by Lazy Sloth
Update Inventory Quantity with Shopify FastAPI
import uvicorn
from fastapi import FastAPI, HTTPException
from pydantic import BaseModel
import requests
import os
import json
app = FastAPI()
class InventoryUpdateData(BaseModel):
store_url: str
inventory_item_id: int
available_adjustment: int
location_id: int
class InventoryQueryData(BaseModel):
store_url: str
product_id: int
@app.post("/update_inventory", summary="Update Inventory", description="Endpoint to adjust the inventory level of a product.")
async def update_inventory(data: InventoryUpdateData):
# Get the Shopify admin API token from environment variables.
shopify_admin_api_token = os.environ.get('SHOPIFY_ADMIN_API_TOKEN')
Frequently Asked Questions
How can this Update Inventory Quantity with Shopify API template benefit my e-commerce business?
The Update Inventory Quantity with Shopify API template can significantly improve your inventory management process. It allows you to programmatically update inventory levels and retrieve inventory information, which can be crucial for maintaining accurate stock levels, preventing overselling, and ensuring customer satisfaction. This automation can save time, reduce errors, and help you make data-driven decisions about restocking and product availability.
Can I integrate this template with my existing inventory management system?
Yes, the Update Inventory Quantity with Shopify API template is designed to be flexible and can be integrated with existing inventory management systems. You can use the /update_inventory
endpoint to sync inventory changes from your system to Shopify, and the /get_inventory_level_id
endpoint to retrieve current inventory levels. This integration can help create a seamless flow of inventory data across your entire business ecosystem.
How does this template handle multi-location inventory management in Shopify?
The Update Inventory Quantity with Shopify API template supports multi-location inventory management through the location_id
parameter in the InventoryUpdateData
model. When updating inventory, you can specify the location for which you're adjusting the inventory. This allows you to manage stock levels across different warehouses or retail locations, ensuring accurate inventory tracking for each specific location in your Shopify store.
How can I modify the template to include error logging for failed API calls?
You can enhance the Update Inventory Quantity with Shopify API template to include error logging by modifying the error handling in the API call sections. Here's an example of how you could modify the update_inventory
function:
```python import logging
# Set up logging logging.basicConfig(filename='inventory_api.log', level=logging.ERROR)
@app.post("/update_inventory") async def update_inventory(data: InventoryUpdateData): # ... existing code ...
try:
response = requests.post(f'https://{data.store_url}/admin/api/2023-01/inventory_levels/adjust.json', headers=headers, json=payload)
response.raise_for_status()
return {"message": "Inventory adjusted successfully."}
except requests.exceptions.RequestException as e:
logging.error(f"Failed to adjust inventory: {str(e)}")
raise HTTPException(status_code=500, detail=f"Failed to adjust inventory: {str(e)}")
```
This modification adds error logging to capture any exceptions that occur during the API call, providing more detailed information for troubleshooting.
How can I extend the template to support batch inventory updates?
To support batch inventory updates in the Update Inventory Quantity with Shopify API template, you can create a new endpoint that accepts a list of inventory updates. Here's an example of how you could implement this:
```python from typing import List
class BatchInventoryUpdateData(BaseModel): store_url: str updates: List[InventoryUpdateData]
@app.post("/batch_update_inventory") async def batch_update_inventory(data: BatchInventoryUpdateData): results = [] for update in data.updates: try: result = await update_inventory(update) results.append({"success": True, "item": update.inventory_item_id, "message": result["message"]}) except HTTPException as e: results.append({"success": False, "item": update.inventory_item_id, "error": str(e.detail)}) return {"results": results} ```
This new endpoint allows you to send multiple inventory updates in a single API call, improving efficiency for bulk inventory adjustments.
Created: | Last Updated:
Introduction to the Inventory Update Template with Shopify API
Welcome to the Inventory Update Template with Shopify API! This template is designed to help you seamlessly update inventory quantities and retrieve inventory level IDs for products in a Shopify store. Whether you're a store owner, developer, or a non-technical builder, this guide will walk you through the process of using this template on the Lazy platform.
Getting Started
To begin using this template, simply click on "Start with this Template" on the Lazy platform. This will pre-populate the code in the Lazy Builder interface, so you won't need to copy, paste, or delete any code.
