WhatsApp Message Scheduler
import os
from flask import Flask, render_template, request, flash, redirect, url_for
from flask_sqlalchemy import SQLAlchemy
from datetime import datetime
from gunicorn.app.base import BaseApplication
app = Flask(__name__)
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///messages.db'
app.config['SECRET_KEY'] = 'your_secret_key' # Change this to a random secret key
db = SQLAlchemy(app)
class ScheduledMessage(db.Model):
id = db.Column(db.Integer, primary_key=True)
message = db.Column(db.String(500), nullable=False)
scheduled_time = db.Column(db.DateTime, nullable=False)
created_at = db.Column(db.DateTime, default=datetime.utcnow)
with app.app_context():
db.create_all()
@app.route("/", methods=['GET', 'POST'])
def home():
if request.method == 'POST':
message = request.form.get('message')
Frequently Asked Questions
How can the WhatsApp Message Scheduler benefit businesses?
The WhatsApp Message Scheduler can greatly benefit businesses by allowing them to plan and automate their customer communications. For example, businesses can schedule reminders for appointments, send promotional messages during peak hours, or deliver timely updates to customers without manual intervention. This tool helps improve customer engagement and saves time for business owners and marketing teams.
Can the WhatsApp Message Scheduler be integrated with existing CRM systems?
While the current template doesn't include direct CRM integration, the WhatsApp Message Scheduler can be extended to work with existing CRM systems. Developers can modify the code to pull customer data and message content from a CRM database, allowing for more personalized and targeted messaging. This integration would enable businesses to streamline their communication processes and maintain consistent customer interactions across platforms.
How can I customize the message input form in the WhatsApp Message Scheduler?
You can easily customize the message input form in the WhatsApp Message Scheduler by modifying the HTML and CSS in the index.html
file. For example, to add a subject line to the form, you can insert the following HTML code just before the message input:
html
<label for="subject">Subject:</label>
<input type="text" id="subject" name="subject" required>
Then, update the Python code in main.py
to handle the new field:
python
subject = request.form.get('subject')
new_message = ScheduledMessage(subject=subject, message=message, scheduled_time=scheduled_time)
Don't forget to add the new field to the ScheduledMessage
model in main.py
as well.
What industries could benefit most from using the WhatsApp Message Scheduler?
The WhatsApp Message Scheduler can be particularly beneficial for industries that rely heavily on timely customer communications, such as:
- Healthcare: Sending appointment reminders and follow-up care instructions
- E-commerce: Notifying customers about order status and delivery updates
- Hospitality: Sending booking confirmations and check-in information
- Education: Delivering course updates and assignment reminders to students
- Real estate: Scheduling property viewings and sending market updates to clients
By implementing the WhatsApp Message Scheduler, these industries can improve customer satisfaction and operational efficiency.
How can I implement message templates in the WhatsApp Message Scheduler?
To implement message templates in the WhatsApp Message Scheduler, you can create a dictionary of pre-defined templates and add a dropdown menu to the HTML form. Here's an example of how to do this:
In main.py
, add the following code:
```python message_templates = { "reminder": "Don't forget your appointment on {date} at {time}.", "promotion": "Limited time offer: {discount}% off on all products! Use code {code} at checkout.", "update": "Your order #{order_number} has been {status}. Track it here: {tracking_link}" }
@app.route("/", methods=['GET', 'POST']) def home(): return render_template('index.html', templates=message_templates) ```
In index.html
, add a dropdown menu for templates:
html
<label for="template">Message Template:</label>
<select id="template" name="template">
<option value="">Custom Message</option>
{% for key, value in templates.items() %}
<option value="{{ key }}">{{ value }}</option>
{% endfor %}
</select>
This implementation allows users to choose from pre-defined templates or enter custom messages, making the WhatsApp Message Scheduler more versatile and user-friendly.
Created: | Last Updated:
Here's a step-by-step guide on how to use the WhatsApp Message Scheduler template:
Introduction
The WhatsApp Message Scheduler is a web application that allows users to schedule messages for future delivery. It provides a simple interface for composing messages and setting send times, making it easy to plan your communications in advance.
Getting Started
To begin using this template:
- Click the "Start with this Template" button in the Lazy Builder interface.
Test the Application
Once you've started with the template:
- Click the "Test" button in the Lazy Builder interface.
- This will initiate the deployment process and launch the Lazy CLI.
Using the Application
After the deployment is complete, Lazy will provide you with a dedicated server link to access the web interface. Follow these steps to use the application:
- Open the provided link in your web browser.
- You'll see a simple form with two fields:
- Message: Enter the text of your WhatsApp message here.
- Schedule Time: Use the datetime picker to select when you want the message to be sent.
- Fill in both fields and click the "Schedule Message" button.
- If successful, you'll see a flash message confirming that your message has been scheduled.
Important Notes
- This template provides the scheduling interface but does not actually send WhatsApp messages. To integrate this with a WhatsApp messaging service, you would need to implement additional functionality or connect it to a WhatsApp API.
- The scheduled messages are stored in a SQLite database, which is suitable for demonstration purposes but may not be ideal for production use with high volumes of data.
- The application uses Flask and SQLAlchemy, which are automatically set up by Lazy. You don't need to worry about installing dependencies or configuring the environment.
Next Steps
To turn this into a fully functional WhatsApp scheduling system, you would need to:
- Integrate with a WhatsApp Business API or a third-party service that can send WhatsApp messages.
- Implement a background job system to check for scheduled messages and send them at the appropriate times.
- Add user authentication to allow multiple users to schedule their own messages securely.
Remember, this template serves as a starting point for building a message scheduling system. You can expand upon it to add more features and integrate it with actual messaging services as needed.
Here are 5 key business benefits for the WhatsApp Message Scheduler template:
Template Benefits
-
Improved Customer Engagement: Businesses can schedule personalized messages to customers at optimal times, enhancing engagement and response rates.
-
Efficient Marketing Campaigns: Marketers can plan and schedule promotional messages in advance, ensuring timely delivery of campaigns across different time zones.
-
Automated Customer Service: Companies can set up automated reminders, follow-ups, or support messages to be sent at specific times, improving customer service efficiency.
-
Enhanced Team Communication: For internal use, teams can schedule important announcements or reminders to be sent to colleagues at predetermined times, improving coordination.
-
Time Management and Productivity: By allowing messages to be composed and scheduled in advance, businesses can better manage their communication workflow and save time during busy periods.
Technologies
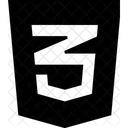
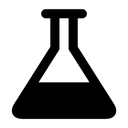
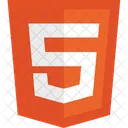