by Lazy Sloth
Open AI GPT-4 Exam Generator
from flask import Flask, request, render_template, redirect, url_for
import logging
from gunicorn.app.base import BaseApplication
from upload import handle_upload_file
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
from app_init import app
@app.route("/", methods=['GET'])
def home_route():
generated_exams = get_generated_exams_count()
return render_template("home.html", generated_exams=generated_exams)
@app.route("/upload", methods=["POST"])
def upload_file():
return handle_upload_file()
class StandaloneApplication(BaseApplication):
def __init__(self, app, options=None):
self.application = app
self.options = options or {}
super().__init__()
Frequently Asked Questions
How can businesses benefit from using the GPT-4 Exam Generator?
The GPT-4 Exam Generator offers several benefits for businesses, particularly in the education and training sectors:
- Time-saving: It automates the process of creating new exam papers, reducing the workload for educators and trainers.
- Consistency: The generator maintains a consistent style based on the uploaded past exam, ensuring uniformity in assessment methods.
- Scalability: Businesses can quickly generate multiple unique exams for different courses or training programs.
- Cost-effective: By reducing the time and resources needed for exam creation, it can lead to significant cost savings.
- Customization: The "Boost mathematical accuracy" option allows for tailoring the output to specific subject needs.
What are the potential applications of the GPT-4 Exam Generator outside of traditional education?
The GPT-4 Exam Generator has versatile applications beyond traditional education:
- Corporate Training: Companies can use it to create assessments for employee training programs.
- Certification Programs: Professional organizations can generate varied exam papers for certification processes.
- Self-assessment Tools: Online learning platforms can offer auto-generated practice exams to their users.
- Market Research: Businesses can adapt the tool to create surveys or questionnaires based on previous successful formats.
- Quality Control: It can be used to generate inspection checklists or quality assurance tests in manufacturing or service industries.
How does the GPT-4 Exam Generator ensure the security and privacy of uploaded exam papers?
The GPT-4 Exam Generator implements several security measures:
- Temporary Storage: Uploaded files are saved in a temporary folder ("/tmp") and are not permanently stored.
- File Type Restriction: Only PDF files are allowed, reducing the risk of malicious file uploads.
- Secure File Handling: The app uses the
secure_filename
function from Werkzeug to sanitize filenames. - Limited File Size: The app checks if the uploaded PDF has more than 15 pages, preventing excessively large files.
- Data Minimization: The generated exam is created without storing the content of the original exam.
How can I modify the GPT-4 Exam Generator to include custom styling for the generated PDF?
To include custom styling for the generated PDF, you can modify the process_pdf
function in the upload.py
file. Here's an example of how you might add a custom header to each page:
```python from reportlab.lib.styles import getSampleStyleSheet from reportlab.platypus import Paragraph, Frame
def process_pdf(file_path, contains_math=False): # ... existing code ...
def add_custom_header(canvas, doc):
canvas.saveState()
styles = getSampleStyleSheet()
header_text = "GPT-4 Generated Exam"
P = Paragraph(header_text, styles['Heading2'])
w, h = P.wrap(doc.width, doc.topMargin)
P.drawOn(canvas, doc.leftMargin, doc.height + doc.topMargin - h)
canvas.restoreState()
c = canvas.Canvas(new_pdf_path, pagesize=letter)
c.setPageTemplates([PageTemplate(frames=Frame(inch, inch, 6*inch, 9*inch), onPage=add_custom_header)])
# ... rest of the existing code ...
```
This modification adds a custom header to each page of the generated PDF.
Can the GPT-4 Exam Generator be integrated with a database to store exam statistics?
Yes, the GPT-4 Exam Generator already includes basic database integration for storing exam generation statistics. To expand this functionality, you can modify the database.py
file and the related functions in main.py
and upload.py
. Here's an example of how you might add a function to store more detailed exam information:
```python # In database.py def store_exam_details(conn, exam_type, question_count, math_enabled): sql = ''' INSERT INTO exam_details(exam_type,question_count,math_enabled) VALUES(?,?,?) ''' cur = conn.cursor() cur.execute(sql, (exam_type, question_count, math_enabled)) conn.commit() return cur.lastrowid
# In upload.py from database import store_exam_details
def process_pdf(file_path, contains_math=False): # ... existing code ...
# After generating new questions
conn = create_connection("./pythonsqlite.db")
exam_type = "Generated Exam" # You might want to determine this based on the content
question_count = len(new_questions.split("\n"))
store_exam_details(conn, exam_type, question_count, contains_math)
conn.close()
# ... rest of the existing code ...
```
This modification would store additional details about each generated exam in the database, allowing for more comprehensive analytics and reporting.
Created: | Last Updated:
How to Use the Exam Generator Template on Lazy
Introduction to the Exam Generator Template
The Exam Generator template is a powerful tool for educators and students alike, allowing you to generate a new exam PDF based on an uploaded PDF of a past exam. This template uses a web interface for file upload and displays the generated exam for download. It's perfect for creating practice exams or preparing for upcoming tests.
Getting Started with the Exam Generator Template
To begin using this template, simply click on Start with this Template on the Lazy platform. This will set up the template in your Lazy Builder interface, pre-populating the code so you can start customizing or testing right away.
Initial Setup
No environment secrets setup is required for this template. All necessary libraries and dependencies are handled by Lazy, ensuring a seamless experience.
Test: Deploying the App
Once you're ready to see the Exam Generator in action, press the Test button. This will begin the deployment of your app and launch the Lazy CLI. The Lazy platform will handle the deployment process, so you won't need to worry about setting up your environment or installing any libraries.
