WhatsApp Business Humanized Chatbot for Customer Support
import logging
from gunicorn.app.base import BaseApplication
from app_init import create_initialized_flask_app
# Flask app creation should be done by create_initialized_flask_app to avoid circular dependency problems.
app = create_initialized_flask_app()
# Setup logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
class StandaloneApplication(BaseApplication):
def __init__(self, app, options=None):
self.application = app
self.options = options or {}
super().__init__()
def load_config(self):
# Apply configuration to Gunicorn
for key, value in self.options.items():
if key in self.cfg.settings and value is not None:
self.cfg.set(key.lower(), value)
def load(self):
Frequently Asked Questions
How can the WhatsApp Business Humanized Chatbot benefit my business?
The WhatsApp Business Humanized Chatbot can significantly enhance your customer engagement and support. It provides automated responses that feel more natural and human-like, improving the overall customer experience. This can lead to increased customer satisfaction, faster response times, and the ability to handle a higher volume of inquiries without increasing staff. The chatbot can be particularly useful for handling common questions, scheduling appointments, or providing product information, freeing up your human staff to focus on more complex issues.
What types of businesses would benefit most from implementing this WhatsApp Business Humanized Chatbot?
This chatbot solution is versatile and can benefit a wide range of businesses. It's particularly valuable for: - Retail businesses looking to provide quick product information and support - Service-based businesses that need to manage appointments and inquiries - E-commerce companies aiming to improve customer service and reduce cart abandonment - Small businesses looking to scale their customer support without hiring additional staff - Any business that receives a high volume of similar inquiries through WhatsApp
How customizable is the WhatsApp Business Humanized Chatbot for my specific business needs?
The WhatsApp Business Humanized Chatbot is highly customizable. You can tailor the chatbot's responses, conversation flow, and functionality to match your specific business needs and brand voice. The template provides a solid foundation that can be extended with additional features, integrations with your existing systems, and custom logic to handle various scenarios unique to your business.
How can I extend the chatbot's functionality to handle more complex interactions?
You can extend the chatbot's functionality by modifying the receive_message
route in the routes.py
file. Here's an example of how you might implement more complex logic:
```python from flask import request, jsonify from models import db, Message
@app.route("/receive_message", methods=["POST"]) def receive_message(): data = request.json user_message = data.get('message')
# Implement your chatbot logic here
if "product" in user_message.lower():
response = "We offer a wide range of products. Can you specify what you're looking for?"
elif "appointment" in user_message.lower():
response = "Certainly! I can help you schedule an appointment. What date works best for you?"
else:
response = "Thank you for your message. How can I assist you today?"
# Save the interaction to the database
new_message = Message(content=user_message, response=response)
db.session.add(new_message)
db.session.commit()
return jsonify({"response": response})
```
This example shows how you can add conditional logic to handle different types of inquiries and save interactions to the database.
How do I set up the database for the WhatsApp Business Humanized Chatbot?
The template uses SQLAlchemy with SQLite for simplicity. To set up the database, you'll need to initialize it and create the tables. Here's how you can do this:
Created: | Last Updated:
Introduction to the Template
The WhatsApp Business Humanized Chatbot template provides an automated chatbot for WhatsApp Business with humanized response logic to engage customers effectively. This template leverages Flask for the backend, SQLAlchemy for database management, and Tailwind CSS for styling.
Clicking Start with this Template
To get started with the template, click Start with this Template in the Lazy Builder interface.
Test: Pressing the Test Button
After starting with the template, press the Test button. This will deploy the app and launch the Lazy CLI. Follow any prompts that appear in the CLI.
Entering Input
The template does not require any user input through the CLI.
Using the App
The app provides a simple web interface with the following features:
- Home Page: Displays a basic home page.
- Receive Message Endpoint: An endpoint to receive messages via POST requests.
Home Page
The home page is rendered using the home.html
template. It includes a header and a container for content. The header has a navigation bar that adapts to both desktop and mobile views.
Receive Message Endpoint
The /receive_message
endpoint is designed to handle POST requests. This is where the chatbot logic will be implemented to process incoming messages.
Integrating the App
To integrate the app with WhatsApp Business, follow these steps:
- Set Up WhatsApp Business API:
- Register for the WhatsApp Business API.
-
Obtain the necessary API keys and credentials.
-
Configure Webhook:
- Set up a webhook in the WhatsApp Business API to point to the
/receive_message
endpoint of your deployed app. -
Ensure the webhook URL is accessible from the internet.
-
Sample Request and Response:
Sample Request:
json
{
"message": "Hello, I need help with my order."
}
Sample Response:
json
{
"response": "Hello! Thanks for reaching out. We'll get back to you shortly."
}
External Integration Steps
- Register for WhatsApp Business API:
-
Visit the WhatsApp Business API documentation to register and obtain API credentials.
-
Set Up Webhook:
- In the WhatsApp Business API settings, configure the webhook URL to point to your app's
/receive_message
endpoint. -
Example:
https://your-app-url/receive_message
-
Test the Integration:
- Send a test message to your WhatsApp Business number.
- Verify that the message is received and processed by your app.
By following these steps, you will have a fully functional WhatsApp Business Humanized Chatbot integrated with your WhatsApp Business account.
Template Benefits
-
Enhanced Customer Engagement: This WhatsApp Business chatbot template enables businesses to provide instant, personalized responses to customer inquiries 24/7, significantly improving customer engagement and satisfaction.
-
Scalable Customer Support: By automating initial customer interactions, businesses can handle a higher volume of inquiries without increasing staff, allowing human agents to focus on more complex issues.
-
Cost-Effective Operations: The chatbot reduces the need for a large customer service team, lowering operational costs while maintaining high-quality customer support.
-
Data-Driven Insights: The template's structure allows for easy integration of analytics, providing valuable insights into customer behavior, common inquiries, and areas for business improvement.
-
Seamless Multi-Platform Integration: Built with Flask and modern web technologies, this template can be easily adapted to work across various platforms beyond WhatsApp, offering businesses flexibility in their communication channels.
Technologies
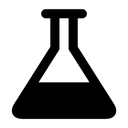