by Lazy Sloth
SecureUserAuthenticator
import logging
from flask import Flask
from flask_login import LoginManager
from gunicorn.app.base import BaseApplication
from routes import register_routes
from models import db, User
from migrations import run_migrations
def create_app():
app = Flask(__name__, static_folder='static')
app.secret_key = 'supersecretkey'
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///database.sqlite'
db.init_app(app)
login_manager = LoginManager()
login_manager.login_view = 'login'
login_manager.init_app(app)
@login_manager.user_loader
def load_user(user_id):
return User.query.get(int(user_id))
with app.app_context():
db.create_all()
SecureUserAuthenticator
Created: | Last Updated:
Introduction to the Secure User Authentication Template
The Secure User Authentication template helps you build a secure user authentication system for users to register, log in, and manage their profiles. This template lays the foundation for user-specific data management and permissions in a CMS.
Clicking Start with this Template
To get started with the template, click the "Start with this Template" button in the Lazy Builder interface.
Test
Press the "Test" button to begin the deployment of the app. The Lazy CLI will launch, and you will be prompted for any required user input.
Entering Input
The code requires user input through the CLI. After pressing the "Test" button, provide the following inputs when prompted:
- **Username**: Enter the username for registration. - **Email**: Enter the email address for registration. - **Password**: Enter the password for registration.
Using the App
Once the app is deployed, you can use the following features:
- **Home Page**: The home page provides links to log in or register if you are not logged in. If you are logged in, it displays a message indicating that you are logged in. - **Register Page**: Allows new users to register by providing a username, email, and password. - **Login Page**: Allows existing users to log in by providing their email and password. - **Profile Page**: Displays the logged-in user's profile information, including username and email.
Integrating the App
To integrate the app into your service or frontend, follow these steps:
1. **Access the App**: Use the server link provided by the Lazy CLI to access the app. 2. **API Endpoints**: If applicable, use the provided API endpoints to interact with the app. For example, you can use the `/login` endpoint to authenticate users.
Sample request for the login endpoint:
`POST /login
Content-Type: application/json
{
"email": "user@example.com",
"password": "password123"
}`
Sample response:
{
"message": "Login successful!",
"user": {
"id": 1,
"username": "user",
"email": "user@example.com"
}
}
By following these steps, you can successfully set up and integrate the Secure User Authentication template into your application.
Technologies
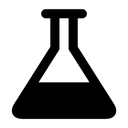
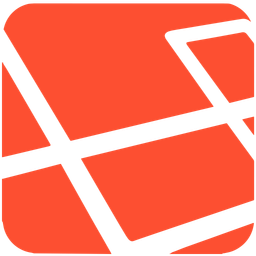

