by davi
AI Web Chatbot
import logging
from flask import Flask, render_template, session
from flask_session import Session
from gunicorn.app.base import BaseApplication
from abilities import apply_sqlite_migrations
from app_init import create_initialized_flask_app
from models import db
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
# Flask app creation should be done by create_initialized_flask_app to avoid circular dependency problems.
app = create_initialized_flask_app()
# Configuring server-side session
app.config["SESSION_PERMANENT"] = False
app.config["SESSION_TYPE"] = "filesystem"
Session(app)
from abilities import llm
from flask import request, jsonify
Frequently Asked Questions
What kind of businesses can benefit from using this Web Based Chatbot with LLM template?
This Web Based Chatbot with LLM template is versatile and can benefit a wide range of businesses. It's particularly useful for: - Customer service departments looking to automate initial customer interactions - E-commerce platforms wanting to provide 24/7 product information and support - Educational institutions offering an AI tutor for students - Healthcare providers giving preliminary health information or appointment scheduling - Financial services offering basic account information and transaction support
The template's integration with a Large Language Model (LLM) allows for intelligent, context-aware responses, making it suitable for complex interactions across various industries.
How can I customize the chatbot's responses to align with my brand voice?
To customize the chatbot's responses in the Web Based Chatbot with LLM template, you can modify the LLM prompt and fine-tune the model. In the send_message
route of main.py
, you can adjust the conversation history and add specific instructions to the LLM. For example:
python
conversation_history = "Brand Voice: Professional and friendly. " + " ".join([f"user: {msg.get('user', '')} bot: {msg.get('bot', '')}" for msg in session['history']])
response = llm(prompt=conversation_history, response_schema={"type": "object", "properties": {"response": {"type": "string"}}}, model="gpt-4o", temperature=0.7)["response"]
You can also fine-tune the LLM on your brand's specific content to make responses more aligned with your voice and domain expertise.
What are the potential ROI benefits of implementing this chatbot solution?
Implementing the Web Based Chatbot with LLM template can offer several ROI benefits: - Reduced customer service costs by handling a significant portion of customer queries automatically - Increased customer satisfaction through 24/7 availability and quick response times - Improved lead generation and sales by providing instant product information and guiding customers through the sales funnel - Enhanced data collection on customer needs and preferences, informing business strategy - Freed up human resources to focus on more complex tasks and high-value interactions
While the exact ROI will vary by implementation, many businesses report significant cost savings and efficiency gains from chatbot solutions.
How can I extend the functionality of the chatbot to handle more complex tasks?
The Web Based Chatbot with LLM template can be extended to handle more complex tasks by integrating additional services and APIs. For example, you could add a product database lookup:
```python from product_database import get_product_info
@app.route("/send_message", methods=['POST']) def send_message(): user_message = request.json['message'] if 'product' in user_message.lower(): product_info = get_product_info(user_message) response = f"Here's the product information: {product_info}" else: # Existing LLM logic here return jsonify({"message": response}) ```
You can also implement more sophisticated natural language understanding to detect user intents and trigger specific actions or API calls based on those intents.
What measures are in place to ensure data privacy and security in this chatbot template?
The Web Based Chatbot with LLM template includes several measures to enhance data privacy and security: - It uses server-side sessions to manage conversation history, which helps prevent client-side tampering. - The template uses HTTPS by default when deployed properly, ensuring encrypted communication. - Database interactions use SQLAlchemy, which helps prevent SQL injection attacks. - The template doesn't store personal information by default, but if implemented, you should ensure compliance with relevant data protection regulations like GDPR or CCPA.
To further enhance security, you could implement user authentication, rate limiting, and regular security audits of your deployed chatbot.
Created: | Last Updated:
Here's a step-by-step guide on how to use the Web Based Chatbot with LLM template:
Introduction
This template provides a foundation for creating a web-based chatbot using a Large Language Model (LLM). It features a clean, responsive user interface built with Tailwind CSS and Flask for the backend. The chatbot maintains a conversation history and uses GPT-4 to generate responses.
Getting Started
- Click "Start with this Template" to begin using this template in the Lazy Builder interface.
Test the Application
-
Press the "Test" button in the Lazy Builder interface to start the deployment process.
-
Once the deployment is complete, you will receive a dedicated server link to access your chatbot application.
Using the Chatbot
-
Open the provided server link in your web browser. You'll see a simple chat interface with a message input box at the bottom.
-
Type your message in the input box and either press Enter or click the "Send" button to send your message to the chatbot.
-
The chatbot will process your message and respond. The conversation history is maintained for context, allowing for more coherent interactions.
Customizing the Chatbot
To customize your chatbot, you can modify the following aspects:
-
Update the chatbot's name: In the
template.html
file, locate the<span class="ml-3 text-xl">Your Chatbot</span>
line and change "Your Chatbot" to your preferred name. -
Adjust the LLM settings: In the
main.py
file, find thellm()
function call. You can modify parameters such astemperature
to control the randomness of responses. -
Extend conversation history: If you want to maintain a longer conversation history, adjust the limit in the
main.py
file. Look for the lineif len(session['history']) > 10:
and change the number as needed.
