by Lazy Sloth
To-Do App on Flask
from flask import Flask, render_template, request, redirect, url_for
import logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
app = Flask(__name__)
from flask_sqlalchemy import SQLAlchemy
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///tasks.db'
app.config['SQLALCHEMY_TRACK_MODIFICATIONS'] = False
db = SQLAlchemy(app)
class Task(db.Model):
id = db.Column(db.Integer, primary_key=True)
description = db.Column(db.String(255), nullable=False)
completed = db.Column(db.Boolean, default=False)
with app.app_context():
db.create_all()
@app.route("/", methods=["GET", "POST"])
def home():
Frequently Asked Questions
How can this To-Do App template be customized for business use?
The To-Do App template can be easily customized for various business applications. For example, you could adapt it for project management by adding categories for different projects, assigning tasks to team members, or including priority levels. You could also integrate it with a company's existing systems, such as adding a feature to sync tasks with a company calendar or email system. The flexible nature of the To-Do App allows for seamless integration of business-specific features.
What industries could benefit most from implementing this To-Do App?
The To-Do App template is versatile and can benefit various industries. It's particularly useful for: - Software development teams for sprint planning and bug tracking - Marketing agencies for campaign task management - Educational institutions for assignment tracking - Freelancers for managing client projects - Retail businesses for inventory and restocking tasks The To-Do App's adaptability makes it a valuable tool across multiple sectors.
How can the To-Do App improve productivity in a business setting?
The To-Do App can significantly boost productivity by: - Providing a clear, organized view of tasks - Allowing easy prioritization and categorization of tasks - Enabling team collaboration and task delegation - Offering visual cues for task completion status - Facilitating quick addition and modification of tasks By implementing the To-Do App, businesses can streamline their workflow, reduce time spent on task management, and increase overall efficiency.
How can I add a due date feature to tasks in the To-Do App?
To add a due date feature, you'll need to modify the Task model, form, and template. Here's an example of how to update the Task model in models.py
:
```python from datetime import datetime
class Task(db.Model): id = db.Column(db.Integer, primary_key=True) description = db.Column(db.String(255), nullable=False) completed = db.Column(db.Boolean, default=False) due_date = db.Column(db.DateTime, nullable=True) ```
Then, update the form in home.html
to include a date input:
html
<input type="date" name="due_date" id="due_date">
Finally, modify the route in main.py
to handle the due date:
python
@app.route("/", methods=["GET", "POST"])
def home():
if request.method == "POST":
task_content = request.form.get("task")
due_date_str = request.form.get("due_date")
if task_content:
due_date = datetime.strptime(due_date_str, '%Y-%m-%d') if due_date_str else None
new_task = Task(description=task_content, due_date=due_date)
db.session.add(new_task)
db.session.commit()
return redirect(url_for("home"))
tasks = Task.query.all()
return render_template("home.html", tasks=tasks)
This modification allows users to set and track due dates for tasks in the To-Do App.
How can I implement task categories in the To-Do App?
To implement task categories, you'll need to create a new model for categories and establish a relationship with tasks. Here's how you can do it:
In models.py
, add a new Category model and update the Task model:
```python class Category(db.Model): id = db.Column(db.Integer, primary_key=True) name = db.Column(db.String(50), nullable=False)
class Task(db.Model): id = db.Column(db.Integer, primary_key=True) description = db.Column(db.String(255), nullable=False) completed = db.Column(db.Boolean, default=False) category_id = db.Column(db.Integer, db.ForeignKey('category.id'), nullable=True) category = db.relationship('Category', backref=db.backref('tasks', lazy=True)) ```
Then, update the form in home.html
to include a category dropdown:
html
<select name="category" id="category">
{% for category in categories %}
<option value="{{ category.id }}">{{ category.name }}</option>
{% endfor %}
</select>
Finally, modify the route in main.py
to handle categories:
python
@app.route("/", methods=["GET", "POST"])
def home():
if request.method == "POST":
task_content = request.form.get("task")
category_id = request.form.get("category")
if task_content:
new_task = Task(description=task_content, category_id=category_id)
db.session.add(new_task)
db.session.commit()
return redirect(url_for("home"))
tasks = Task.query.all()
categories = Category.query.all()
return render_template("home.html", tasks=tasks, categories=categories)
These changes will allow users to categorize tasks in the To-Do App, making it more organized and efficient for managing various types of tasks.
