by Lazy Sloth
Get All Products with Shopify API
import uvicorn
from fastapi import FastAPI, HTTPException
from fastapi.middleware.cors import CORSMiddleware
from pydantic import BaseModel
import requests
import os
app = FastAPI()
# CORS configuration
origins = ["*"]
app.add_middleware(
CORSMiddleware,
allow_origins=origins,
allow_credentials=True,
allow_methods=["*"],
allow_headers=["*"],
)
class Data(BaseModel):
store_url: str
# Endpoint to get all products in JSON format.
Frequently Asked Questions
What are the main business benefits of using the Shopify Get All Products Downloader?
The Shopify Get All Products Downloader offers several key business benefits: - Efficient product data retrieval: It allows you to quickly fetch all product data from your Shopify store, which is crucial for inventory management and analysis. - Easy integration: The downloader can be easily integrated into existing systems, enabling seamless data flow between your Shopify store and other business tools. - Data-driven decision making: By having access to comprehensive product data, businesses can make more informed decisions about pricing, inventory, and marketing strategies.
How can the Shopify Get All Products Downloader be used to improve e-commerce operations?
The Shopify Get All Products Downloader can significantly enhance e-commerce operations in several ways: - Inventory synchronization: It allows for real-time synchronization of product data across multiple platforms or marketplaces. - Product catalog management: Easily export and manage your entire product catalog for backup or analysis purposes. - Custom storefront development: Use the retrieved product data to build custom storefronts or mobile apps with up-to-date product information.
Is the Shopify Get All Products Downloader suitable for large-scale Shopify stores?
Yes, the Shopify Get All Products Downloader is designed to handle large-scale Shopify stores efficiently. It uses pagination to retrieve products in batches of 250, which is the maximum allowed by the Shopify API. This ensures that all products are retrieved regardless of the store size, making it suitable for stores with thousands of products.
How can I modify the Shopify Get All Products Downloader to retrieve only specific product fields?
You can modify the API call in the get_all_products
function to include specific fields. Here's an example of how to retrieve only the id, title, and price of products:
python
params = {
'limit': 250,
'fields': 'id,title,variants'
}
if since_id:
params['since_id'] = since_id
response = requests.get(f'https://{store_url}/admin/api/2023-01/products.json', headers=headers, params=params)
This modification will reduce the amount of data transferred and processed, potentially improving performance for large stores.
Can the Shopify Get All Products Downloader be extended to include other Shopify resources?
Yes, the Shopify Get All Products Downloader can be extended to include other Shopify resources. You can create additional endpoints for different resources by following a similar pattern. For example, to add an endpoint for retrieving all orders:
python
@app.post("/orders", summary="Get All Orders", description="Endpoint to get all orders in JSON format.")
async def get_all_orders(data: Data):
# Similar implementation to get_all_products, but use the orders endpoint
response = requests.get(f'https://{store_url}/admin/api/2023-01/orders.json', headers=headers, params=params)
# Process and return orders
Remember to update the frontend code accordingly to use the new endpoint for downloading orders or other resources.
Created: | Last Updated:
Introduction to the Shopify API Get All Products Template
Welcome to the Shopify API Get All Products template! This template is designed to help you connect to a Shopify store, retrieve all products, and return them in JSON format. It's a FastAPI application that simplifies the process of interacting with the Shopify API. Additionally, it includes frontend JavaScript code to call the backend API and download the products. This guide will walk you through the steps to set up and use this template on the Lazy platform.
Getting Started
To begin using this template, click on "Start with this Template" on the Lazy platform. This will pre-populate the code in the Lazy Builder interface, so you won't need to copy, paste, or delete any code manually.
Initial Setup: Adding Environment Secrets
Before you can use the template, you'll need to set up some environment secrets. These are necessary for the app to authenticate with the Shopify API. Here's how to set them up:
- Log in to your Shopify admin dashboard.
- Go to the "Apps" section and create a new private app to obtain the API key and password.
- Once you have your Shopify API key and password, go to the Environment Secrets tab within the Lazy Builder.
- Add two new secrets:
SHOPIFY_API_KEY
andSHOPIFY_ADMIN_API_TOKEN
. - Enter the values you obtained from your Shopify admin dashboard for these secrets.