Integrating the Chatbot
This chatbot application runs as a standalone web service. If you want to integrate it into an existing website:
- Use an iframe to embed the chatbot:
```html
```
Replace YOUR_LAZY_APP_URL
with the actual URL provided by Lazy after deployment.
-
Alternatively, you can use the API endpoint to integrate the chatbot functionality into your own frontend:
-
Endpoint:
YOUR_LAZY_APP_URL/send_message
- Method: POST
- Headers:
Content-Type: application/json
- Body:
{"message": "Your message here"}
Example request using JavaScript fetch:
javascript
fetch('YOUR_LAZY_APP_URL/send_message', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({ message: 'Hello, chatbot!' }),
})
.then(response => response.json())
.then(data => console.log(data.message))
.catch((error) => console.error('Error:', error));
By following these steps, you'll have a functional web-based chatbot that you can use as-is or integrate into your existing projects.
Here are 5 key business benefits for this web-based chatbot template:
Template Benefits
-
Rapid Deployment of AI-Powered Customer Support: This template allows businesses to quickly set up an AI chatbot for customer service, reducing response times and operational costs while providing 24/7 support.
-
Customizable User Interface: With a clean, responsive design using Tailwind CSS, companies can easily customize the look and feel to match their brand, enhancing user experience and brand consistency.
-
Scalable Architecture: Built on Flask with Gunicorn, this template provides a solid foundation for scaling the chatbot service as user demand grows, ensuring reliable performance under increasing loads.
-
Persistent Conversation History: The implementation of server-side sessions allows for maintaining context in conversations, leading to more personalized and effective interactions with users.
-
Integration-Ready Backend: With a SQLite database and migration system in place, businesses can easily extend the chatbot's capabilities by integrating it with existing systems or adding new features like user authentication or data analytics.
Technologies
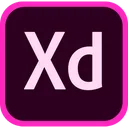
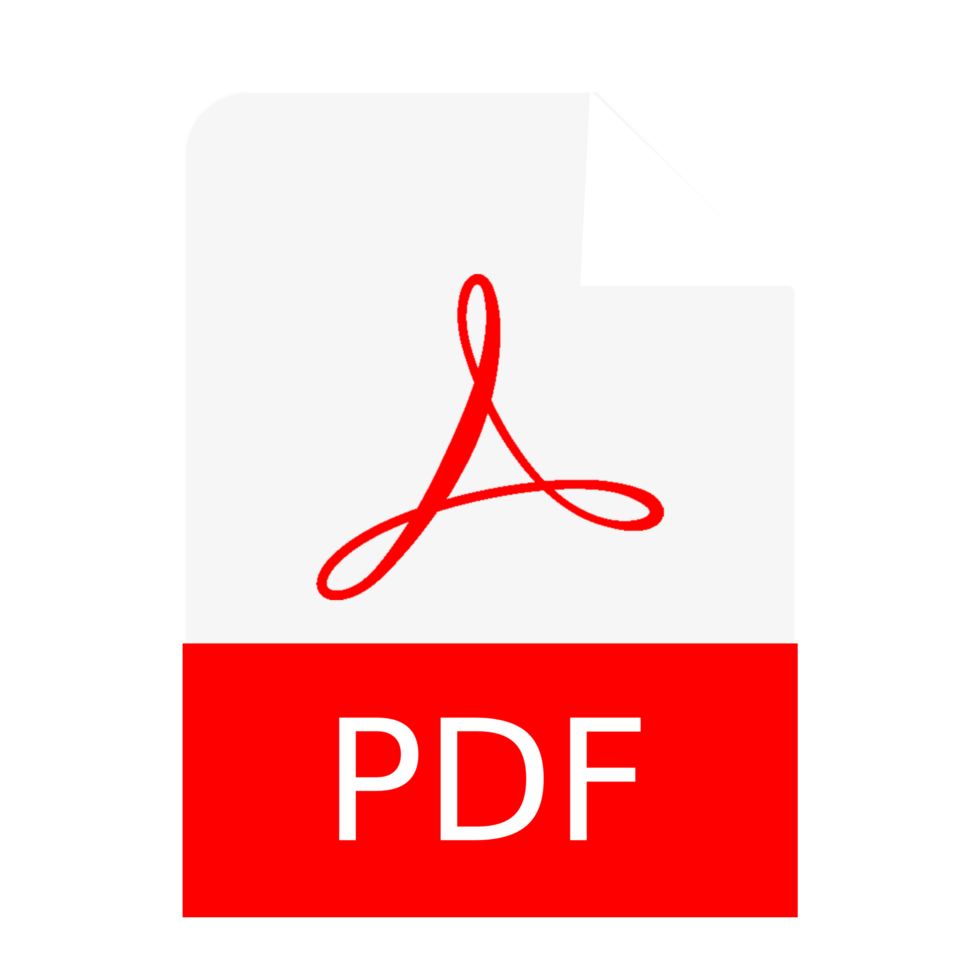