Created: | Last Updated:
Introduction to the To-Do App Template
Welcome to the To-Do App template on Lazy! This template is a fantastic starting point for anyone looking to build a feature-rich task management system. With this template, you can add, edit, delete, and prioritize tasks with ease. The application is built using Flask and SQLAlchemy for backend operations, and the user interface is designed with Tailwind CSS for a responsive and visually appealing experience. Whether you're looking to create a simple to-do list or a more complex project management tool, this template provides a solid foundation that can be customized and expanded to fit your needs.
Getting Started
To begin using this template, simply click on "Start with this Template" on the Lazy platform. This will set up the code in the Lazy Builder interface, so you can start customizing and testing your application right away.
Initial Setup
There's no need to worry about environment variables for this template. Lazy handles all the deployment details, so you can focus on building your application.
Test: Deploying the App
Once you're ready to see your application in action, press the "Test" button. This will deploy your app and launch the Lazy CLI. If the application requires any user input, you will be prompted to provide it through the Lazy CLI interface.
Entering Input
For this To-Do App, there is no need for initial user input through the CLI. All interactions with the app will be through the web interface once it is running.
Using the App
After deploying the app, you will be provided with a dedicated server link to access your To-Do List app's interface. Here's how you can interact with the app:
- Add a new task by entering the task description in the provided input field and clicking the "Add Task" button.
- Edit an existing task by clicking the "Edit" button next to the task, making changes, and submitting the update.
- Delete a task by clicking the "Delete" button next to the task you wish to remove.
- Mark a task as complete by clicking the "Complete" button, or mark it as incomplete by clicking the "Mark as Incomplete" button.
The changes you make will be reflected in real-time, and the tasks' statuses will be updated accordingly in the database.
Integrating the App
If you wish to integrate this To-Do App with other tools or services, you may need to use the server link provided by Lazy. For example, if you want to embed the To-Do List into another webpage, you can use an iframe with the server link as the source.
Here's a sample code snippet for embedding the To-Do List:
<iframe src="YOUR_SERVER_LINK" width="100%" height="600"></iframe>
Replace "YOUR_SERVER_LINK" with the actual link provided by Lazy after deploying your app.
If you need to interact with the app's backend directly, you can use the server link to make HTTP requests to the endpoints defined in the Flask application.
That's it! You now have a fully functional To-Do List app ready to use and integrate. Enjoy building with Lazy!
Here are the top 5 business benefits or applications of this To-Do App template:
Template Benefits
-
Improved Productivity Management: This template provides a solid foundation for businesses to create a task management system, helping employees organize their work, prioritize tasks, and meet deadlines more effectively.
-
Scalable Project Management Tool: With its modular design, the template can be easily expanded to include project management features, making it suitable for small to medium-sized businesses looking for a customizable project tracking solution.
-
Team Collaboration Enhancement: By adding user authentication and task sharing capabilities, this template can be transformed into a collaborative platform, improving communication and coordination among team members.
-
Cost-Effective Custom Solution: Instead of purchasing expensive off-the-shelf task management software, businesses can use this template as a starting point to develop a tailored solution that fits their specific needs and workflows.
-
Data-Driven Decision Making: The SQLAlchemy integration allows for easy data collection and analysis of task completion rates and productivity trends, enabling managers to make informed decisions about resource allocation and process improvements.
Technologies
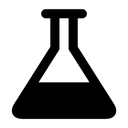