by Lazy Sloth
Search Email with GMail API
import os
import json
import requests
from fastapi import FastAPI, HTTPException, Request, Depends, status
from fastapi.responses import JSONResponse, RedirectResponse
from google.oauth2.credentials import Credentials
from google_auth_oauthlib.flow import InstalledAppFlow
from google.auth.transport.requests import Request as GoogleRequest
from googleapiclient.discovery import build
from fastapi.security import HTTPBearer, HTTPAuthorizationCredentials
from fastapi.middleware.cors import CORSMiddleware
from starlette.responses import Response
app = FastAPI()
app.credentials = None
CLIENT_ID = os.environ["CLIENT_ID"]
CLIENT_SECRET = os.environ["CLIENT_SECRET"]
REDIRECT_URI = os.environ["REDIRECT_URI"]
SCOPE = "https://www.googleapis.com/auth/gmail.readonly"
from pydantic import BaseModel
Frequently Asked Questions
How can this Search Email with GMail API template benefit businesses?
The Search Email with GMail API template can significantly enhance business productivity by allowing companies to efficiently search and retrieve specific emails from their Gmail accounts. This can be particularly useful for customer service teams who need to quickly access communication history, sales teams tracking client interactions, or management reviewing important correspondence. The template's ability to search based on various parameters makes it a versatile tool for businesses of all sizes.
What are some potential use cases for this template in a corporate setting?
In a corporate setting, the Search Email with GMail API template can be utilized in several ways: - Automating email archiving and categorization - Generating reports on communication patterns - Assisting in compliance audits by quickly retrieving relevant emails - Supporting data analysis projects by providing easy access to email content - Integrating email search capabilities into existing business applications or dashboards
How does the Search Email with GMail API template ensure data security and privacy?
The Search Email with GMail API template incorporates OAuth2 authentication, ensuring that user credentials are securely handled. It uses environment variables for sensitive information like CLIENT_ID and CLIENT_SECRET, which helps prevent accidental exposure of these credentials. Additionally, the template only requests read-only access to Gmail data, further enhancing security. However, businesses should always review and potentially enhance the security measures based on their specific requirements and compliance needs.
How can I modify the Search Email with GMail API template to include additional email details in the search results?
You can modify the search_emails
function in the template to include additional email details. For example, to add the sender's email address to the results, you can update the emails_details.append()
section like this:
python
emails_details.append({
"id": email_response['id'],
"subject": email_subject,
"body": email_body,
"date": email_date,
"sender": next(header['value'] for header in email_response['payload']['headers'] if header['name'] == 'From')
})
This modification will include the sender's email address in the search results returned by the Search Email with GMail API template.
Can you explain how to implement error handling for API rate limits in the Search Email with GMail API template?
Certainly! To handle API rate limits, you can implement a retry mechanism with exponential backoff. Here's an example of how you could modify the search_emails
function:
```python import time from tenacity import retry, stop_after_attempt, wait_exponential
@retry(stop=stop_after_attempt(3), wait=wait_exponential(multiplier=1, min=4, max=10)) def make_api_request(url, headers): response = requests.get(url, headers=headers) if response.status_code == 429: # Too Many Requests raise Exception("Rate limit exceeded") return response
@app.post("/search_emails") async def search_emails(search_query: SearchQuery): # ... (existing code) try: response = make_api_request(search_url, headers) # ... (rest of the function) except Exception as e: raise HTTPException(status_code=429, detail="Rate limit exceeded. Please try again later.") ```
This modification to the Search Email with GMail API template adds a retry mechanism that will attempt the API request up to 3 times with exponential backoff if a rate limit error is encountered.
Created: | Last Updated:
Introduction to the Search Email with Gmail API Template
Welcome to the step-by-step guide on how to use the "Search Email with Gmail API" template on Lazy. This template is designed to help you build an application that can fetch and display emails from a Gmail account based on user-defined search queries. It leverages the Gmail API and FastAPI to create a robust backend service that can be integrated with other applications or used as a standalone tool.
Getting Started
To begin using this template, simply click on "Start with this Template" on the Lazy platform. This will pre-populate the code in the Lazy Builder interface, so you won't need to copy or paste any code manually.
Initial Setup
Before you can test and use the application, you need to set up some environment secrets within the Lazy Builder. These are necessary for OAuth2 authentication with the Gmail API. Here's what you need to do:
- Go to the "Environment Secrets" tab in the Lazy Builder.
- Set the following secrets with the respective values from your Google API credentials:
CLIENT_ID
: Your Google API client ID.CLIENT_SECRET
: Your Google API client secret.REDIRECT_URI
: The URI where you will receive the OAuth2 callback.
- To obtain these values, you need to create a project in the Google Developers Console, enable the Gmail API, and set up the OAuth consent screen. Then, create credentials for an OAuth 2.0 client ID, where you will get the
CLIENT_ID
andCLIENT_SECRET
. TheREDIRECT_URI
will be the endpoint in your application that receives the authentication code from Google.
For detailed instructions on setting up your Google API credentials, please refer to the Google Cloud documentation.