Entering Input
If the template requires user input, you will be prompted to provide it through the Lazy CLI after pressing the Test button. Follow the prompts in the CLI to input any required information.
Using the App
After deployment, Lazy will provide you with a dedicated server link to access the web interface of the Exam Generator. Here's how to use it:
- Visit the provided server link to access the Exam Generator's web interface.
- Follow the on-screen instructions to upload a PDF of a past exam.
- Use the toggle switch to enable or disable the experimental feature for boosting mathematical accuracy.
- Click on the "Create new exam" button to generate your exam.
- Once the exam is generated, you will see a link to download the new exam PDF.
Integrating the App
If you wish to integrate the Exam Generator into another service or frontend, you may need to use the server link provided by Lazy. For example, you could embed the link in an educational platform or share it with students for easy access to the exam generation feature.
Here's a sample request you might make to the server to upload a PDF and generate a new exam:
POST /upload HTTP/1.1<br>
Host: [Your Lazy Server Link]<br>
Content-Type: multipart/form-data; boundary=----WebKitFormBoundary7MA4YWxkTrZu0gW<br>
------WebKitFormBoundary7MA4YWxkTrZu0gW<br>
Content-Disposition: form-data; name="file"; filename="past_exam.pdf"<br>
Content-Type: application/pdf<br>
<br>
[PDF data]<br>
------WebKitFormBoundary7MA4YWxkTrZu0gW--<br>
And here's a sample response you might receive:
HTTP/1.1 200 OK<br>
Content-Type: application/json<br>
<br>
{<br>
"link": "https://[Your Lazy Server Link]/download/generated_exam.pdf"<br>
}
Remember, the actual server link and the endpoints will be provided by Lazy after you press the Test button.
Template Benefits
-
Efficient Exam Creation: Educators can quickly generate new exam papers based on past exams, saving significant time and effort in the exam preparation process.
-
Consistent Quality: The use of GPT-4 ensures that generated exams maintain a consistent level of quality and difficulty, aligning with the standards set by previous exams.
-
Customizable Learning Assessment: The option to boost mathematical accuracy allows for tailored exam generation, making it suitable for various subjects and difficulty levels.
-
Reduced Bias and Increased Variety: AI-generated exams can help reduce human bias in question creation and introduce more variety in exam content, potentially leading to fairer assessments.
-
Scalable Solution for Educational Institutions: This template offers a scalable solution for schools, universities, and online learning platforms to create multiple unique exam papers quickly, supporting large-scale testing and frequent assessment needs.
Technologies
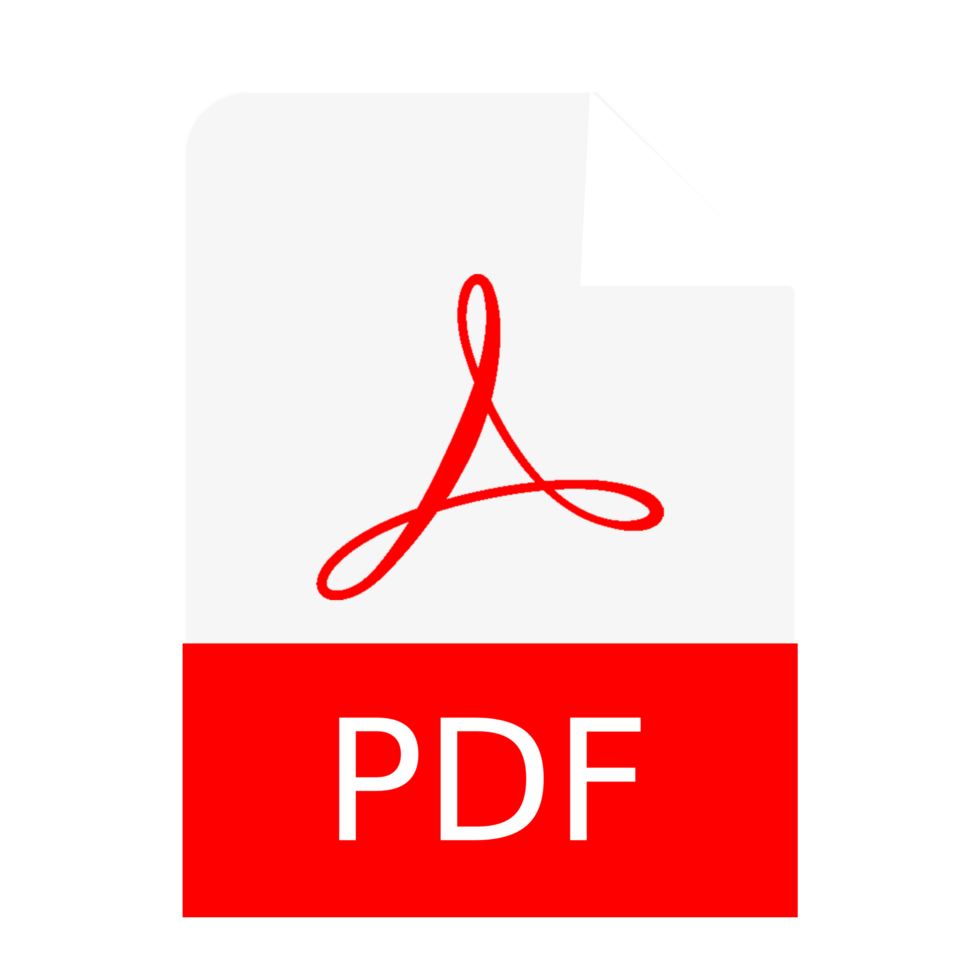