by wolfyblai
Text Summarizer Web App
import logging
import json
import argparse
from abilities import llm
# Setup logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
def summarize_text(text):
"""Summarize the given text using LLM."""
try:
prompt = f"Please summarize the following text in under 200 words while maintaining the key points and meaning:\n\n{text}"
response_schema = {
"type": "object",
"properties": {
"summary": {
"type": "string",
"description": "The summarized text"
}
},
"required": ["summary"]
}
Frequently Asked Questions
How can businesses benefit from using the Text Summarizer Web App?
The Text Summarizer Web App offers several benefits for businesses: - Time-saving: Quickly condense long documents or articles into concise summaries. - Improved efficiency: Helps employees grasp key information from large volumes of text. - Enhanced decision-making: Provides quick insights from reports, research papers, or customer feedback. - Consistent communication: Ensures uniform summarization across different team members.
What industries could find the Text Summarizer Web App particularly useful?
The Text Summarizer Web App can be valuable in various industries, including: - Media and journalism: Summarizing news articles or press releases. - Legal: Condensing lengthy legal documents or case studies. - Research and academia: Summarizing scientific papers or literature reviews. - Customer service: Quickly understanding and responding to customer inquiries or feedback. - Marketing: Summarizing market research reports or competitor analysis.
How can I customize the summarization process in the Text Summarizer Web App?
The Text Summarizer Web App allows for customization through the summarize_text
function. You can modify the prompt or adjust the LLM parameters. For example, to change the summary length:
python
def summarize_text(text, max_words=200):
prompt = f"Please summarize the following text in under {max_words} words while maintaining the key points and meaning:\n\n{text}"
# ... rest of the function
You can also adjust the temperature
parameter in the llm
function call to control the creativity of the summary.
Can the Text Summarizer Web App handle multiple languages?
The current implementation of the Text Summarizer Web App doesn't specify language handling. However, you can enhance it to support multiple languages by modifying the prompt and potentially using a multilingual LLM model. Here's an example of how you might adapt the summarize_text
function:
python
def summarize_text(text, language="english"):
prompt = f"Please summarize the following {language} text in under 200 words while maintaining the key points and meaning:\n\n{text}"
# ... rest of the function
Remember to choose an appropriate LLM model that supports the desired languages.
How scalable is the Text Summarizer Web App for handling high volumes of requests?
The current implementation of the Text Summarizer Web App is designed as a command-line tool, which may not be suitable for high-volume requests. To make it more scalable: - Convert it into a web application using a framework like Flask or FastAPI. - Implement caching mechanisms to store frequently requested summaries. - Consider using asynchronous programming to handle multiple requests concurrently. - Deploy the application on a cloud platform that can auto-scale based on demand.
These enhancements would allow the Text Summarizer Web App to handle higher volumes of requests efficiently.
Created: | Last Updated:
Here's a step-by-step guide on how to use the Text Summarizer Web App template:
Introduction
The Text Summarizer Web App is a powerful tool that allows you to summarize text using AI technology. This template provides a simple command-line interface for summarizing text, which can be easily integrated into other applications or used as a standalone tool.
Getting Started
To begin using this template, follow these steps:
- Click the "Start with this Template" button in the Lazy Builder interface.
Test the Application
Once you've started with the template:
- Click the "Test" button in the Lazy Builder interface.
- This will launch the Lazy CLI and begin the deployment of your app.
Entering Input
After pressing the "Test" button, you'll be prompted to enter the text you want to summarize through the Lazy CLI:
- When prompted, enter or paste the text you want to summarize.
- Press Enter to submit the text.
Using the App
The app will process your input and provide a summary:
- The app will use AI to generate a summary of your text, aiming to capture the key points in under 200 words.
- The summary will be displayed in the CLI, formatted with dashes above and below for easy readability.
Integrating the App
This Text Summarizer can be easily integrated into other applications or workflows. Here's an example of how you might use it in a Python script:
```python import requests
def summarize_text(text): url = "YOUR_LAZY_APP_URL_HERE" # Replace with the URL provided by Lazy response = requests.post(url, json={"text": text}) if response.status_code == 200: return response.json()["summary"] else: return "Error: Unable to summarize text"
Example usage
text_to_summarize = "Your long text here..." summary = summarize_text(text_to_summarize) print(summary) ```
Remember to replace "YOUR_LAZY_APP_URL_HERE"
with the actual URL provided by Lazy when you deploy your app.
By following these steps, you'll be able to use the Text Summarizer Web App to quickly and efficiently summarize text using AI technology. This tool can be valuable for content creators, researchers, or anyone who needs to distill large amounts of text into concise summaries.
Template Benefits
-
Improved Productivity: This text summarization tool can help businesses quickly distill large volumes of text into concise summaries, saving time and improving efficiency in information processing and decision-making.
-
Enhanced Content Creation: Content creators and marketers can use this tool to generate brief summaries of longer articles or reports, making it easier to produce social media posts, email newsletters, or executive briefings.
-
Research Acceleration: Researchers and analysts can leverage this template to quickly summarize academic papers, market reports, or competitor analyses, allowing them to cover more ground in less time.
-
Customer Service Optimization: Support teams can use this tool to summarize lengthy customer inquiries or feedback, enabling faster response times and more efficient handling of customer issues.
-
Educational Support: Educational institutions and e-learning platforms can integrate this summarization tool to help students quickly grasp key concepts from textbooks, lectures, or online resources, enhancing the learning experience.
Technologies
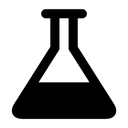
