Cinepresa
import logging
from flask import Flask, url_for, request, session
from gunicorn.app.base import BaseApplication
from routes import routes as routes_blueprint
from authentication import auth, auth_required
from models import db, User
from abilities import apply_sqlite_migrations
# Setup logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
def create_app():
app = Flask(__name__, static_folder='static')
app.config['APP_NAME'] = 'Cinepresa AI'
app.secret_key = 'supersecretkey'
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///database.sqlite'
db.init_app(app)
with app.app_context():
apply_sqlite_migrations(db.engine, db.Model, 'migrations')
app.register_blueprint(routes_blueprint)
app.register_blueprint(auth)
Frequently Asked Questions
How can Viral Video Shorts Creator benefit content creators?
Viral Video Shorts Creator offers content creators a powerful tool to repurpose their existing long-form YouTube content into engaging short-form videos. By leveraging AI-powered analysis, the platform identifies the most compelling moments from longer videos, making it easier to create viral-ready shorts for platforms like TikTok, Instagram Reels, and YouTube Shorts. This can help creators expand their reach, increase engagement, and potentially grow their audience across multiple platforms without significantly increasing their workload.
What sets Viral Video Shorts Creator apart from other video editing tools?
Viral Video Shorts Creator distinguishes itself through its AI-driven approach to content analysis and optimization. Unlike traditional video editing tools, it doesn't just cut videos; it intelligently selects the most engaging segments based on AI analysis. Additionally, the platform offers cross-platform publishing capabilities, allowing creators to seamlessly distribute their shorts to multiple social media platforms with a single click. The AI-powered suggestions for maximizing viral potential across different platforms provide creators with valuable insights to optimize their content strategy.
Can Viral Video Shorts Creator be integrated into existing content workflows?
Yes, Viral Video Shorts Creator is designed to seamlessly integrate into existing content workflows. Content creators can easily incorporate it as an additional step in their post-production process. After uploading a long-form video to YouTube, creators can use Viral Video Shorts Creator to automatically generate multiple short-form versions optimized for different platforms. This integration can significantly streamline the content repurposing process, allowing creators to maintain a consistent presence across various social media platforms without dramatically increasing their workload.
How does Viral Video Shorts Creator handle user authentication and social media connections?
Viral Video Shorts Creator uses a flexible authentication system that supports multiple social media platforms. The authentication process is handled in the authentication.py
file, which includes functions for connecting and disconnecting various social media accounts. Here's an example of how the platform updates user tokens after authentication:
```python def update_user_social_tokens(): if 'user' in session: email = session['user'].get('user_email') if email: user = User.query.filter_by(email=email).first() if not user: user = User(email=email) db.session.add(user)
auth_provider = session['user'].get('auth_provider')
if auth_provider == 'google':
user.youtube_token = session['user'].get('access_token')
user.youtube_channel_id = session['user'].get('youtube_channel_id')
elif auth_provider == 'instagram':
user.instagram_token = session['user'].get('access_token')
user.instagram_username = session['user'].get('instagram_username')
elif auth_provider == 'tiktok':
user.tiktok_token = session['user'].get('access_token')
user.tiktok_username = session['user'].get('tiktok_username')
db.session.commit()
```
This function updates the user's social media tokens in the database based on the authentication response, allowing the platform to maintain connections with various social media accounts.
How does Viral Video Shorts Creator ensure the security of user data and social media tokens?
Viral Video Shorts Creator prioritizes user data security through several measures. First, it uses SQLAlchemy ORM to interact with the database, which helps prevent SQL injection attacks. Sensitive data like social media tokens are stored in the database, not in client-side storage. The platform also implements secure session management. Here's an example of how the user model is structured to store sensitive information:
python
class User(db.Model):
id = db.Column(db.Integer, primary_key=True)
email = db.Column(db.String(150), unique=True, nullable=False)
profile_picture = db.Column(db.String(255))
instagram_token = db.Column(db.String(500))
instagram_username = db.Column(db.String(100))
tiktok_token = db.Column(db.String(500))
tiktok_username = db.Column(db.String(100))
youtube_token = db.Column(db.String(500))
youtube_channel_id = db.Column(db.String(100))
This structure ensures that sensitive tokens are stored securely in the database. Additionally, the platform uses HTTPS for all communications and implements proper authentication checks before allowing access to sensitive data or operations. It's important to note that in a production environment, additional security measures such as token encryption at rest would be implemented.
Created: | Last Updated:
Here's a step-by-step guide for using the Viral Video Shorts Creator template:
Introduction
The Viral Video Shorts Creator is a web application that allows you to transform long YouTube videos into engaging short-form content. This template provides features such as customizable subtitles, optional background music, and AI-driven content analysis to help create viral-ready shorts for platforms like TikTok, Instagram Reels, and YouTube Shorts.
Getting Started
To begin using this template:
- Click the "Start with this Template" button in the Lazy Builder interface.
Test the Application
After starting with the template:
- Click the "Test" button in the Lazy Builder interface.
- This will initiate the deployment of the app and launch the Lazy CLI.
