Hussain's Shop: Comprehensive Web App with Login, News, and Chatbot Integration
import logging
from gunicorn.app.base import BaseApplication
from app_init import app
# IMPORT ALL ROUTES
from routes import *
# Setup logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
class StandaloneApplication(BaseApplication):
def __init__(self, app, options=None):
self.application = app
self.options = options or {}
super().__init__()
def load_config(self):
# Apply configuration to Gunicorn
for key, value in self.options.items():
if key in self.cfg.settings and value is not None:
self.cfg.set(key.lower(), value)
Frequently Asked Questions
How can Hussain's Shop benefit from implementing this web application?
Hussain's Shop can greatly benefit from this web application in several ways: - Enhanced customer engagement through the AI chatbot, providing instant support and information. - Improved user experience with a clean, responsive design that works on both desktop and mobile devices. - Ability to showcase latest news and updates, keeping customers informed about new products or promotions. - Secure user authentication system, allowing for personalized experiences and potential future features like order history or loyalty programs.
What types of businesses, besides retail shops, could adapt this template for their needs?
The Hussain's Shop template is versatile and can be adapted for various business types, including: - Restaurants: Showcasing menus, daily specials, and allowing reservations through the chatbot. - Service providers (e.g., salons, repair shops): Displaying service offerings, pricing, and enabling appointment booking. - Educational institutions: Presenting course information, campus news, and providing student support via the chatbot. - Non-profit organizations: Sharing mission updates, event information, and facilitating donations.
How can the news section in Hussain's Shop's template be leveraged for marketing purposes?
The news section in Hussain's Shop's template offers excellent marketing opportunities: - Announce new product arrivals or seasonal collections. - Highlight special promotions, sales, or limited-time offers. - Share customer success stories or product reviews. - Provide educational content related to products (e.g., usage tips, care instructions). - Communicate important business updates, such as changes in store hours or new locations.
How can I modify the chat functionality in Hussain's Shop's template to handle specific product inquiries?
To handle specific product inquiries in the chat functionality, you can modify the chat_route
function in routes.py
. Here's an example of how you could extend it:
```python from database_operations import get_product_by_name
@app.route("/chat", methods=['POST']) def chat_route(): data = request.get_json() user_message = data.get('message', '')
# Check if the message is a product inquiry
if user_message.lower().startswith("tell me about"):
product_name = user_message[13:].strip()
product = get_product_by_name(product_name)
if product:
response = f"Here's information about {product['name']}: Price: ${product['price']}, Stock: {product['stock']}"
else:
response = f"I'm sorry, I couldn't find information about {product_name}."
else:
# Use the existing LLM for general inquiries
result = llm(
prompt=f"You are a helpful assistant for Hussain's Shop. Respond to: {user_message}",
response_schema=response_schema,
image_url=None,
model="gpt-4o",
temperature=0.7
)
response = result.get("response", "I apologize, but I couldn't process your request.")
return jsonify({"response": response})
```
This modification allows the chatbot to handle specific product inquiries while still using the LLM for general questions.
How can I add a product listing page to Hussain's Shop's template?
To add a product listing page to Hussain's Shop's template, you'll need to create a new route, template, and update the sidebar. Here's a basic example:
In routes.py
, add:
```python from database_operations import get_all_products
@app.route("/products") @with_sidebar def products_route(): products = get_all_products() return render_template("products.html", products=products) ```
Create a new file products.html
in the templates folder:
```html
{% extends "layout.html" %}
{% block content %}
{% endblock %} ```
Finally, update _sidebar.html
to include the new products page:
html
<a class="nav-link {% if request.endpoint == 'products_route' %}active{% endif %}" href="{{ url_for('products_route') }}">
<i class="fas fa-shopping-bag"></i> Products
</a>
These changes will add a new Products page to Hussain's Shop's template, displaying all available products in a grid layout.
Created: | Last Updated:
Here's a step-by-step guide for using the Hussain's Shop template:
Introduction
This template provides a comprehensive web application for Hussain's Shop, featuring user authentication, a news information page, and an integrated AI chatbot for support and content generation. The app includes a landing page, user profiles, and a sidebar navigation system.
Getting Started
- Click "Start with this Template" to begin using the Hussain's Shop template in the Lazy Builder interface.
Test the Application
- Press the "Test" button in the Lazy Builder interface to deploy the application and launch the Lazy CLI.
Using the Application
Once the application is deployed, you can access and use its features:
Landing Page
- The landing page welcomes users to Hussain's Shop and provides a login button.
User Authentication
- Users can log in using the provided authentication system.
- After logging in, users will see their profile picture and name in the sidebar.
Profile Page
- The profile page displays a welcome message and the latest news from Hussain's Shop.
- An AI-powered chatbot is available on this page for customer support.
Chatbot Interaction
To interact with the chatbot: 1. Type your message in the input field at the bottom of the chat window. 2. Click the "Send" button or press Enter to send your message. 3. The AI will respond with helpful information about Hussain's Shop.
Navigation
- Use the sidebar to navigate between different sections of the app.
- The sidebar includes links to the user's profile and a logout option.
Customization
To customize the application for your specific needs:
- Modify the
landing.html
,profile.html
, and other template files to change the content and layout. - Update the CSS files (
landing.css
,profile.css
,sidebar.css
) to adjust the styling. - Edit the
database_operations.py
file to change database operations if needed. - Modify the
routes.py
file to add or change route handlers.
Integrating the Chatbot
The chatbot is already integrated into the application. To customize its behavior:
- Locate the
chat_route
function in theroutes.py
file. - Modify the prompt sent to the LLM to change the chatbot's personality or knowledge base:
python
result = llm(
prompt=f"You are a helpful assistant for Hussain's Shop. Respond to: {user_message}",
response_schema=response_schema,
image_url=None,
model="gpt-4o",
temperature=0.7
)
- Adjust the
temperature
parameter to control the creativity of the responses.
By following these steps, you'll have a fully functional web application for Hussain's Shop with user authentication, news updates, and an AI-powered chatbot for customer support.
Here are 5 key business benefits for this template:
Template Benefits
-
Enhanced Customer Engagement: The integrated AI chatbot provides instant support and information to customers, improving their shopping experience and potentially increasing sales conversions.
-
Secure User Management: The robust authentication system ensures user data protection and personalized experiences, fostering customer loyalty and trust in the e-commerce platform.
-
Scalable E-commerce Foundation: With product and cart database models in place, the template offers a solid foundation for building a full-fledged online store, allowing for easy expansion of product offerings and features.
-
Efficient Content Management: The news section allows for quick dissemination of updates, promotions, and important information to customers, keeping them informed and engaged with the brand.
-
Responsive Design: The mobile-friendly layout ensures a seamless shopping experience across devices, potentially increasing mobile conversions and reaching a wider customer base.
Technologies
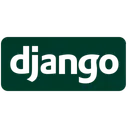
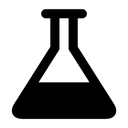

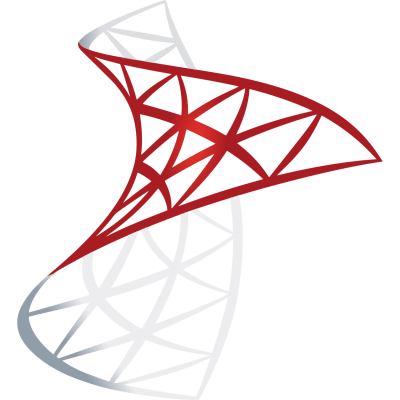