by Lazy Sloth
Create Price with Stripe API & Flask
import os
from flask import Flask, jsonify, request, render_template_string
# Set the Stripe API key from environment variables
stripe_api_key = os.environ.get('STRIPE_API_KEY')
if not stripe_api_key:
raise ValueError("The STRIPE_API_KEY environment variable is not set.")
import stripe
stripe.api_key = stripe_api_key
app = Flask(__name__)
# HTML form for the frontend
HTML_FORM = '''
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Create Price Object</title>
</head>
<body>
Frequently Asked Questions
What business problem does this Create Price with Stripe API template solve?
The Create Price with Stripe API template addresses the need for businesses to dynamically create and manage pricing for their products or services. It allows companies to easily integrate Stripe's pricing functionality into their existing systems, enabling them to quickly adjust prices, create new price points, or set up different pricing tiers without manual intervention in their payment system.
How can this template be customized for different business models?
The Create Price with Stripe API template can be adapted for various business models: - Subscription-based services can use it to create different subscription tiers. - E-commerce platforms can dynamically adjust prices based on inventory or demand. - SaaS companies can implement usage-based pricing by creating prices tied to specific usage metrics. - Event organizers can set up tiered pricing for early bird, regular, and VIP tickets.
What are the security considerations when using this template in a production environment?
When using the Create Price with Stripe API template in production, consider the following: - Ensure the Stripe API key is securely stored and not exposed in the code. - Implement proper authentication and authorization to prevent unauthorized price creation. - Use HTTPS to encrypt data in transit. - Validate and sanitize all user inputs to prevent injection attacks. - Implement rate limiting to prevent abuse of the price creation endpoint.
How can I modify the template to include additional price attributes supported by Stripe?
To include additional price attributes, you can modify both the HTML form and the Flask route. For example, to add a recurring
attribute for subscription pricing:
In the HTML form, add:
html
<label for="recurring">Recurring:</label>
<select id="recurring" name="recurring">
<option value="month">Monthly</option>
<option value="year">Yearly</option>
</select>
In the Flask route, modify the stripe.Price.create()
call:
python
price = stripe.Price.create(
product=data['product'],
unit_amount=int(data['unit_amount']),
currency=data['currency'],
recurring={"interval": data['recurring']}
)
Remember to update the JavaScript to include the new field when sending data to the server.
Can this template be extended to handle bulk price creation?
Yes, the Create Price with Stripe API template can be extended to handle bulk price creation. You would need to modify the frontend to accept multiple price inputs and update the backend to process them. Here's a basic example of how you could modify the Flask route:
python
@app.route('/create_bulk_prices', methods=['POST'])
def create_bulk_prices():
try:
data = request.json
prices = []
for price_data in data['prices']:
price = stripe.Price.create(
product=price_data['product'],
unit_amount=int(price_data['unit_amount']),
currency=price_data['currency'],
)
prices.append(price)
return jsonify(prices), 200
except Exception as e:
return jsonify(error=str(e)), 400
This modification allows the Create Price with Stripe API template to create multiple price objects in a single API call, which can be useful for businesses that need to set up pricing for multiple products or services simultaneously.
Created: | Last Updated:
Introduction to the Create Price with Stripe API Template
Welcome to the Create Price with Stripe API Template! This template is designed to help you integrate Stripe's pricing functionality into your applications with ease. By using this template, you can create price objects for your products or services directly through a user-friendly web interface. This is particularly useful for builders looking to add e-commerce capabilities to their software without delving into the complexities of Stripe's API.
Clicking Start with this Template
To begin using this template, simply click on the "Start with this Template" button. This will set up the template in your Lazy builder interface, pre-populating the code so you can start customizing and deploying your application right away.
Initial setup: Adding Environment Secrets
Before you can use this template, you'll need to set up an environment secret for the Stripe API key. Here's how to do it:
- Log in to your Stripe account and navigate to the API keys section.
- Copy your Stripe API key. If you don't have one, you'll need to create it.
- In the Lazy Builder, go to the Environment Secrets tab.
- Create a new secret with the key as
STRIPE_API_KEY
and paste your Stripe API key as the value.
With the environment secret in place, your application will be able to authenticate with Stripe's services.
Test: Pressing the Test Button
Once you have set up your environment secret, press the "Test" button to begin the deployment of your application. The Lazy CLI will handle the deployment process, and you won't need to worry about installing libraries or setting up your environment.
Using the App
After deployment, Lazy will provide you with a dedicated server link to access your application's interface. Here's how to use it:
- Open the provided server link in your web browser.
- You will see a form where you can enter the Product ID, Unit Amount, and Currency to create a new price object.
- Fill in the details and click "Create Price".
- The app will display the result below the form, indicating whether the price object was created successfully or if there was an error.
This interface allows you to interact with the Stripe API to create price objects without writing any additional code.
Integrating the App
If you wish to integrate this functionality into another service or frontend, you can use the provided endpoint /create_price
. Here's a sample request you might make to this endpoint:
POST /create_price HTTP/1.1<br>
Host: [Your Lazy Server Link]<br>
Content-Type: application/json<br>
<br>
{<br>
"product": "prod_XXXXXXXXXXXXXX",<br>
"unit_amount": 2000,<br>
"currency": "usd"<br>
}
And here's a sample response you might receive:
{<br>
"id": "price_1XXXXXXXXXXXXXX",<br>
"object": "price",<br>
"billing_scheme": "per_unit",<br>
...<br>
}
Use the server link provided by Lazy to make API calls from your external tools or services. Ensure that you handle the API responses appropriately in your application's code.
By following these steps, you can seamlessly integrate Stripe's pricing functionality into your applications using the Create Price with Stripe API Template on the Lazy platform.
Here are 5 key business benefits for this template:
Template Benefits
-
Streamlined Pricing Management: This template allows businesses to quickly create and manage price objects for their products or services through Stripe, enabling efficient pricing updates and flexibility.
-
Easy Integration: The template provides a simple way to integrate Stripe's pricing functionality into existing web applications, reducing development time and complexity.
-
Improved User Experience: By offering a user-friendly interface for creating price objects, businesses can empower non-technical staff to manage pricing without direct access to the Stripe dashboard.
-
Real-time Price Creation: The template enables instant price object creation and confirmation, allowing businesses to respond quickly to market changes or implement dynamic pricing strategies.
-
Secure API Handling: By using environment variables for API keys and implementing proper error handling, the template promotes secure interactions with the Stripe API, helping businesses maintain data security and comply with best practices.
Technologies
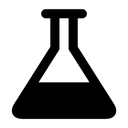
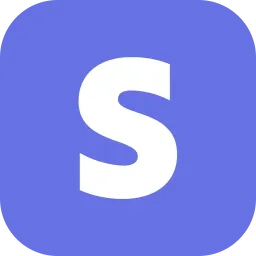