by yahya
Discord Server Stats Bot
import os
import discord
from discord.ext import commands
from datetime import datetime, timedelta
from collections import Counter
import pytz
intents = discord.Intents.all()
bot = commands.Bot(command_prefix='!', intents=intents)
@bot.command()
async def serverstats(ctx):
# Total number of members in the server.
total_members = ctx.guild.member_count
progress_message = await ctx.reply('Gathering stats...')
await progress_message.edit(content='Gathered total members...')
# Number of online members.
online_members = len([member for member in ctx.guild.members if member.status == discord.Status.online])
await progress_message.edit(content='Gathered online members...')
# Number of offline members.
offline_members = len([member for member in ctx.guild.members if member.status == discord.Status.offline])
Frequently Asked Questions
How can this Discord Server Stats Bot benefit my community or business?
The Discord Server Stats Bot can provide valuable insights into your community's engagement and growth. By offering real-time statistics on member count, activity levels, and channel usage, it helps community managers and business owners make data-driven decisions. For example, you can track the effectiveness of marketing campaigns by monitoring the number of new members joining in the last 24 hours, or assess the popularity of different channels to optimize content strategy.
Can the Discord Server Stats Bot be customized for specific business needs?
Yes, the Discord Server Stats Bot is highly customizable. While the template provides a solid foundation with commands like !serverstats
and !channelstats
, you can easily extend its functionality to suit your specific business needs. For instance, you could add commands to track engagement with product announcements, monitor support ticket trends, or analyze user retention rates over time.
How does the Discord Server Stats Bot handle data privacy and security?
The Discord Server Stats Bot is designed with privacy and security in mind. It only accesses the data it needs to generate statistics and doesn't store any personal information. However, it's important to note that the bot requires certain permissions to function properly. Always ensure that you're complying with data protection regulations and Discord's terms of service when using the bot in your server.
How can I add custom statistics to the Discord Server Stats Bot?
You can easily add custom statistics by modifying the serverstats
command in the main.py
file. For example, if you want to add a statistic for the number of members with a specific role, you could add the following code:
```python @bot.command() async def serverstats(ctx): # ... existing code ...
# Number of members with a specific role
role_name = "VIP"
role = discord.utils.get(ctx.guild.roles, name=role_name)
vip_members = len(role.members) if role else 0
stats_message += f"\nVIP Members: {vip_members}"
await progress_message.edit(content=stats_message)
```
This would add a count of members with the "VIP" role to your server statistics.
How can I make the Discord Server Stats Bot run commands automatically at set intervals?
To run commands automatically, you can use Discord.py's tasks feature. Here's an example of how you could modify the Discord Server Stats Bot to post server stats every 24 hours:
```python from discord.ext import tasks
# ... existing imports and bot setup ...
@tasks.loop(hours=24) async def daily_stats(): channel = bot.get_channel(STATS_CHANNEL_ID) # Replace with your channel ID ctx = await bot.get_context(await channel.fetch_message(channel.last_message_id)) await serverstats(ctx)
@bot.event async def on_ready(): daily_stats.start()
# ... rest of your bot code ... ```
This would call the serverstats
command every 24 hours and post the results in a specified channel. Remember to replace STATS_CHANNEL_ID
with the ID of the channel where you want the stats to be posted.
Created: | Last Updated:
Here's a step-by-step guide for using the Discord Server Stats Bot template:
Introduction
This template provides a Discord bot that can generate statistics for your Discord server. The bot offers two main commands: !serverstats
for overall server statistics and !channelstats
for specific channel statistics.
Getting Started
- Click "Start with this Template" to begin using this template in the Lazy Builder interface.
Initial Setup
- Set up the Discord Bot Token:
- Go to the Discord Developer Portal (https://discord.com/developers/applications)
- Create a new application or select an existing one
- Navigate to the "Bot" section
- Create a bot if you haven't already
- Copy the bot token
- In the Lazy Builder, go to the Environment Secrets tab
-
Add a new secret with the name 'DISCORD_BOT_TOKEN' and paste your bot token as the value
-
Invite the bot to your server:
- In the Discord Developer Portal, go to the "OAuth2" section
- In the "Scopes" section, select "bot"
- In the "Bot Permissions" section, select the following permissions:
- Read Messages/View Channels
- Send Messages
- Read Message History
- Copy the generated OAuth2 URL
- Open this URL in a new browser tab and select your server to invite the bot
Test
- Click the "Test" button in the Lazy Builder to deploy and run the bot.
Using the Bot
-
Once the bot is running, you can use the following commands in your Discord server:
-
!serverstats
: This command will provide overall statistics for your server, including:- Total members
- Online members
- Offline members
- Number of bots
- Number of text and voice channels
- Number of roles
- Server creation date
- Members joined in the last 24 hours
-
!channelstats [channel_name]
: This command will provide statistics for a specific text channel, including:- Number of messages in the last 24 hours
- Most active members
- Most used emoji
Replace [channel_name]
with the name of the channel you want to analyze.
Integrating the Bot
- The bot is now ready to use in your Discord server. You don't need to perform any additional integration steps. Simply use the commands in your server's text channels to get the statistics you need.
Remember that the bot needs to have the necessary permissions in your server to read messages and access channel histories for accurate statistics.
Here are 5 key business benefits for this Discord Server Stats Bot template:
Template Benefits
-
Enhanced Community Insights: Provides valuable data on server activity and member engagement, allowing businesses to better understand their Discord community dynamics and make informed decisions.
-
Improved Moderation and Management: Offers quick access to server statistics, helping moderators and administrators efficiently monitor growth, identify trends, and manage resources accordingly.
-
Data-Driven Marketing Strategies: Enables businesses to track user engagement and channel popularity, informing targeted marketing efforts and content strategies within the Discord platform.
-
Real-Time Performance Metrics: Delivers up-to-date statistics on server activity, allowing businesses to measure the success of events, campaigns, or community initiatives in real-time.
-
Customizable Analytics Tool: Serves as a foundation for building more complex analytics features, allowing businesses to tailor the bot to their specific needs and extract the most relevant data for their operations.
Technologies
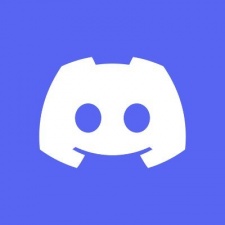