by Lazy Sloth
Create Product using Stripe API & Flask
import os
from flask import Flask, jsonify, render_template_string, request
# Set the Stripe API key from environment variables
stripe_api_key = os.environ.get('STRIPE_API_KEY')
if not stripe_api_key:
raise ValueError("The STRIPE_API_KEY environment variable is not set.")
import stripe
stripe.api_key = stripe_api_key
app = Flask(__name__)
# HTML content for creating a product with a price
HTML_CONTENT = '''
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Create a Stripe Product with Price</title>
</head>
<body>
Frequently Asked Questions
What business problem does this "Create Product using Stripe API" template solve?
This template addresses the need for businesses to quickly and easily create products and their associated prices in their Stripe account. It's particularly useful for e-commerce platforms, subscription-based services, or any business that needs to dynamically add new products to their Stripe catalog without directly accessing the Stripe dashboard.
How can this template be customized for different types of products?
The "Create Product using Stripe API" template can be easily customized to accommodate various product types. For instance, you could add fields for product images, metadata, or custom attributes. For subscription products, you could modify the form to include recurring interval information. Here's an example of how you might extend the form for a subscription product:
html
<input type="text" id="interval" name="interval" placeholder="Billing Interval (month/year)" required />
<input type="number" id="interval_count" name="interval_count" placeholder="Interval Count" required />
Then, in the backend, you would create a recurring price instead of a one-time price.
What are the potential business applications of this template beyond e-commerce?
While the "Create Product using Stripe API" template is immediately applicable to e-commerce, it has broader applications. It could be used in: - SaaS platforms for creating new service tiers - Event management systems for creating ticketed events - Digital content platforms for pricing new content or courses - Marketplace applications where sellers can create and price their own products - Donation platforms for creating different donation tiers
How can error handling be improved in this template?
Error handling in the "Create Product using Stripe API" template can be enhanced by adding more specific error checks and providing more detailed feedback. Here's an example of how you might improve the error handling in the create_product route:
```python @app.route('/create_product', methods=['POST']) def create_product(): try: name = request.json['name'] amount = int(request.json['amount']) currency = request.json['currency']
if not name or amount <= 0 or not currency:
return jsonify(error="Invalid input data"), 400
# Create product and price as before...
except KeyError as e:
return jsonify(error=f"Missing required field: {str(e)}"), 400
except ValueError as e:
return jsonify(error=f"Invalid value: {str(e)}"), 400
except stripe.error.StripeError as e:
return jsonify(error=f"Stripe API error: {str(e)}"), 500
except Exception as e:
return jsonify(error=f"Unexpected error: {str(e)}"), 500
```
How can businesses ensure the security of their Stripe API key when using this template?
Security of the Stripe API key is crucial when using the "Create Product using Stripe API" template. Best practices include: - Never hardcode the API key in the source code - Use environment variables to store the API key, as demonstrated in the template - Ensure that the API key is not exposed in client-side code or logs - Use Stripe's test mode and test API keys during development - Implement proper authentication and authorization in your application to control who can access the product creation functionality - Regularly rotate your API keys and monitor your Stripe account for any suspicious activity
Created: | Last Updated:
Introduction to the Stripe Product Creation Template
Welcome to the Stripe Product Creation Template! This template is designed to help you seamlessly create products and their associated prices using the Stripe API. It includes a Flask web service with an endpoint that facilitates the creation of a product and its price on Stripe based on the data submitted through a simple web form. This is an excellent tool for builders looking to integrate Stripe's payment processing capabilities into their applications without delving into the complexities of API calls and backend coding.
Getting Started
To begin using this template, click on "Start with this Template" in the Lazy builder interface. This will pre-populate the code in the Lazy Builder, so you won't need to copy or paste any code manually.
Initial Setup
Before you can test and use the app, you'll need to set up an environment secret for the Stripe API key. Here's how to do it:
- Log in to your Stripe account and navigate to the API section to find your API keys.
- Copy the secret key.
- In the Lazy Builder interface, go to the Environment Secrets tab.
- Create a new secret with the key
STRIPE_API_KEY
and paste your Stripe secret key as the value.
This step is crucial as the application uses the Stripe API key to authenticate requests to Stripe's servers.
Test: Pressing the Test Button
Once you have set up the environment secret, press the "Test" button in the Lazy builder. This will deploy your application and launch the Lazy CLI. No user input is required at this stage.
Using the App
After pressing the "Test" button, Lazy will provide you with a dedicated server link. Use this link to access the web interface for creating a Stripe product. The interface includes a form where you can enter the product name, description, price in cents, and currency. Once you submit the form, the backend will process the request and create the product and price in your Stripe account.
Integrating the App
If you wish to integrate this functionality into an existing service or frontend, you can use the provided server link as the endpoint for your product creation form. Ensure that the form data is sent to the /create_product
route as a POST request with JSON content.
Here's a sample request you might use in your external tool:
`POST /create_product HTTP/1.1
Host: [Your Lazy Server Link]
Content-Type: application/json
{
"name": "Sample Product",
"description": "This is a sample product",
"amount": 1000,
"currency": "usd"
}`
And a sample response you would receive:
{
"product_id": "prod_XXXXXXXXXXXXXX",
"price_id": "price_XXXXXXXXXXXXXX"
}
Remember to replace [Your Lazy Server Link]
with the actual link provided by Lazy after deployment.
By following these steps, you should now have a fully functional Stripe product creation tool at your disposal. Happy building!
Template Benefits
-
Streamlined Product Management: This template allows businesses to quickly create and price new products in their Stripe account, streamlining the process of expanding their product catalog without leaving their own application.
-
Integration-Ready E-commerce Solution: By providing a ready-to-use interface for Stripe product creation, this template serves as a foundation for building more complex e-commerce systems, reducing development time and costs.
-
Customizable Pricing Strategy: The ability to set prices in various currencies enables businesses to implement flexible pricing strategies for different markets or customer segments, potentially increasing global reach and revenue.
-
Improved Operational Efficiency: Automating the product creation process through this API integration reduces manual data entry, minimizing errors and freeing up staff time for more strategic tasks.
-
Enhanced Developer Experience: The template offers a clear structure for interacting with Stripe's API, making it easier for developers to understand and extend the functionality, potentially speeding up feature development and reducing onboarding time for new team members.
Technologies
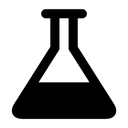
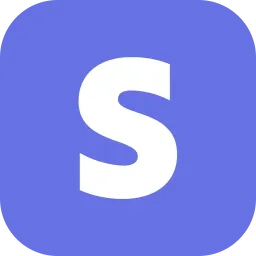