YouTube Video Downloader
import logging
from gunicorn.app.base import BaseApplication
from app_init import create_initialized_flask_app
# Flask app creation should be done by create_initialized_flask_app to avoid circular dependency problems.
app = create_initialized_flask_app()
# Setup logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
class StandaloneApplication(BaseApplication):
def __init__(self, app, options=None):
self.application = app
self.options = options or {}
super().__init__()
def load_config(self):
# Apply configuration to Gunicorn
for key, value in self.options.items():
if key in self.cfg.settings and value is not None:
self.cfg.set(key.lower(), value)
def load(self):
Frequently Asked Questions
How can this YouTube Video Downloader template be monetized as a business?
The YouTube Video Downloader template can be monetized in several ways: - Implement a freemium model where basic downloads are free, but high-quality or batch downloads require a subscription. - Integrate ads into the application, displaying them while users wait for their downloads. - Offer a white-label version of the YouTube Video Downloader for businesses to rebrand and use internally. - Provide API access for developers to integrate the downloading functionality into their own applications for a fee.
What are some potential legal considerations when using this YouTube Video Downloader template?
When using the YouTube Video Downloader template, it's crucial to consider: - YouTube's Terms of Service, which prohibit unauthorized downloading of content. - Copyright laws, as downloading videos may infringe on content creators' rights. - Potential liability for users' actions if the tool is misused. - Compliance with data protection regulations if user data is collected.
It's recommended to consult with a legal professional to ensure the application complies with all relevant laws and regulations.
How can the YouTube Video Downloader template be adapted for educational purposes?
The YouTube Video Downloader template can be adapted for educational use by: - Implementing content filtering to allow only educational videos to be downloaded. - Adding features for teachers to curate and share video playlists with students. - Integrating with learning management systems for seamless use in online courses. - Including tools for students to take notes or create quizzes based on downloaded videos.
These adaptations can transform the template into a valuable educational resource while maintaining its core functionality.
How can I add a progress bar to show the download status in the YouTube Video Downloader template?
To add a progress bar, you can use JavaScript to update the UI based on the download progress. Here's a basic example of how you might implement this:
``javascript
// Add this to home.js
function updateProgressBar(progress) {
const progressBar = document.getElementById('download-progress');
progressBar.style.width =
${progress}%;
progressBar.textContent =
${progress}%`;
}
// In your download function fetch('/download', { method: 'POST', body: JSON.stringify({ url: videoUrl }) }) .then(response => { const reader = response.body.getReader(); const contentLength = +response.headers.get('Content-Length'); let receivedLength = 0;
return new ReadableStream({
start(controller) {
function push() {
reader.read().then(({ done, value }) => {
if (done) {
controller.close();
return;
}
receivedLength += value.length;
const progress = Math.round((receivedLength / contentLength) * 100);
updateProgressBar(progress);
controller.enqueue(value);
push();
});
}
push();
}
});
})
.then(stream => new Response(stream))
.then(response => response.blob())
.then(blob => {
// Handle the completed download
});
```
This code assumes you've added a progress bar element to your HTML with the id 'download-progress'.
How can I implement video format selection in the YouTube Video Downloader template?
To implement video format selection, you'll need to modify both the frontend and backend of the YouTube Video Downloader template. Here's a basic example:
In the HTML (home.html):
html
<select id="format-select">
<option value="mp4">MP4</option>
<option value="webm">WebM</option>
<option value="audio">Audio Only (MP3)</option>
</select>
In the JavaScript (home.js): ```javascript const formatSelect = document.getElementById('format-select');
function downloadVideo() { const videoUrl = document.getElementById('video-url').value; const format = formatSelect.value;
fetch('/download', {
method: 'POST',
headers: { 'Content-Type': 'application/json' },
body: JSON.stringify({ url: videoUrl, format: format })
})
.then(response => response.blob())
.then(blob => {
// Handle the download
});
} ```
In the Python backend (routes.py): ```python from flask import request, send_file import yt_dlp
@app.route("/download", methods=['POST']) def download_video(): data = request.json url = data['url'] format = data['format']
ydl_opts = {
'format': 'bestaudio/best' if format == 'audio' else f'best[ext={format}]',
'postprocessors': [{
'key': 'FFmpegExtractAudio',
'preferredcodec': 'mp3',
'preferredquality': '192',
}] if format == 'audio' else [],
}
with yt_dlp.YoutubeDL(ydl_opts) as ydl:
info = ydl.extract_info(url, download=False)
filename = ydl.prepare_filename(info)
ydl.download([url])
return send_file(filename, as_attachment=True)
```
This implementation allows users to select their preferred format before downloading, enhancing the functionality of the YouTube Video Downloader template.
Created: | Last Updated:
Here's a step-by-step guide for using the YouTube Video Downloader template:
Introduction
This template provides a web application for downloading YouTube videos by submitting video URLs. It offers a simple interface for users to input YouTube video links and download the corresponding videos.
Getting Started
To begin using this template:
- Click the "Start with this Template" button in the Lazy Builder interface.
Test the Application
Once you've started with the template:
- Click the "Test" button in the Lazy Builder interface.
- The application will begin deployment, and the Lazy CLI will appear.
Using the YouTube Video Downloader
After the application has been deployed:
- Lazy will provide you with a dedicated server link to access the web application.
- Open the provided link in your web browser.
- You'll see a simple interface with a text input field and a "Download" button.
- Enter a valid YouTube video URL into the input field.
- Click the "Download" button.
- The application will process your request and provide a download link for the video.
Important Notes
- Ensure that you have the right to download and use the YouTube videos in compliance with YouTube's terms of service and copyright laws.
- The application may have limitations on video quality or file size, depending on the implementation details.
- Download speeds may vary based on your internet connection and the size of the video.
By following these steps, you'll be able to use the YouTube Video Downloader template to create a functional web application for downloading YouTube videos. Remember to use this tool responsibly and in accordance with applicable laws and regulations.
Here are 5 key business benefits for this template:
Template Benefits
-
Responsive Design: The template includes both mobile and desktop layouts, ensuring a seamless user experience across devices. This can lead to increased user engagement and retention.
-
Scalable Architecture: The use of Flask with Gunicorn and SQLAlchemy provides a solid foundation for building scalable web applications. This allows businesses to easily expand their online presence as they grow.
-
Easy Customization: The modular structure of the template, with separate files for HTML, CSS, and JavaScript, makes it simple to customize the look and functionality of the online store. This allows businesses to quickly adapt the template to their specific brand and needs.
-
SEO-Friendly Structure: The semantic HTML structure and use of appropriate tags can help improve search engine optimization, potentially leading to better visibility in search results and increased organic traffic.
-
Built-in Database Management: The inclusion of a database setup with migration capabilities allows for easy management of product data, user information, and other critical business data. This can streamline operations and improve data integrity.
Technologies
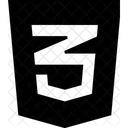
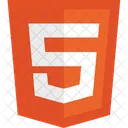