Test: Pressing the Test Button
Once you have set up the environment secrets, you can press the "Test" button on the Lazy platform. This will deploy your application and launch the Lazy CLI. If the application requires any user input, you will be prompted to provide it through the Lazy CLI.
Using the App
After deployment, Lazy will provide you with a dedicated server link to use the API. If you're using FastAPI, you will also receive a link to the API documentation, which will help you understand the available endpoints and how to interact with them.
To search for emails, you will use the /search_emails
endpoint. You can send a POST request to this endpoint with a JSON body containing the search query parameters. Here's an example of a search query:
{
"query": {
"from": "example@example.com",
"subject": "meeting"
}
}
The response will include a list of emails that match your search criteria, including details like the email's subject, body, and date.
Integrating the App
If you wish to integrate this backend service with a frontend or another tool, you can use the server link provided by Lazy. For instance, you can make HTTP requests from your frontend application to the deployed server to search and display emails.
Remember to handle the OAuth2 authentication flow in your frontend by redirecting users to the /oauth2callback
endpoint and handling the authentication code that Google provides.
By following these steps, you should be able to set up and use the "Search Email with Gmail API" template on Lazy to create an application that interacts with the Gmail API to search and display emails based on user-defined queries.
Template Benefits
-
Efficient Email Management: This template enables businesses to quickly search and retrieve specific emails based on custom queries, improving productivity and information retrieval efficiency.
-
Integration Capability: The FastAPI framework allows for easy integration with other business systems and applications, making it simple to incorporate email search functionality into existing workflows.
-
Secure Authentication: By utilizing OAuth2 for Gmail API access, the template ensures secure and authorized access to email data, maintaining user privacy and adhering to data protection standards.
-
Customizable Search Queries: The flexible search functionality allows businesses to create tailored search parameters, enabling precise filtering of emails based on various criteria such as date, sender, or content.
-
Scalable Architecture: Built on FastAPI, this template provides a foundation for a scalable email search solution that can handle growing data volumes and user requests, making it suitable for businesses of various sizes.
Technologies
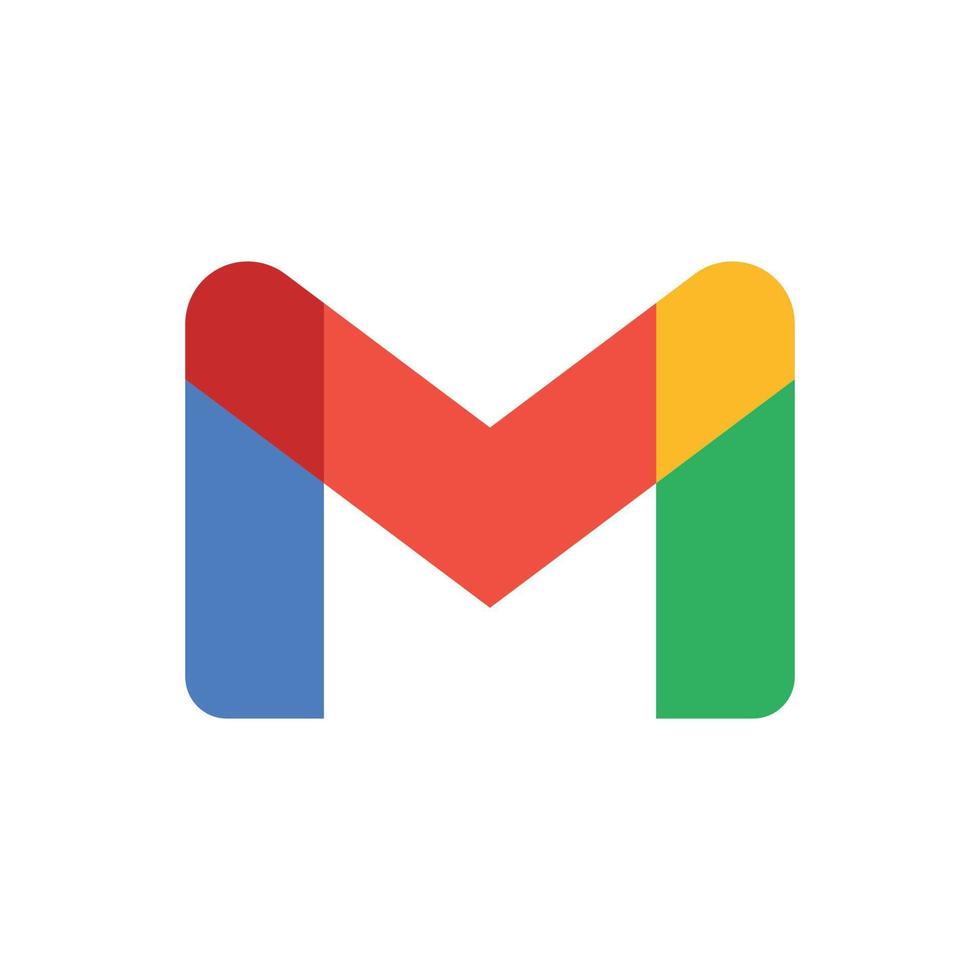