Test: Pressing the Test Button
With the environment secrets set up, you're ready to test the app. Press the "Test" button on the Lazy platform. This will deploy the app and launch the Lazy CLI. If the app requires any user input, you will be prompted to provide it through the Lazy CLI.
Using the App
Once the app is deployed, Lazy will provide you with a dedicated server link to use the API. You can interact with the app using this link. Additionally, if you're using FastAPI, Lazy will also provide a docs link where you can see the available endpoints and their specifications.
To use the backend API, you'll need to make a POST request to the /products
endpoint with the store URL in the request body. Here's a sample request:
`POST /products HTTP/1.1
Host: [BACKEND_URL_GENERATED_BY_LAZY]
Content-Type: application/json
{
"store_url": "YOUR_STORE_URL"
}`
And here's what a sample response might look like:
[
{
"id": 123456789,
"title": "Example Product",
"body_html": "<strong>Great product!</strong>",
"vendor": "Example Vendor",
"product_type": "Widgets",
"created_at": "2023-01-01T12:00:00-05:00",
// More product fields...
}
// More products...
]
Integrating the App
If you want to integrate the backend API with your frontend application, you can use the provided JavaScript code. Replace YOUR_STORE_URL
with the actual URL of your store and BACKEND_URL_GENERATED_BY_LAZY
with the backend URL provided by Lazy after deploying the app. This script will call the backend API and download the products as a JSON file when executed in your frontend application.
Here's the JavaScript code you can use in your frontend:
`async function downloadProducts() {
// Define the store URL.
const storeUrl = 'YOUR_STORE_URL';
// Define the API endpoint.
const apiEndpoint = 'BACKEND_URL_GENERATED_BY_LAZY/products';
// Define the request body.
const requestBody = {
store_url: storeUrl
};
try {
// Make the API call.
const response = await fetch(apiEndpoint, {
method: 'POST',
body: JSON.stringify(requestBody),
headers: {
'Content-Type': 'application/json'
}
});
// Get the products from the response.
const products = await response.json();
// Convert the products to a JSON string.
const productsJson = JSON.stringify(products, null, 2);
// Download the products as a JSON file.
const blob = new Blob([productsJson], {type: 'application/json'});
const link = document.createElement('a');
link.href = URL.createObjectURL(blob);
link.download = 'products.json';
link.click();
} catch (error) {
console.error('Error:', error);
}
}`
Follow these steps, and you'll be able to retrieve and download all products from your Shopify store using the Lazy platform. Happy building!
Here are 5 key business benefits for this template:
Template Benefits
-
Efficient Product Data Retrieval: This template allows businesses to quickly and efficiently retrieve all product data from their Shopify store, enabling easier management and analysis of their product catalog.
-
Data Integration Capabilities: By providing product data in JSON format, the template facilitates seamless integration with other systems or applications, such as inventory management tools or third-party marketplaces.
-
Scalability for Large Catalogs: The pagination feature ensures that even stores with thousands of products can retrieve their entire catalog without running into API limits or timeout issues.
-
Custom Frontend Integration: The included JavaScript code demonstrates how to easily integrate this functionality into any frontend application, allowing businesses to create custom interfaces for product data retrieval.
-
Automated Product Backup: This template can be used to create regular backups of product data, helping businesses safeguard their product information and quickly recover in case of data loss or system issues.
Technologies
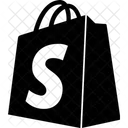
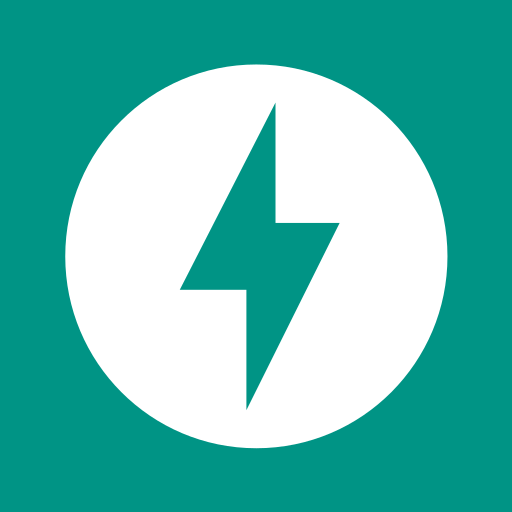