by Lazy Sloth
Discord Multi Channel Message Bot
import os
import discord
from discord.ext import commands
from discord.ext.commands import has_permissions
intents = discord.Intents.all()
intents.members = True
bot = commands.Bot(command_prefix='!', intents=intents)
# List to store multiple channel IDs
multi_channel_ids = []
@bot.command()
@has_permissions(administrator=True)
async def add_channel(ctx, channel: discord.TextChannel):
if channel.id not in multi_channel_ids:
multi_channel_ids.append(channel.id)
await ctx.send(f'Channel {channel.mention} added to the multiple msg channel list.')
@bot.command()
@has_permissions(administrator=True)
async def remove_channel(ctx, channel: discord.TextChannel):
if channel.id in multi_channel_ids:
Discord Multi Channel Message Bot
Created: | Last Updated:
Introduction to the Discord Multi Channel Message Bot Template
Welcome to the Discord Multi Channel Message Bot template! This template is designed to help you create a Discord bot that can manage a list of channels and send messages to all of them with a single command. This is particularly useful for server administrators who need to broadcast announcements or updates across multiple channels efficiently.
Getting Started with the Template
To begin using this template, simply click on the "Start with this Template" button. This will set up the template in your Lazy builder interface, pre-populating the code so you can start customizing and deploying your bot without any hassle.
Initial Setup
Before you can test and use your bot, you need to set up an environment secret for your Discord bot token. This token allows your bot to interact with the Discord API.
- Go to the Discord Developer Portal and create a new application.
- Under the "Bot" tab, click "Add Bot" and confirm the creation.
- Copy the token provided for your new bot.
- In the Lazy Builder interface, navigate to the Environment Secrets tab.
- Create a new secret with the key `DISCORD_BOT_TOKEN` and paste your bot token as the value.
Test: Pressing the Test Button
Once you have set up your environment secret, you can press the "Test" button. This will deploy your application and launch the Lazy CLI. The CLI will not prompt for any user input at this stage, as the bot uses commands within Discord to operate.
Using the App
After testing and deploying your bot, you can invite it to your Discord server using the OAuth2 URL generated in the Discord Developer Portal. Once the bot is in your server, you can use the following commands:
!add_channel #channel-name
- Adds a channel to the list of channels where messages will be sent.!remove_channel #channel-name
- Removes a channel from the list.!send_message Your message here
- Sends a message to all channels in the list after confirmation.
Note: These commands require administrator permissions to use.
Integrating the App
There are no additional external integration steps required for this template. Once your bot is added to your Discord server and you have set up the environment secret with your bot token, you are ready to use the bot's commands to manage your message distribution across multiple channels.
If you need further assistance or have any questions, refer to the discord.py documentation for more details on how to customize and enhance your bot's capabilities.
Technologies
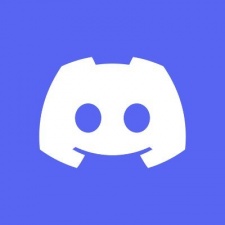
