How to Implement Stripe Payment Gateway into React
import os
import logging
from fastapi import FastAPI, HTTPException
from fastapi.middleware.cors import CORSMiddleware
from pydantic import BaseModel
import stripe
import uvicorn
# Constants
STRIPE_SECRET_KEY = os.environ['STRIPE_SECRET_KEY']
YOUR_DOMAIN = os.environ['YOUR_DOMAIN']
# Configure Stripe API key
stripe.api_key = STRIPE_SECRET_KEY
# FastAPI app initialization
app = FastAPI()
# CORS configuration
origins = ["*"]
app.add_middleware(
CORSMiddleware,
allow_origins=origins,
allow_credentials=True,
Frequently Asked Questions
How can this Stripe payment gateway template benefit my e-commerce business?
This Stripe payment gateway template offers significant advantages for e-commerce businesses. It provides a quick and efficient way to set up a secure payment system, allowing you to start accepting payments rapidly. The template supports both one-time payments and subscriptions, giving you flexibility in your pricing models. By integrating this template, you can offer a smooth checkout experience to your customers, potentially increasing conversion rates and customer satisfaction.
Can I use this template for a SaaS (Software as a Service) business model?
Absolutely! This Stripe payment gateway template is particularly well-suited for SaaS businesses. It supports subscription-based payments, which is a common requirement for SaaS models. You can easily set up recurring billing for your services, manage different subscription tiers, and handle upgrades or downgrades. The template's ability to pass UTM parameters also allows you to track the effectiveness of your marketing campaigns in driving subscriptions.
How does this template handle different currencies and international payments?
The Stripe payment gateway template is designed to work with Stripe's global payment infrastructure. Stripe supports payments in over 135 currencies, and this template can accommodate that functionality. You would need to set up the appropriate currency and pricing in your Stripe dashboard, and the template will handle the rest. This makes it an excellent solution for businesses looking to expand internationally or serve a global customer base.
How can I customize the checkout page style to match my brand?
The template provides a foundation for integrating Stripe payments, but the actual styling of the checkout page can be customized in your React component. Here's an example of how you might add some basic styling:
```jsx import React from 'react'; import styled from 'styled-components';
const CheckoutButton = styled.buttonbackground-color: #4CAF50;
border: none;
color: white;
padding: 15px 32px;
text-align: center;
text-decoration: none;
display: inline-block;
font-size: 16px;
margin: 4px 2px;
cursor: pointer;
;
const Checkout = () => { // ... existing code ...
return (
<div>
<h2>Proceed to Checkout</h2>
<CheckoutButton onClick={fetchSession}>
Checkout subscription
</CheckoutButton>
</div>
);
};
export default Checkout; ```
This example uses styled-components to create a custom styled button, but you can use any CSS-in-JS solution or traditional CSS to style your components to match your brand.
Can this template handle multiple products or pricing tiers?
Yes, the Stripe payment gateway template can handle multiple products or pricing tiers. You would need to set up different price IDs in your Stripe dashboard for each product or tier. Then, you can modify the React component to pass the appropriate price_id
based on the user's selection. Here's an example of how you might modify the component to handle multiple pricing tiers:
```jsx import React, { useState } from 'react';
const Checkout = () => { const [selectedTier, setSelectedTier] = useState('basic');
const priceTiers = {
basic: 'price_basic123',
pro: 'price_pro456',
enterprise: 'price_enterprise789'
};
const fetchSession = async () => {
const response = await fetch('YOUR_SERVER_URL/create-checkout-session', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({ months: 1, price_id: priceTiers[selectedTier] })
});
// ... rest of the checkout logic ...
};
return (
<div>
<h2>Select Your Plan</h2>
<select onChange={(e) => setSelectedTier(e.target.value)}>
<option value="basic">Basic</option>
<option value="pro">Pro</option>
<option value="enterprise">Enterprise</option>
</select>
<button onClick={fetchSession}>
Checkout {selectedTier} subscription
</button>
</div>
);
};
export default Checkout; ```
This modification allows users to select different pricing tiers before proceeding to checkout, demonstrating the flexibility of the Stripe payment gateway template.
