Text-to-Speech Converter
import logging
from gunicorn.app.base import BaseApplication
from app_init import create_initialized_flask_app
# Flask app creation should be done by create_initialized_flask_app to avoid circular dependency problems.
app = create_initialized_flask_app()
# Setup logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
class StandaloneApplication(BaseApplication):
def __init__(self, app, options=None):
self.application = app
self.options = options or {}
super().__init__()
def load_config(self):
# Apply configuration to Gunicorn
for key, value in self.options.items():
if key in self.cfg.settings and value is not None:
self.cfg.set(key.lower(), value)
def load(self):
Frequently Asked Questions
What are some potential business applications for this Text-to-Speech Converter?
The Text-to-Speech Converter has numerous business applications across various industries:
- Accessibility: Companies can use it to make their content more accessible to visually impaired users.
- E-learning: Educational platforms can convert written materials into audio for auditory learners.
- Customer Service: Businesses can use it to create automated voice responses for their phone systems.
- Content Creation: Media companies can quickly convert written articles into audio content for podcasts or audio articles.
- Marketing: Advertisers can create audio versions of their marketing materials for wider reach.
How can this Text-to-Speech Converter be monetized?
There are several ways to monetize the Text-to-Speech Converter:
- Freemium Model: Offer basic functionality for free, with premium features (e.g., more voices, longer text inputs) for a fee.
- API Access: Provide API access to developers who want to integrate text-to-speech capabilities into their own applications.
- Enterprise Solutions: Offer customized solutions for businesses with specific needs, such as bulk conversion or integration with existing systems.
- Pay-per-use: Charge users based on the amount of text converted or the duration of audio generated.
- White-label Solution: License the technology to other businesses to rebrand and sell as their own.
What industries could benefit most from implementing this Text-to-Speech Converter?
Several industries could greatly benefit from the Text-to-Speech Converter:
- Publishing: Convert e-books and articles into audiobooks and podcasts.
- Healthcare: Create audio instructions for patients or convert medical records into speech for easier review.
- Tourism: Develop audio guides for tourist attractions or convert travel information into multiple languages.
- Finance: Generate audio reports for investors or create voice alerts for market changes.
- Legal: Convert legal documents into audio format for easier review or for clients with reading difficulties.
How can I customize the voice output in the Text-to-Speech Converter?
The current implementation uses gTTS (Google Text-to-Speech) which has limited customization options. However, you can modify the convert_text_to_speech
function in routes.py
to use different TTS engines or add voice customization options. For example, to change the language:
```python @app.route("/convert", methods=["POST"]) def convert_text_to_speech(): text = request.json.get("text", "") lang = request.json.get("lang", "en") # Get language from request tts = gTTS(text=text, lang=lang)
# Rest of the function remains the same
```
You would also need to update the frontend to allow language selection.
How can I add user authentication to the Text-to-Speech Converter?
To add user authentication, you can use Flask-Login. First, install it with pip install flask-login
. Then, update app_init.py
:
```python from flask_login import LoginManager
def create_initialized_flask_app(): app = Flask(name, static_folder='static')
# ... existing code ...
login_manager = LoginManager()
login_manager.init_app(app)
@login_manager.user_loader
def load_user(user_id):
return User.query.get(int(user_id))
return app
```
You'll need to create a User model and implement login/logout routes. Then, you can protect routes that require authentication:
```python from flask_login import login_required
@app.route("/convert", methods=["POST"]) @login_required def convert_text_to_speech(): # ... existing code ... ```
This ensures only authenticated users can access the text-to-speech conversion functionality.
Created: | Last Updated:
Here's a step-by-step guide for using the Text-to-Speech Converter template:
Introduction
This template provides a web application that converts text messages to audio using a text-to-speech engine. It allows users to input text and receive an audio file of the spoken text.
Getting Started
- Click "Start with this Template" to begin using the Text-to-Speech Converter template in the Lazy Builder interface.
Test the Application
- Press the "Test" button in the Lazy Builder interface to deploy the application and launch the Lazy CLI.
Using the Text-to-Speech Converter
-
Once the application is deployed, you'll receive a dedicated server link to access the web interface.
-
Open the provided link in your web browser to access the Text-to-Speech Converter application.
-
You'll see a simple interface with the following elements:
- A text area to input your message
- A "Convert to Speech" button
-
An audio player (initially hidden)
-
To use the converter:
- Enter the text you want to convert into the text area
- Click the "Convert to Speech" button
- Wait for the conversion process to complete
-
Once done, an audio player will appear with your converted speech
-
You can play the audio directly in the browser or download it for later use.
Integration (Optional)
This Text-to-Speech Converter can be used as a standalone web application. However, if you want to integrate it into another service or application, you can use its API endpoint.
To integrate the Text-to-Speech Converter API into your own application:
-
Use the server link provided by Lazy as your API base URL.
-
Send a POST request to the
/convert
endpoint with the following structure:
json
{
"text": "Your text to be converted to speech"
}
-
The API will respond with an audio file (MP3 format) containing the converted speech.
-
Here's a sample code snippet for making an API request using JavaScript:
```javascript async function convertTextToSpeech(text) { const response = await fetch('YOUR_SERVER_LINK/convert', { method: 'POST', headers: { 'Content-Type': 'application/json', }, body: JSON.stringify({ text: text }), });
if (response.ok) { const blob = await response.blob(); const audioUrl = URL.createObjectURL(blob); // Use the audioUrl to play or download the audio } else { console.error('Error converting text to speech'); } } ```
Replace YOUR_SERVER_LINK
with the actual server link provided by Lazy after deployment.
By following these steps, you'll have a functional Text-to-Speech Converter application that you can use directly through the web interface or integrate into your own projects using the API.
Here are 5 key business benefits for this Text-to-Speech Converter template:
Template Benefits
-
Accessibility Enhancement: This template can be used to create applications that improve accessibility for visually impaired users or those with reading difficulties, allowing businesses to reach a wider audience and comply with accessibility standards.
-
Content Versatility: By converting text to speech, businesses can repurpose written content into audio formats, creating podcasts, audio blogs, or voice-based user interfaces, thus expanding their content offerings and engagement channels.
-
Productivity Tool: The template can be adapted for internal business use, such as converting emails, reports, or documents to audio, allowing employees to consume information while multitasking or on-the-go, potentially increasing productivity.
-
Language Learning Aid: Language schools or educational technology companies can utilize this template to develop tools that help students improve pronunciation and listening skills by converting text in various languages to speech.
-
Customer Service Enhancement: Businesses can integrate this functionality into their customer service platforms, allowing for automated voice responses or the conversion of text-based FAQs and support documents into audio format, potentially improving customer experience and reducing support costs.
Technologies
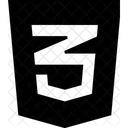
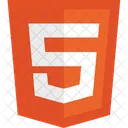