Using the Application
Once the application is deployed:
- The Lazy CLI will provide you with a dedicated server link to access the web application.
- Open this link in your web browser to access the Viral Video Shorts Creator interface.
Creating Video Shorts
To create video shorts using the application:
- On the home page, you'll see a form to enter a YouTube video URL.
- Paste the full URL of the YouTube video you want to convert into shorts.
- Click the "Process Video" button.
Note: The actual video processing feature is not yet implemented in this template. Currently, it will show an alert saying "Video processing feature coming soon!"
Connecting Social Media Accounts
The application allows you to connect various social media accounts:
- Navigate to the profile page using the sidebar menu.
- You'll see options to connect Instagram, TikTok, and YouTube accounts.
- Click on the respective "Connect" buttons for each platform you want to link.
Note: The actual OAuth flows for connecting these accounts are not yet implemented in this template. The UI is prepared for future functionality.
Logging Out
To log out of the application:
- Click the "Logout" button in the sidebar menu or at the top-right corner of the screen.
- Confirm the logout action when prompted.
Future Enhancements
This template provides a foundation for a video shorts creation tool. To fully implement the functionality, you would need to:
- Implement the YouTube video processing logic.
- Set up OAuth flows for Instagram, TikTok, and YouTube.
- Develop AI-driven content analysis for identifying engaging moments in videos.
- Implement features for adding customizable subtitles and background music.
Remember, this template is a starting point and would require further development to achieve full functionality as a viral video shorts creator.
Template Benefits
-
Content Repurposing: This template enables content creators to efficiently repurpose long-form YouTube videos into short-form content, maximizing the value of existing material and expanding reach across multiple platforms.
-
AI-Powered Optimization: By leveraging AI analysis to identify engaging moments and optimize for viral potential, the template helps creators increase their chances of producing successful, attention-grabbing short-form content.
-
Cross-Platform Publishing: The ability to seamlessly publish shorts to YouTube, Instagram, and TikTok from a single interface streamlines the content distribution process, saving time and effort for creators and marketers.
-
User Engagement: Customizable subtitles and background music options allow creators to enhance their shorts, potentially increasing viewer engagement and retention across different social media platforms.
-
Analytics and Insights: The template's integration with multiple social media platforms provides a centralized location for creators to track performance and gather insights, enabling data-driven decision-making for future content strategies.
Technologies
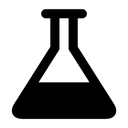
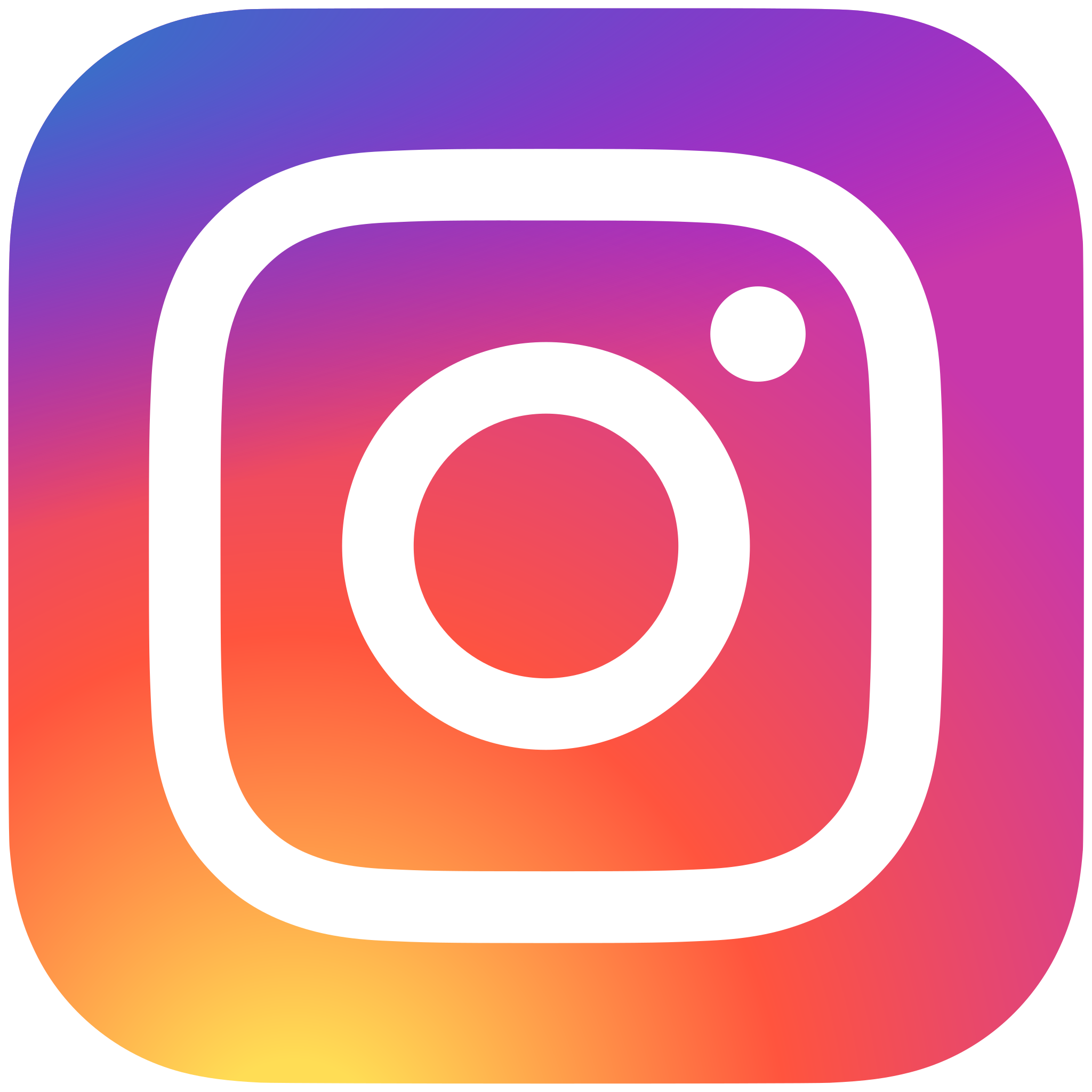


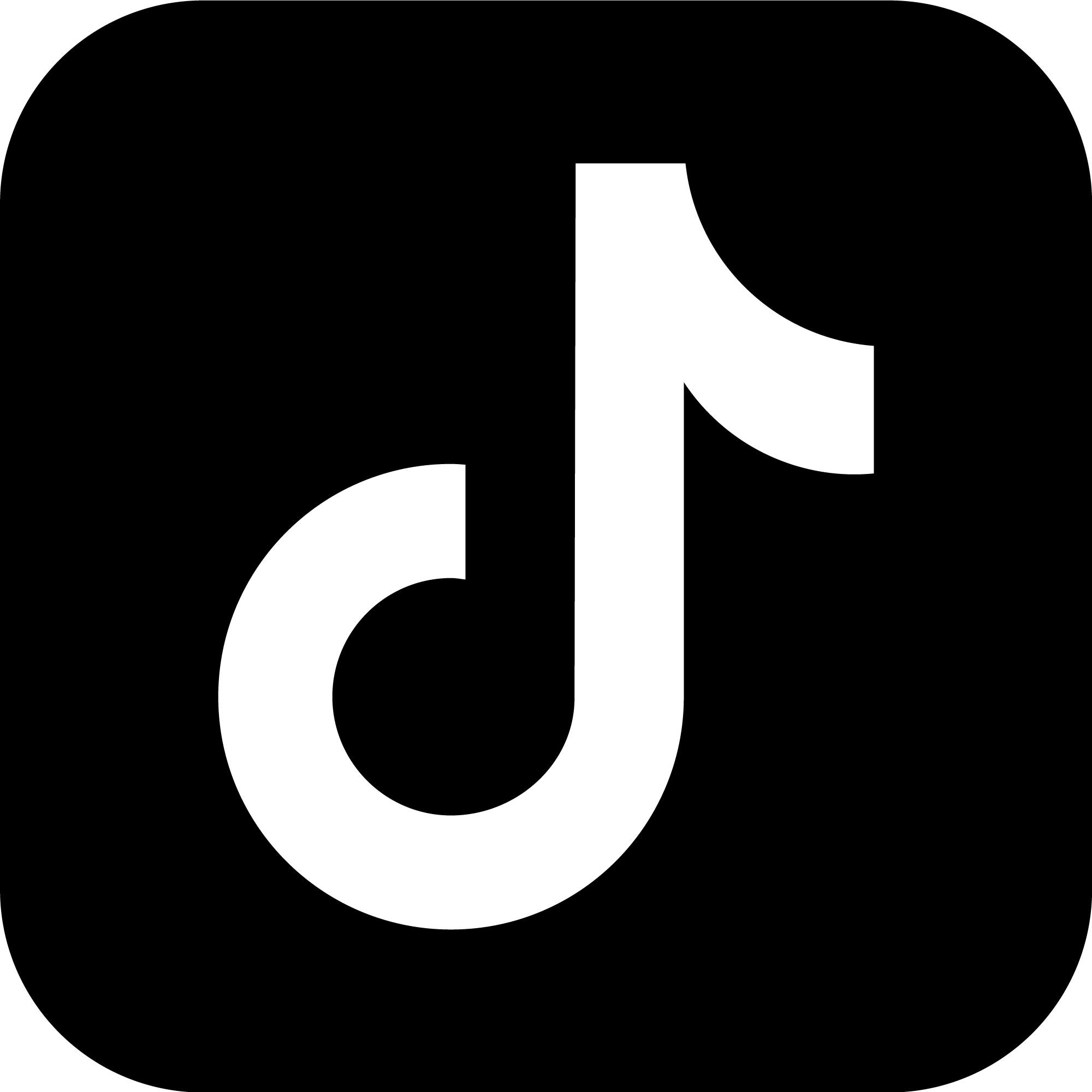