Browser-use AI Agent Prompt Manager
import logging
from gunicorn.app.base import BaseApplication
from app_init import create_app
# Setup logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
class StandaloneApplication(BaseApplication):
def __init__(self, app, options=None):
self.application = app
self.options = options or {}
super().__init__()
def load_config(self):
# Apply configuration to Gunicorn
for key, value in self.options.items():
if key in self.cfg.settings and value is not None:
self.cfg.set(key.lower(), value)
def load(self):
return self.application
if __name__ == "__main__":
Created: | Last Updated:
To use this code template for a Browser-use AI Agent Prompt Manager, here's a step-by-step guide:
-
First, ensure you have the required directory structure:
your_project/ ├── static/ │ ├── css/ │ │ └── styles.css │ └── js/ │ ├── home.js │ ├── settings.js │ └── task_generator.js ├── templates/ │ ├── partials/ │ │ └── _header.html │ ├── 404.html │ ├── 500.html │ ├── home.html │ ├── settings.html │ └── task_generator.html ├── migrations/ │ ├── 000_example.sql │ ├── 001_create_settings_table.sql │ └── 002_add_continuous_run_column.sql ├── abilities.py ├── app_init.py ├── main.py ├── models.py ├── routes.py └── requirements.txt
-
Create the
abilities.py
file with a basic LLM function and migration handler:
```python import os import logging
def llm(prompt, response_schema, image_url=None, model="gpt-4", temperature=0.7): # Add your LLM implementation here pass
def apply_sqlite_migrations(engine, model, migrations_dir): # Add your migration implementation here pass ```
-
Install dependencies:
bash pip install -r requirements.txt
-
Run the application:
bash python main.py
The template provides:
- A modern UI with Bootstrap styling
- Settings management for AI agent configuration
- Task generation interface
- Database migrations for SQLite
- RESTful API endpoints
- Error handling pages
Key features:
- Settings persistence
- Task prompt generation
- Version history
- Dynamic prompt templating
- Browser configuration
- LLM integration
To customize:
- Modify the HTML templates to match your branding
- Update the CSS in styles.css
- Implement the LLM function in abilities.py
- Add your own database migrations as needed
- Extend the Settings model for additional fields
- Add new routes as required
The application will run on http://localhost:8080 by default.
Template Benefits
- Streamlined AI Agent Management
- Centralizes configuration and management of AI agents that operate in web browsers
- Reduces setup time and eliminates manual prompt engineering for each new use case
-
Enables consistent prompt formatting across multiple Twitter automation tasks
-
Enhanced Social Media Automation
- Facilitates intelligent Twitter engagement through customized AI responses
- Maintains brand voice consistency while allowing personalized interactions
-
Scales social media presence without compromising authenticity
-
Flexible Configuration System
- Provides granular control over AI agent behavior, browser settings, and LLM parameters
- Supports multiple agent configurations with version history tracking
-
Allows quick restoration of previous successful settings
-
Business Process Integration
- Seamlessly incorporates business-specific details and customer profiles into AI interactions
- Generates task descriptions tailored to company objectives and target audience
-
Maintains audit trails of AI agent activities and performance
-
Risk Management & Compliance
- Implements structured error handling and monitoring capabilities
- Maintains detailed logs of AI agent actions for compliance purposes
- Provides controls to ensure AI responses align with business guidelines and tone
Technologies
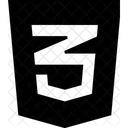
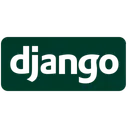
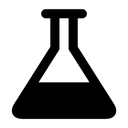
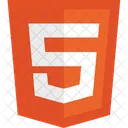


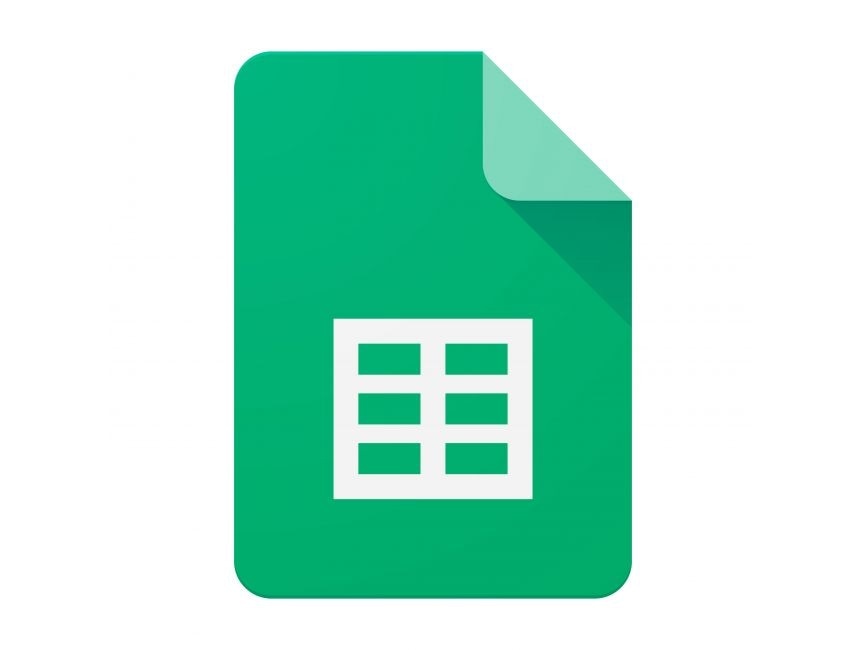