Website Stats App
from selenium import webdriver
from selenium.webdriver.chrome.options import Options
import time
import requests
import os
import asyncio
from discord_webhook import DiscordWebhook
# Obtain the Discord webhook URL from environment variables
discord_webhook_url = os.environ.get('DISCORD_WEBHOOK_URL', None)
# Obtain the website URL from environment variables
website_link = os.environ.get('WEBSITE_URL', None)
def website_stats():
# Check if the URL is correct
if not website_link.startswith("http://") and not website_link.startswith("https://"):
print("The URL is not correct. Please enter a valid URL.")
return
options = Options()
options.add_argument("--no-sandbox")
options.add_argument("--headless")
Frequently Asked Questions
How can the Website Stats App benefit my business?
The Website Stats App can provide valuable insights into your website's performance and security. By regularly monitoring load times, status codes, and security protocols, you can ensure your website is running optimally for your customers. This can lead to improved user experience, better search engine rankings, and potentially increased conversions. The automatic Discord updates every 7 hours also allow you to stay informed about your website's performance without constant manual checking.
Can I use the Website Stats App to monitor multiple websites?
Yes, you can use the Website Stats App to monitor multiple websites. However, you would need to set up separate instances of the app for each website you want to monitor. Each instance would require its own set of environment variables (DISCORD_WEBHOOK_URL and WEBSITE_URL). This allows you to track and compare the performance of different websites in your portfolio or monitor your competitors' websites.
How can I customize the frequency of updates from the Website Stats App?
The Website Stats App is designed to post updates every 7 hours by default. However, you can easily customize this frequency by modifying the sleep time in the post_updates()
function. For example, if you want to receive updates every 4 hours instead of 7, you would change the following line:
python
await asyncio.sleep(25200) # 7 hours
to:
python
await asyncio.sleep(14400) # 4 hours
Remember that more frequent updates will increase the load on your server and the website being monitored, so choose a frequency that balances your need for information with resource considerations.
Is it possible to add more metrics to the Website Stats App?
Absolutely! The Website Stats App is designed to be extensible. You can add more metrics by modifying the website_stats()
function. For example, if you wanted to add a check for the presence of a specific element on the page, you could add the following code:
```python from selenium.webdriver.common.by import By from selenium.webdriver.support.ui import WebDriverWait from selenium.webdriver.support import expected_conditions as EC
# Inside the website_stats() function, after driver.get(website_link): try: element = WebDriverWait(driver, 10).until( EC.presence_of_element_located((By.ID, "myElement")) ) element_present = "Element found" except: element_present = "Element not found"
# Then include this in your Discord webhook message ```
This would check for the presence of an element with the ID "myElement" and report whether it was found or not.
How secure is the Website Stats App when it comes to handling sensitive information like webhook URLs?
The Website Stats App is designed with security in mind. It uses environment variables to store sensitive information like the Discord webhook URL and the website URL to be monitored. This approach prevents hardcoding of sensitive data directly into the script, which could be a security risk if the code were to be shared or exposed. However, it's important to ensure that your environment variables are properly secured on the system where the app is running. Additionally, when using the Website Stats App, make sure to follow best practices for securing your Discord webhook and consider using HTTPS for the monitored website to ensure secure communication.
Created: | Last Updated:
Here's a step-by-step guide on how to use the Website Stats App template:
Introduction
The Website Stats App is a powerful tool that provides detailed statistics about a specified website. It monitors the website's load time, status, and security level, and can automatically post updates to a Discord channel every 7 hours.
Getting Started
To begin using this template:
- Click the "Start with this Template" button in the Lazy Builder interface.
Initial Setup
Before running the app, you need to set up two environment secrets:
- Open the Environment Secrets tab in the Lazy Builder.
- Add the following secrets:
DISCORD_WEBHOOK_URL
: This is the webhook URL for your Discord channel.WEBSITE_URL
: The URL of the website you want to monitor.
To obtain a Discord webhook URL: 1. Open your Discord server settings. 2. Navigate to the "Integrations" tab. 3. Click on "Webhooks" and then "New Webhook". 4. Copy the webhook URL provided.
Test the App
Once you've set up the environment secrets:
- Click the "Test" button in the Lazy Builder interface.
- The app will start running, and you'll see output in the Lazy CLI.
Using the App
The Website Stats App will automatically run every 7 hours, providing the following information:
- Page Load Time
- Page Status (HTTP status code)
- Website Security (whether it uses HTTPS)
This information will be posted to your specified Discord channel via the webhook.
Integrating the App
This app doesn't require any additional integration steps. Once it's running, it will automatically post updates to your Discord channel at regular intervals.
To make the most of this app:
- Ensure your Discord webhook URL is correct and points to the desired channel.
- Make sure the website URL you've provided is accurate and includes the protocol (http:// or https://).
- Monitor your Discord channel for regular updates on your website's performance and status.
By following these steps, you'll have a powerful website monitoring tool that provides regular updates without any manual intervention.
Here are 5 key business benefits for this Website Stats App template:
Template Benefits
-
Automated Website Monitoring: Provides continuous, hands-off monitoring of website performance and availability, allowing businesses to quickly identify and address issues.
-
Enhanced Security Awareness: Regularly checks and reports on website security status, helping businesses maintain a secure online presence and protect customer data.
-
Performance Optimization: By tracking page load times, businesses can identify performance bottlenecks and optimize their websites for better user experience and improved search engine rankings.
-
Proactive Issue Resolution: With real-time Discord notifications, IT teams can respond quickly to website problems, minimizing downtime and potential revenue loss.
-
Compliance and Reporting: Generates consistent performance and security reports, which can be valuable for meeting industry regulations, client requirements, or internal quality standards.
Technologies


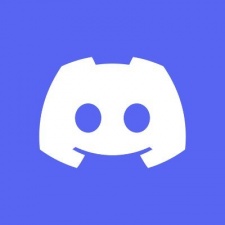