Nylas Email Auth
import logging
from flask import Flask, url_for, request, session
from gunicorn.app.base import BaseApplication
from routes import routes as routes_blueprint
from authentication import auth, auth_required
from models import db, User
from abilities import apply_sqlite_migrations
# Setup logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
def create_app():
app = Flask(__name__, static_folder='static')
app.secret_key = 'supersecretkey'
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///database.sqlite'
db.init_app(app)
with app.app_context():
apply_sqlite_migrations(db.engine, db.Model, 'migrations')
app.register_blueprint(routes_blueprint)
app.register_blueprint(auth)
Frequently Asked Questions
What are some potential business applications for the Nylas Email Auth template?
The Nylas Email Auth template offers several business applications: - Email management systems for customer service teams - Personal productivity tools for professionals - Email analytics platforms for marketing teams - Automated email sorting and categorization for businesses - Integration of email functionality into existing business applications
By leveraging the Nylas API, this template provides a foundation for building robust email-centric solutions.
How can the Nylas Email Auth template improve workflow efficiency for businesses?
The Nylas Email Auth template can significantly enhance workflow efficiency by: - Providing quick access to recent emails without switching between applications - Enabling the development of custom email management tools tailored to specific business needs - Facilitating the creation of automated email processing systems - Allowing for seamless integration of email data into other business processes - Streamlining communication by centralizing email access within a custom application
What industries could benefit most from implementing the Nylas Email Auth template?
Several industries could greatly benefit from the Nylas Email Auth template: - Legal firms for managing client communications - Real estate agencies for tracking property inquiries - Customer support centers for efficient ticket management - Sales teams for monitoring lead communications - Human resources departments for managing job applications
The template's flexibility allows it to be adapted to various industry-specific email management needs.
How can I modify the Nylas Email Auth template to retrieve more than 10 emails?
To retrieve more than 10 emails, you can modify the list_emails_route
function in the routes.py
file. Specifically, change the limit
parameter in the Nylas client call:
python
messages = nylas_client.messages.list(user_data['nylas_token'], {"limit": 50}).data
This example increases the limit to 50 emails. You can adjust this number based on your needs, but be mindful of performance implications when requesting a large number of emails.
How can I add email sending functionality to the Nylas Email Auth template?
To add email sending functionality, you can create a new route in routes.py
that uses the Nylas API to send emails. Here's an example of how you might implement this:
```python @routes.route("/send_email", methods=['POST']) def send_email_route(): if 'user' not in session or 'user_email' not in session['user']: return jsonify({"error": "User not authenticated"}), 401
user_data = get_user_by_email(session['user']['user_email'])
if not user_data or not user_data.get('nylas_token'):
return jsonify({"error": "Gmail not connected"}), 400
nylas_client = Client(os.environ["NYLAS_API_KEY"])
try:
draft = nylas_client.drafts.create(user_data['nylas_token'])
draft.subject = request.json.get('subject')
draft.body = request.json.get('body')
draft.to = [{'email': request.json.get('to_email')}]
message = draft.send()
return jsonify({"message": "Email sent successfully", "message_id": message.id})
except Exception as e:
return jsonify({"error": f"Failed to send email: {str(e)}"}), 500
```
This new route allows authenticated users to send emails through their connected Gmail account using the Nylas API. Remember to handle the request data securely and validate inputs before sending.
Created: | Last Updated:
Here's a step-by-step guide for using the Nylas Email Auth template:
Introduction
This template creates an application that connects to Google Calendar via Nylas, displays the user's recent emails, and requires user authentication. The app provides a simple interface for users to connect their Gmail account and view their recent emails.
Getting Started
- Click "Start with this Template" to begin using this template in the Lazy Builder interface.
Initial Setup
-
Set up the following environment secrets in the Environment Secrets tab within the Lazy Builder:
-
NYLAS_CLIENT_ID
: Your Nylas application client ID NYLAS_API_KEY
: Your Nylas API key
To obtain these credentials: - Go to the Nylas Dashboard (https://dashboard.nylas.com/) - Create a new application or select an existing one - Navigate to the "API" section - Copy the "Client ID" and "API Key" values
Test the Application
- Click the "Test" button to start the deployment process and launch the Lazy CLI.
Using the Application
-
Once the app is deployed, you'll receive a server link to access the application's interface.
-
Open the provided link in your web browser to access the landing page.
-
Click the "Log in" button to authenticate.
-
After logging in, you'll be redirected to the home page.
-
If you haven't connected your Gmail account yet, you'll see a "Connect Gmail" button. Click it to start the OAuth process.
-
Follow the prompts to authorize the application to access your Gmail account.
-
Once connected, the app will display your recent emails on the home page.
Features
- The app shows a list of your recent emails, including the subject, sender, date, and a snippet of the content.
- Unread emails are highlighted and marked with a "New" badge.
- You can reconnect your calendar if needed using the "Reconnect Calendar" button.
This template provides a simple way to integrate Nylas email functionality into your application, allowing users to view their recent emails after authentication.
Here are 5 key business benefits for this template:
Template Benefits
-
Streamlined Email Integration: Easily connect and access Gmail accounts through Nylas API, allowing businesses to incorporate email functionality into their applications without complex email server setup.
-
Enhanced User Authentication: Implements secure user authentication and session management, ensuring that only authorized users can access sensitive email data and functionality.
-
Improved User Experience: Provides a clean, responsive interface for viewing recent emails, with clear visual indicators for unread messages and easy-to-read email previews.
-
Scalable Architecture: Built with Flask and Gunicorn, the template offers a solid foundation for building scalable web applications that can handle increased user loads.
-
Customizable Design: Includes CSS templates for easy customization of the user interface, allowing businesses to tailor the look and feel to match their brand identity.
Technologies
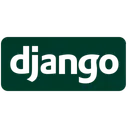
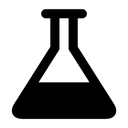

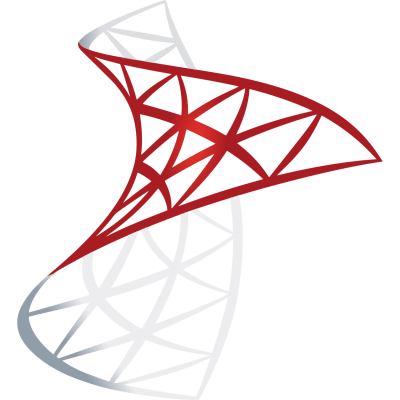