Created: | Last Updated:
Introduction to the Stripe Payment Gateway Integration Template
This template is designed to help you integrate a Stripe payment gateway into your React application. It provides a backend service built with FastAPI that handles the creation of Stripe checkout sessions and the retrieval of session information. The backend service is complemented by a frontend component that allows users to initiate the checkout process. This step-by-step guide will walk you through the process of using this template on the Lazy platform.
Clicking Start with this Template
To begin, click on "Start with this Template" on the Lazy platform. This will pre-populate the code in the Lazy Builder interface, so you won't need to copy, paste, or delete any code manually.
Initial Setup: Adding Environment Secrets
Before testing the app, you need to set up the necessary environment secrets. These are not set in your operating system but within the Lazy Builder under the Environment Secrets tab.
1. Obtain your Stripe secret key and publishable key from your Stripe dashboard. 2. Set the `STRIPE_SECRET_KEY` environment secret in the Lazy Builder with your Stripe secret key. 3. Set the `YOUR_DOMAIN` environment secret with the domain where your React application is hosted.
Test: Pressing the Test Button
Once you have configured the environment secrets, press the "Test" button on the Lazy platform. This will deploy your app and launch the Lazy CLI. If the code requires any user input, you will be prompted to provide it through the Lazy CLI.
Entering Input
If the code requires user input, you will be prompted to enter it after pressing the test button. Follow the instructions in the Lazy CLI to provide the necessary input.
Using the App
After deployment, Lazy will provide you with a server link. This link is the endpoint URL for your backend service. You can use this link to interact with the API endpoints provided by the FastAPI server.
Integrating the App
To integrate the backend service with your React frontend, follow these steps:
1. Insert the provided frontend code into your React component. 2. Replace `"PUBLISHABLE STRIPE API KEY"` with your actual publishable API key from Stripe. 3. Replace `"LAZY SERVER LINK"` with the endpoint URL provided by Lazy after deployment. 4. Replace `"PRICE_ID"` with the actual price ID you have set up in your Stripe dashboard.
Here is the sample React component code with placeholders:
`import React, { useEffect } from 'react';
import { loadStripe } from '@stripe/stripe-js';
const stripePromise = loadStripe('PUBLISHABLE STRIPE API KEY');
const Checkout = () => {
const fetchSession = async () => {
const response = await fetch('LAZY SERVER LINK/create-checkout-session', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify({ months: 1, price_id: 'PRICE_ID' })
});
const { sessionId } = await response.json();
const stripe = await stripePromise;
const { error } = await stripe.redirectToCheckout({ sessionId });
if (error) {
console.error(error);
}
};
return (
Proceed to Checkout
);
};
export default Checkout;`
By following these steps, you will have successfully integrated a Stripe payment gateway into your React application using the Lazy template.
Template Benefits
-
Rapid Payment Integration: This template allows businesses to quickly implement a secure Stripe payment gateway into their React applications, reducing development time and accelerating time-to-market for e-commerce features.
-
Flexible Pricing Models: The template supports various pricing structures, including one-time payments and subscriptions, enabling businesses to easily adapt their payment options to different products or services.
-
Enhanced User Experience: By utilizing Stripe's embedded UI mode, businesses can provide a seamless checkout experience directly within their application, potentially increasing conversion rates.
-
Marketing Attribution: The inclusion of UTM parameter tracking allows businesses to measure the effectiveness of their marketing campaigns by attributing sales to specific channels or initiatives.
-
Scalability and Reliability: Built on FastAPI and integrated with Stripe's robust API, this template provides a scalable and reliable payment solution that can grow with the business, handling increased transaction volumes without compromising performance.
Technologies
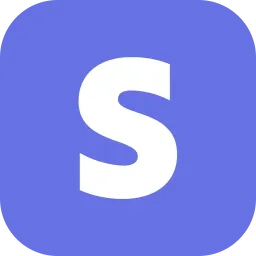
