Email Action Point Generator
import logging
from gunicorn.app.base import BaseApplication
from app_init import app
# IMPORT ALL ROUTES
from routes import *
# Setup logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
class StandaloneApplication(BaseApplication):
def __init__(self, app, options=None):
self.application = app
self.options = options or {}
super().__init__()
def load_config(self):
# Apply configuration to Gunicorn
for key, value in self.options.items():
if key in self.cfg.settings and value is not None:
self.cfg.set(key.lower(), value)
Frequently Asked Questions
Workflow Optimization - Streamlines email-to-task conversion, reducing the risk of missed deadlines or forgotten commitments Q2: Which industries would benefit most from using the Email Action Point Generator?
The Email Action Point Generator is particularly valuable for: - Professional Services (consulting, legal, accounting) where client communications drive actions - Project Management firms handling multiple concurrent projects - Sales organizations managing customer relationships and follow-ups - Educational institutions coordinating faculty and administrative tasks - Healthcare administration managing patient follow-ups and administrative tasks
Q3: How does the Email Action Point Generator improve team productivity compared to traditional email management?
A: The system enhances productivity by:
Enabling better workload distribution and management Q4: How can I customize the action point analysis criteria in the Email Action Point Generator?
You can modify the analysis criteria by adjusting the LLM prompt in the analyze_emails_route function. Here's an example:
```python prompt = f"""Analyze these emails and generate action points with deadlines. Focus on emails that require: - Client responses (Priority: High) - Meeting scheduling (Priority: Medium) - Document reviews (Priority: Medium) - Team updates (Priority: Low)
For each email, create an action point with:
Action type (Response/Meeting/Review/Update) Skip emails that are: - Marketing newsletters - System notifications - Social updates """ ``` Q5: How can I implement custom email filtering in the Email Action Point Generator?
You can add custom email filtering by modifying the Gmail API query parameters. Here's an example:
```python def get_filtered_emails(service, days_back, custom_filters): start_date = (datetime.utcnow() - timedelta(days=days_back)).strftime('%Y/%m/%d')
# Build query string with custom filters
query = f'after:{start_date} '
if custom_filters.get('from'):
query += f'from:{custom_filters["from"]} '
if custom_filters.get('subject'):
query += f'subject:{custom_filters["subject"]} '
if custom_filters.get('has_attachment'):
query += 'has:attachment '
results = service.users().messages().list(
userId='me',
q=query
).execute()
return results.get('messages', [])
```
This allows you to filter emails based on sender, subject, attachments, and other Gmail search criteria before processing them through the Email Action Point Generator.
Created: | Last Updated:
Email Action Point Generator Template
This template creates an AI-powered application that analyzes emails to automatically generate actionable tasks with deadlines. It features Gmail integration and secure user authentication.
Getting Started
- Click "Start with this Template" to begin using the template in Lazy Builder
Environment Setup
You'll need to set up Google OAuth2 credentials for Gmail integration:
- Go to the Google Cloud Console
- Create a new project or select an existing one
- Enable the Gmail API for your project
- Configure the OAuth consent screen:
- Set the application type to "Web application"
- Add authorized redirect URIs (you'll get this from Lazy after deployment)
- Create OAuth 2.0 credentials:
- Go to "Credentials" section
- Click "Create Credentials" → "OAuth client ID"
- Download the client credentials
Add these environment secrets in the Lazy Builder Environment Secrets tab:
* GOOGLE_CLIENT_ID
: Your Google OAuth client ID
* GOOGLE_CLIENT_SECRET
: Your Google OAuth client secret
Test the Application
- Click the "Test" button in Lazy Builder
- Lazy will deploy the application and provide you with the application URL
Using the Application
The application provides a web interface with the following features:
- Landing page with feature overview
- Secure Google authentication
- Gmail connection interface
- Action points dashboard showing:
- Email-generated tasks
- Deadlines
- Task status tracking
- Direct links to original emails
To use the application:
- Log in using your Google account
- Connect your Gmail account
- Select the time period for email analysis
- View and manage generated action points
- Mark tasks as complete when finished
The application will analyze your emails using AI to: * Identify important tasks and deadlines * Create actionable items from email content * Provide direct links back to original emails * Track completion status of tasks
The interface is responsive and works on both desktop and mobile devices, with a sidebar navigation for easy access to all features.
