by Lazy Sloth
Login and Registration
from forms import AccountForm
import logging
from flask import session
from flask import render_template, request, redirect, url_for, flash
from app_init import app
from models import db, User
from forms import LoginForm, RegistrationForm
# Removed Flask app initialization here. It will be initialized in app_init.py and imported.
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///users.db'
app.config['SECRET_KEY'] = 'a_very_secret_key'
db.init_app(app)
with app.app_context():
db.create_all()
@app.route("/account", methods=['GET', 'POST'])
def account():
if 'username' not in session:
flash('You must be logged in to view this page.')
return redirect(url_for('login'))
form = AccountForm()
Login and Registration
Created: | Last Updated:
Introduction to the Login and Registration Template
This template provides a robust starting point for applications that require user authentication, including features for user registration, login, account settings, and password strength checking. It's built with Flask, a lightweight WSGI web application framework, and uses Tailwind CSS for styling. This template is ideal for builders looking to create software applications on the Lazy platform that require user management capabilities.
Getting Started with the Template
To begin using this template, simply click on "Start with this Template" on the Lazy platform. This will pre-populate the code in the Lazy Builder interface, so you won't need to copy, paste, or delete any code manually.
Initial Setup
Before testing the application, ensure that you have set up the necessary environment secrets if the template requires them. For this template, you do not need to set up any environment secrets as they are already configured within the code.
Test: Pressing the Test Button
Once you're ready, press the "Test" button on the Lazy platform. This will begin the deployment of the app and launch the Lazy CLI. The Lazy platform handles all deployment aspects, so you don't need to worry about installing libraries or setting up your environment.
Entering Input
If the template requires user input, the Lazy CLI will prompt you for it after you press the "Test" button. For this template, you will be prompted to enter details such as username, email, and password when registering a new user or logging in.
Using the App
After deployment, the app will provide a frontend interface where users can register, log in, and manage their account settings. The interface is user-friendly and styled with Tailwind CSS for a modern look and feel.
Integrating the App
If you need to integrate this app with external tools or services, you may need to use the server link provided by Lazy after deployment. For example, if you want to connect the user authentication system with another application, you can use the provided server link to make API requests for registering or logging in users.
Remember, this template is a starting point, and you can customize it further to fit the specific needs of your application. Whether you need to add more fields to the user model, implement additional security measures, or integrate with other services, this template gives you a solid foundation to build upon.
For any further customization or integration, refer to the documentation or sample code provided within the template to guide you through the process.
Technologies
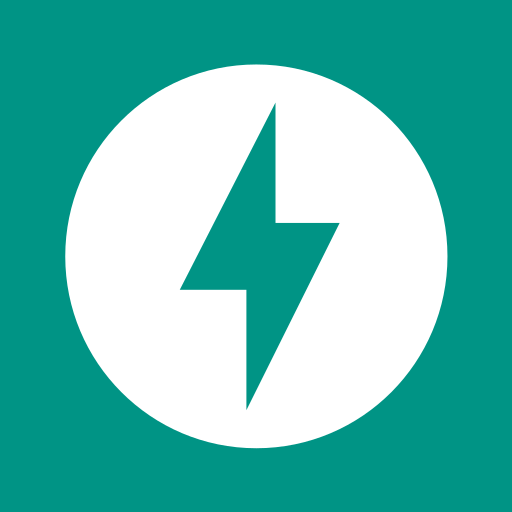
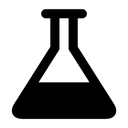
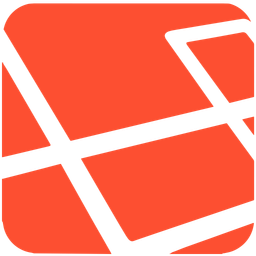