Initial Setup
Before you can use the template, you'll need to set up an environment secret within the Lazy Builder. This secret is the Shopify admin API token, which allows the app to authenticate with your Shopify store.
- Go to the Environment Secrets tab within the Lazy Builder.
- Create a new secret with the key
SHOPIFY_ADMIN_API_TOKEN
. - To obtain your Shopify admin API token, you'll need to create a private app in your Shopify admin. Follow the instructions provided by Shopify here to generate the credentials.
- Once you have your token, enter it as the value for the
SHOPIFY_ADMIN_API_TOKEN
secret.
Test
With the environment secret set, you're ready to test the app. Press the "Test" button on the Lazy platform. This will deploy the app and launch the Lazy CLI.
Entering Input
After pressing the "Test" button, the Lazy App's CLI interface will appear. If the app requires any user input, you will be prompted to provide it there. For this template, you will need to input details such as the store URL, inventory item ID, available adjustment, location ID, and product ID when prompted by the CLI.
Using the App
Once the app is running, you can interact with it using the provided API endpoints:
- To update inventory, send a POST request to the
/update_inventory
endpoint with the necessary data. - To get the inventory level ID, send a POST request to the
/get_inventory_level_id
endpoint with the required product information.
Lazy will provide you with a dedicated server link to use the API. Additionally, since this template uses FastAPI, you will also be provided with a docs link where you can interact with the API and see the available endpoints and their specifications.
Integrating the App
If you need to integrate this app with an external service or frontend, you can use the server link provided by Lazy. For example, you might want to call the API from a custom frontend application or integrate it with other tools that your Shopify store uses.
Here's a sample request to update inventory:
`POST /update_inventory HTTP/1.1
Host: [Your Lazy Server Link]
Content-Type: application/json
{
"store_url": "yourstore.myshopify.com",
"inventory_item_id": 1234567890,
"available_adjustment": 5,
"location_id": 1234567890
}`
And a sample response might look like this:
{
"message": "Inventory adjusted successfully."
}
Follow these steps to ensure your app is set up correctly and ready to manage inventory on your Shopify store. If you encounter any issues or have questions, the Lazy platform's customer support is available to assist you.
Here are 5 key business benefits for this template:
Template Benefits
-
Real-time Inventory Management: This template enables businesses to update their Shopify inventory in real-time, ensuring accurate stock levels across all sales channels. This helps prevent overselling and improves customer satisfaction.
-
Integration Capability: The template provides a foundation for integrating Shopify inventory management with other systems, such as warehouse management software or ERP systems, allowing for seamless data flow and improved operational efficiency.
-
Scalability: By leveraging FastAPI and Shopify's API, this template can handle high volumes of inventory updates, making it suitable for businesses of all sizes, from small e-commerce stores to large enterprises with complex inventory needs.
-
Custom Inventory Reporting: The ability to query inventory levels programmatically allows businesses to create custom reports and analytics, providing valuable insights into stock trends, turnover rates, and potential stockouts.
-
Automation Potential: This template serves as a starting point for building automated inventory management processes, such as triggering restock orders when inventory falls below a certain threshold or adjusting prices based on stock levels, leading to more efficient operations and reduced manual work.
Technologies
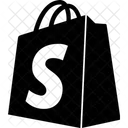
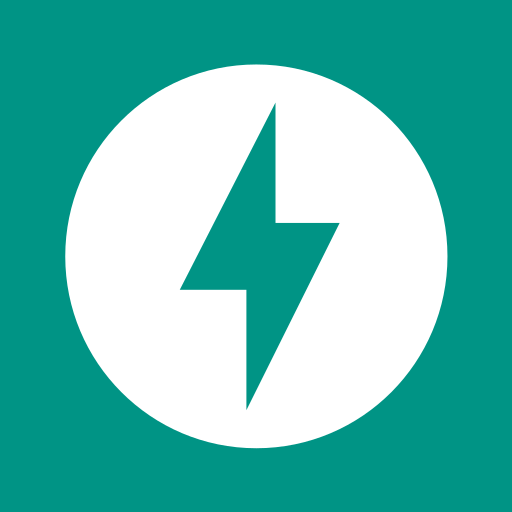