by notyethigh
Image URL Finder for CSV
import logging
from gunicorn.app.base import BaseApplication
from app_init import create_initialized_flask_app
# Setup logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
# Flask app creation should be done by create_initialized_flask_app to avoid circular dependency problems.
app = create_initialized_flask_app()
class StandaloneApplication(BaseApplication):
def __init__(self, app, options=None):
self.application = app
self.options = options or {}
super().__init__()
def load_config(self):
# Apply configuration to Gunicorn
for key, value in self.options.items():
if key in self.cfg.settings and value is not None:
self.cfg.set(key.lower(), value)
def load(self):
Frequently Asked Questions
What is the main purpose of the Image URL Finder for CSV tool?
The Image URL Finder for CSV is a public web tool designed to enhance CSV files containing names by automatically finding and inserting relevant image URLs for each name. This tool streamlines the process of associating visual content with named entities in datasets, which can be particularly useful for businesses dealing with large lists of people, products, or places.
How can businesses benefit from using this Image URL Finder tool?
Businesses can leverage the Image URL Finder for CSV in several ways: - E-commerce: Quickly find product images for inventory lists. - HR departments: Add profile pictures to employee directories. - Marketing teams: Enhance contact lists with visual content for personalized campaigns. - Event planners: Easily add speaker or performer images to event programs. - Real estate agencies: Automatically find property images for listing databases.
Is the Image URL Finder for CSV tool suitable for handling sensitive or proprietary data?
While the Image URL Finder for CSV is a powerful tool, it's important to note that it's a public web application. For sensitive or proprietary data, businesses should exercise caution. The tool uses external image search APIs, so it's not recommended for confidential information. For such cases, companies might consider developing a private, on-premises version of the tool with additional security measures.
How does the Image URL Finder handle the CSV file processing on the backend?
The Image URL Finder processes CSV files using Python's built-in csv
module. Here's a simplified version of the core processing logic:
```python def process_csv(): file = request.files['file'] stream = io.StringIO(file.stream.read().decode("UTF8"), newline=None) csv_input = csv.reader(stream)
processed_rows = []
header = next(csv_input)
header.append('Image URL')
processed_rows.append(header)
for row in csv_input:
if row:
name = row[0].strip()
image_url = search_image_for_name(name)
row.append(image_url)
processed_rows.append(row)
# Create and return the processed CSV
output = io.StringIO()
writer = csv.writer(output)
writer.writerows(processed_rows)
return send_file(io.BytesIO(output.getvalue().encode('utf-8')),
mimetype='text/csv',
as_attachment=True,
download_name='processed_' + file.filename)
```
This function reads the uploaded CSV, processes each row to find an image URL, and creates a new CSV with the added image URLs.
Can the Image URL Finder be customized to use different image search APIs?
Yes, the Image URL Finder for CSV can be customized to use different image search APIs. Currently, it uses SerpAPI, but you can modify the search_image_for_name
function to use any API of your choice. Here's an example of how you might adapt it to use a hypothetical "CustomImageAPI":
```python import requests
def search_image_for_name(name): try: api_key = os.environ['CUSTOM_IMAGE_API_KEY'] url = "https://api.customimageapi.com/search" params = { "query": name, "api_key": api_key, "limit": 1 }
response = requests.get(url, params=params)
response.raise_for_status()
data = response.json()
if 'results' in data and data['results']:
return data['results'][0]['image_url']
return "https://example.com/not-found-image.jpg"
except Exception as e:
logger.error(f"Error searching image for {name}: {str(e)}")
return "https://example.com/not-found-image.jpg"
```
By modifying this function, you can easily integrate different image search APIs into the Image URL Finder for CSV tool, allowing for greater flexibility and customization based on specific business needs or preferences.
Created: | Last Updated:
Here's a step-by-step guide for using the Image URL Finder for CSV template:
Introduction
The Image URL Finder for CSV is a powerful tool that allows you to upload a CSV file containing names and automatically find relevant image URLs for each name. This template provides a user-friendly web interface for processing CSV files and enhancing them with image data.
Getting Started
To begin using this template, follow these steps:
- Click "Start with this Template" in the Lazy Builder interface.
Setting Up Environment Secrets
Before running the app, you need to set up an environment secret:
- Navigate to the Environment Secrets tab in the Lazy Builder.
- Add a new secret with the key
SERPAPI_KEY
. - To obtain a SerpAPI key:
- Go to https://serpapi.com/
- Sign up for an account if you don't have one
- Once logged in, navigate to your dashboard
-
Copy your API key from the dashboard
-
Paste your SerpAPI key as the value for the
SERPAPI_KEY
secret in the Lazy Builder.
Testing the App
Once you've set up the environment secret:
- Click the "Test" button in the Lazy Builder interface.
- Wait for the app to deploy and start.
Using the App
After the app has started:
- Lazy will provide you with a server link to access the web interface.
- Open the provided link in your web browser.
- You'll see a simple interface with a file upload area.
- Prepare a CSV file with names in the first column.
- Either drag and drop your CSV file into the upload area or click to select the file.
- Once a file is selected, click the "Process CSV" button.
- The app will process your file, searching for image URLs for each name.
- After processing, a new CSV file will be downloaded automatically.
- The downloaded CSV will contain all original data plus a new column with image URLs.
Integrating the App
This app is designed to be used as a standalone web tool and doesn't require integration with external services. Users can simply access the provided web interface to process their CSV files and obtain image URLs for names.
By following these steps, you'll be able to use the Image URL Finder for CSV template to enhance your CSV files with relevant image data quickly and easily.
Template Benefits
-
Automated Image Search: This template provides a streamlined process for finding relevant images for names in bulk, saving businesses significant time and manual effort in image research and curation.
-
Enhanced Data Enrichment: By automatically adding image URLs to CSV files, the tool enriches existing datasets, making them more valuable for various applications such as customer profiles, employee directories, or product catalogs.
-
Improved User Experience: The intuitive drag-and-drop interface and progress indicators make the tool accessible to non-technical users, enabling wider adoption across different departments within an organization.
-
Scalability and Performance: The use of Gunicorn with multiple workers and threads allows the application to handle concurrent requests efficiently, making it suitable for high-traffic scenarios or processing large CSV files.
-
Customization and Integration: The modular structure of the code allows for easy customization and integration with existing systems, enabling businesses to tailor the tool to their specific needs or incorporate it into larger workflows.
Technologies
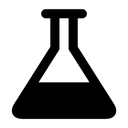
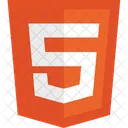


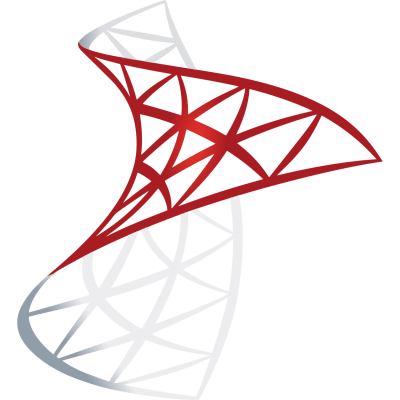