AI Scraper Selenium App
import logging
from gunicorn.app.base import BaseApplication
from app_init import create_initialized_flask_app
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
class StandaloneApplication(BaseApplication):
def __init__(self, app, options=None):
self.application = app
self.options = options or {}
super().__init__()
def load_config(self):
# Apply configuration to Gunicorn
for key, value in self.options.items():
if key in self.cfg.settings and value is not None:
self.cfg.set(key.lower(), value)
def load(self):
return self.application
if __name__ == "__main__":
options = {
Frequently Asked Questions
What are some business applications for the AI Scraper Selenium App?
The AI Scraper Selenium App has numerous business applications, including: - Competitive analysis: Gathering pricing and product information from competitor websites - Market research: Collecting customer reviews and sentiment data from various online sources - Lead generation: Extracting contact information from business directories or industry-specific websites - Content aggregation: Compiling news articles or blog posts from multiple sources for content curation - Price monitoring: Tracking price changes of products across different e-commerce platforms
How can the AI Scraper Selenium App improve efficiency in data collection processes?
The AI Scraper Selenium App significantly improves efficiency in data collection by: - Automating the process of navigating websites and extracting information - Handling dynamic content and JavaScript-rendered pages that traditional web scraping tools might miss - Integrating AI capabilities to interpret and analyze the collected data - Providing a user-friendly interface for non-technical users to input URLs and questions - Scaling data collection efforts without the need for manual intervention
What industries could benefit most from using the AI Scraper Selenium App?
Several industries can benefit from the AI Scraper Selenium App, including: - E-commerce: For price comparison and product research - Finance: To gather real-time market data and financial news - Real estate: To collect property listings and market trends - Travel and hospitality: For monitoring competitor pricing and availability - Healthcare: To aggregate medical research and clinical trial information
How can I extend the SeleniumUtility class in the AI Scraper Selenium App to perform more complex actions on a webpage?
You can extend the SeleniumUtility class by adding new methods for specific actions. For example, to click a button and wait for a new page to load:
```python from selenium.webdriver.common.by import By from selenium.webdriver.support.ui import WebDriverWait from selenium.webdriver.support import expected_conditions as EC
class SeleniumUtility: # ... existing code ...
def click_button_and_wait(self, button_id, timeout=10):
try:
button = WebDriverWait(self.driver, timeout).until(
EC.element_to_be_clickable((By.ID, button_id))
)
button.click()
WebDriverWait(self.driver, timeout).until(
EC.staleness_of(button)
)
logger.info(f"Clicked button {button_id} and waited for page load")
except Exception as e:
logger.error(f"Failed to click button {button_id}: {e}")
```
This new method can be used in the AI Scraper Selenium App to interact with web pages that require button clicks before accessing the desired content.
How can I modify the AI Scraper Selenium App to save the scraped data to a database instead of just displaying it?
You can modify the process_page_info
function in the routes.py
file to save the data to a database. Here's an example using SQLAlchemy:
```python from models import ScrapedData # Assume you've defined this model
@routes.route('/process-page-info', methods=['POST']) def process_page_info(): url = request.form.get("url") question = request.form.get("question") selenium_util = SeleniumUtility() page_text = selenium_util.get_page_text(url)
prompt = f"Answer the following question based on the provided page text:\n\nQuestion: {question}\n\nPage Text: {page_text}"
response = llm(prompt=prompt, response_schema={"type": "object", "properties": {"answer": {"type": "string"}}}, model="gpt-4o", temperature=0)
# Save to database
new_data = ScrapedData(url=url, question=question, answer=response['answer'])
db.session.add(new_data)
db.session.commit()
return render_template("page_information.html", response=response)
```
This modification allows the AI Scraper Selenium App to persistently store the scraped data and AI-generated answers for future reference or analysis.
Created: | Last Updated:
Here's a step-by-step guide for using the AI Scraper Selenium App template:
Introduction
The AI Scraper Selenium App is a powerful tool designed to gather data from websites, even those that might block simpler scraping methods. This template uses Selenium for web scraping and integrates with an AI model to process and analyze the scraped content.
Getting Started
- Click "Start with this Template" to begin using the AI Scraper Selenium App template in the Lazy Builder interface.
Test the App
-
Press the "Test" button in the Lazy Builder interface to deploy and launch the application.
-
Once the app is deployed, you will receive a dedicated server link to access the web interface.
Using the App
-
Open the provided server link in your web browser. You will see a form with two input fields:
-
URL: Enter the website URL you want to scrape.
-
Question: Enter a question about the content you want to analyze.
-
Fill in both fields and click the "Submit" button.
-
The app will use Selenium to scrape the content from the provided URL and then use an AI model (GPT-4) to analyze the content and answer your question.
-
After processing, you will see a results page displaying the AI-generated answer to your question based on the scraped content.
How It Works
- The app uses Selenium to navigate to the specified URL and extract the page content, even from websites that might block simpler scraping methods.
- The extracted content is then sent to an AI model along with your question.
- The AI model analyzes the content and generates an answer to your question.
- The results are displayed on a web page for easy viewing.
This template provides a robust starting point for building web scraping applications that require more advanced techniques and AI-powered analysis. You can further customize the app to suit your specific needs, such as adding more detailed scraping logic or expanding the AI analysis capabilities.
Here are 5 key business benefits for this AI Scraper Selenium App template:
Template Benefits
-
Automated Web Data Extraction: This template enables businesses to automatically extract data from websites, even those with dynamic content or JavaScript-heavy interfaces, saving time and reducing manual effort in data collection processes.
-
Flexible Information Retrieval: By combining Selenium for web scraping with AI-powered natural language processing, businesses can ask specific questions about web content and receive targeted answers, enhancing decision-making capabilities.
-
Scalable Web Monitoring: The template provides a foundation for building scalable web monitoring solutions, allowing businesses to track changes on multiple websites simultaneously and stay updated on competitor activities, market trends, or regulatory changes.
-
Customizable Data Processing: With the integration of AI capabilities, businesses can tailor the data extraction and processing to their specific needs, enabling more sophisticated analysis and insights from web-based information.
-
Robust and Maintainable Architecture: The template's well-structured codebase, including database migrations and modular design, ensures that the application is easy to maintain, extend, and scale as business needs evolve over time.
Technologies
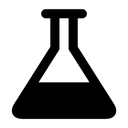