Template Benefits
- Automated Task Management from Email
- Automatically converts email communications into structured action items
- Reduces manual effort in task creation and tracking
-
Ensures important email-based tasks don't get overlooked
-
Enhanced Professional Productivity
- AI-powered analysis identifies critical deadlines and priorities
- Centralizes email-based commitments in one dashboard
-
Streamlines workflow by converting email content into actionable tasks
-
Improved Team Accountability
- Clear tracking of email-based commitments and deadlines
- Transparent status updates on action items
-
Helps managers monitor team responsiveness to email requests
-
Time-Saving Email Processing
- Reduces time spent manually reviewing and organizing emails
- Automatically categorizes and prioritizes action items
-
Eliminates the need for separate task management systems
-
Better Client Relationship Management
- Ensures timely follow-up on client communications
- Prevents important client requests from being missed
- Provides clear tracking of client-related commitments and deadlines
Technologies
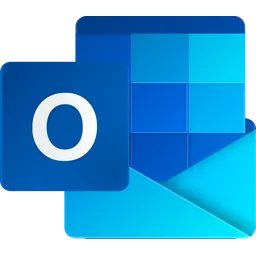

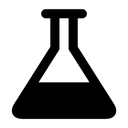
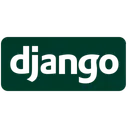
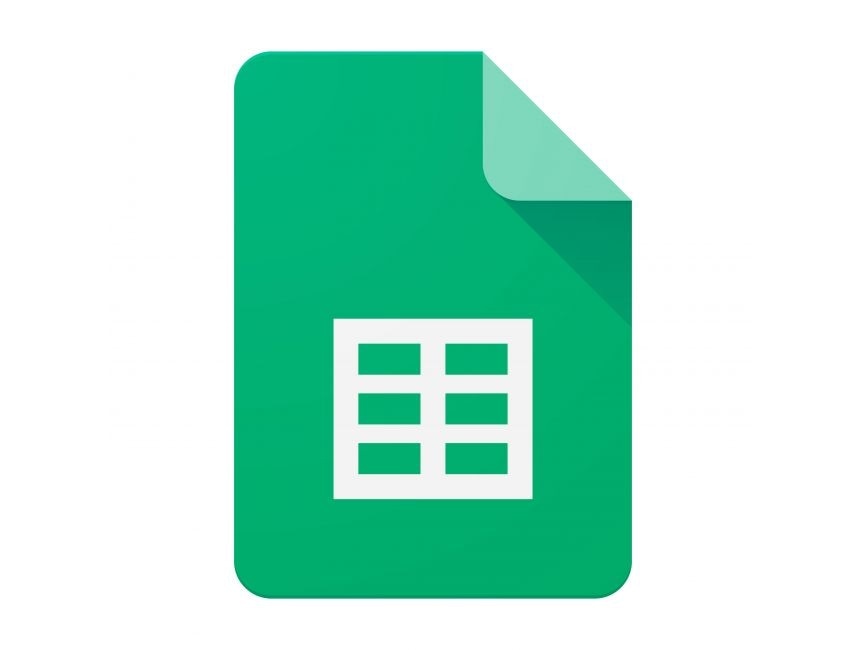
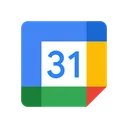
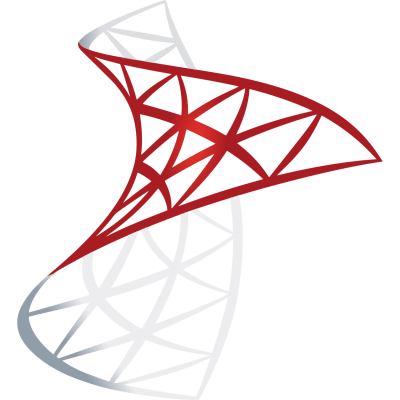