_app to avoid circular dependency problems.
app = create_initialized_flask_app()
# Setup logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
class StandaloneApplication(BaseApplication):
def __init__(self, app, options=None):
self.application = app
self.options = options or {}
super().__init__()
def load_config(self):
# Apply configuration to Gunicorn
for key, value in self.options.items():
if key in self.cfg.settings and value is not None:
self.cfg.set(key.lower(), value)
def load(self):
Frequently Asked Questions
How can businesses benefit from using the AI Agent template?
The AI Agent template offers several benefits for businesses:
- Customization: Companies can tailor the AI's responses to align with their brand voice and specific use cases.
- Team Collaboration: The template includes team management features, allowing multiple team members to contribute to the agent's development.
- Model Flexibility: Businesses can experiment with different AI models (GPT-4, Claude, Gemini) to find the best fit for their needs.
- Real-time Testing: The built-in simulator enables immediate testing of the AI Agent's behavior, ensuring it meets business requirements before deployment.
- Scalability: As a starting point for AI integration, the template can be expanded to handle various business applications, such as customer support, lead generation, or internal knowledge management.
What are some practical applications of the AI Agent in different industries?
The AI Agent template can be adapted for various industries:
- Customer Service: Create a customized chatbot to handle frequently asked questions and provide 24/7 support.
- Healthcare: Develop an AI assistant to help patients with appointment scheduling or basic medical information.
- Education: Build a tutor bot that can answer students' questions on specific subjects.
- Real Estate: Design an AI agent to provide property information and schedule viewings.
- Finance: Create a bot to assist with basic financial advice or account inquiries.
The flexibility of the AI Agent allows businesses to tailor its functionality to their specific industry needs.
How does the team management feature in the AI Agent template enhance collaboration and security?
The team management feature in the AI Agent template provides several collaborative and security benefits:
- Access Control: Administrators can manage who has access to the AI Agent configuration by adding specific email addresses or allowing entire email domains.
- Role Assignment: Team members can be assigned different roles, controlling their level of access and permissions within the system.
- Domain-based Access: Companies can allow all employees from specific email domains to access the agent, streamlining onboarding for larger organizations.
- Blocking Capability: The system includes a block list feature to quickly revoke access if needed, enhancing security.
These features ensure that the right people have the appropriate level of access to the AI Agent, fostering collaboration while maintaining security.
How can I modify the AI Agent's response temperature, and what effect does this have on its output?
The AI Agent's response temperature can be adjusted in the home.html
template. Here's the relevant code snippet:
```html
```
The temperature setting affects the randomness of the AI's responses. A lower temperature (closer to 0) results in more deterministic and focused responses, while a higher temperature (up to 2) increases creativity and variability. This allows users to fine-tune the AI Agent's behavior based on their specific needs, balancing between consistency and creativity in the responses.
How can I add a new AI model option to the AI Agent template?
To add a new AI model option, you'll need to modify both the frontend and backend of the AI Agent template. Here's how:
Created: | Last Updated:
Here's a step-by-step guide for using the AI Agent template:
Introduction to the AI Agent Template
The AI Agent template allows you to create and manage a customizable AI-powered chatbot. This versatile web application lets you configure how the agent responds by providing specific instructions, selecting from different AI models, and adjusting the creativity level of responses. The template also includes team management features, enabling you to control access to the agent by managing email domains and individual users.
Getting Started
To begin using the AI Agent template, follow these steps:
-
Click "Start with this Template" to initialize the project in the Lazy Builder interface.
-
Press the "Test" button to deploy the application and launch the Lazy CLI.
Using the AI Agent Application
Once the application is deployed, you'll be able to access and configure your AI Agent through a web interface. Here's how to use the main features:
Configuring the Chatbot
-
Navigate to the "Home" page of the application.
-
In the "Chatbot Settings" section, you can customize the following:
- Chatbot Instructions: Enter custom instructions for your AI agent in the text area.
- AI Model Selection: Choose from available models such as GPT-4, Claude, or Gemini.
-
AI Response Temperature: Adjust the slider to control the creativity of the AI's responses.
-
Click the "Save Changes" button to apply your settings.
Testing the Chatbot
-
Click on the "Open Simulator" button or navigate to the "AI Chat" page.
-
In the chat interface, type your message and press Enter or click the send button.
-
The AI agent will respond based on your configured settings.
-
You can continue the conversation to test different scenarios and responses.
Managing Team Access
-
Go to the "Team" page to manage access to the AI Agent.
-
To add individual admin access:
- Click "Add New Admin"
- Enter the email address of the user you want to grant access
-
Click "Add Admin"
-
To allow access by email domain:
- Click "Add New Email Ending"
- Enter the domain (e.g., "example.com") without the "@" symbol
-
Click "Allow Domain"
-
You can also block or unblock admins, and delete email domains as needed.
Integrating the AI Agent
The AI Agent is designed to be used as a standalone web application. There's no need for additional integration steps. Users with the appropriate access can simply log in to the web interface to configure and interact with the chatbot.
By following these steps, you'll have a fully functional AI Agent that you can customize to meet your specific needs. This template provides a solid foundation for building AI-powered chatbots that can be easily managed by your team.
Here are 5 key business benefits of this AI Agent template:
Template Benefits
-
Customizable AI Assistant: Easily configure an AI chatbot tailored to your company's specific needs, knowledge base, and communication style without extensive coding.
-
Team Collaboration: Enable multiple team members to collaboratively refine and improve the AI agent through shared access and configuration capabilities.
-
Model Flexibility: Experiment with different AI models (GPT-4, Claude, Gemini) to find the best fit for your use case and budget, all from a single interface.
-
Real-time Testing: Use the built-in simulator to immediately test changes and ensure the AI agent behaves as expected before deployment.
-
Secure Access Management: Control who can access and modify the AI agent through domain-level and individual email permissions, maintaining security and accountability.
Technologies
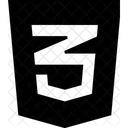
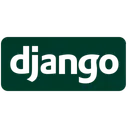
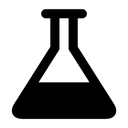
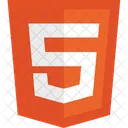

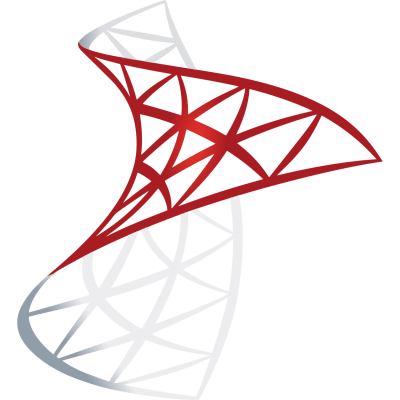