Mr. Cash: Clicker Game with Rewards and Withdrawals
import os
import hashlib
import hmac
import json
import time
from flask import render_template, jsonify, redirect, url_for, request, session, make_response
from app_init import app
from models import UserStats, db
def validate_telegram_auth(auth_data):
# Extract hash and remove it from data for verification
check_hash = auth_data.pop('hash', None)
# Sort and create data check string
data_check_arr = [f"{key}={value}" for key, value in sorted(auth_data.items())]
data_check_string = "\n".join(data_check_arr)
# Get bot token from environment
bot_token = os.environ.get('TELEGRAM_API_TOKEN')
# Create secret key
secret_key = hashlib.sha256(bot_token.encode()).digest()
# Create hash signature
Frequently Asked Questions
Easy scalability for adding more games and features Q2: How can this template be monetized?
The template provides multiple revenue streams: - House edge on slot machine spins - Transaction fees on deposits/withdrawals - Premium features or VIP levels - Special bonus packages - Affiliate/referral programs through Telegram sharing
Q3: What industries besides gambling could adapt this template?
A: The template's structure can be adapted for: - Loyalty reward programs - Digital token distribution platforms - Play-to-earn gaming applications - Educational platforms with reward systems - Digital collectible marketplaces
Q4: How can I modify the slot machine winning logic to implement custom payout rates?
A: You can adjust the payout rates by modifying the checkWin
function in slot.js
:
```javascript function checkWin(results) { // Custom payout multipliers const payoutTable = { triple_diamond: 10, // 💎💎💎 triple_clover: 5, // 🍀🍀🍀 triple_cherry: 3, // 🍒🍒🍒 mixed_symbols: 1 // Any matching pair };
// Check for triple symbols
if (results.every(result => result.icon === results[0].icon)) {
return {
isWin: true,
multiplier: payoutTable[`triple_${results[0].icon}`] || payoutTable.mixed_symbols
};
}
return { isWin: false, multiplier: 0 };
} ```
Q5: How can I implement additional security measures for the Telegram authentication?
A: You can enhance the security by adding rate limiting and additional validation in the validate_telegram_auth
function:
```python from functools import wraps from flask import request import time
def rate_limit(max_requests=5, window=60): cache = {}
def decorator(f):
@wraps(f)
def wrapped(*args, kwargs):
user_id = request.args.get('id')
now = time.time()
# Clear old entries
cache = {k:v for k,v in cache.items() if now - v['timestamp'] < window}
if user_id in cache:
if cache[user_id]['count'] >= max_requests:
raise ValueError('Too many login attempts')
cache[user_id]['count'] += 1
else:
cache[user_id] = {'count': 1, 'timestamp': now}
return f(*args, kwargs)
return wrapped
return decorator
@rate_limit() def validate_telegram_auth(auth_data): # Existing validation code... pass ```
This adds rate limiting to prevent brute force attacks on the authentication endpoint.
Created: | Last Updated:
Getting Started with the Telegram Slot Casino Template
This template creates a slot machine casino game that integrates with Telegram for user authentication and includes TON wallet connectivity features.
Initial Steps
- Click "Start with this Template" to begin
- Set up the following environment secrets in the Environment Secrets tab:
TELEGRAM_API_TOKEN
- Your Telegram bot token (get this from @BotFather)TELEGRAM_BOT_USERNAME
- Your Telegram bot's username without the @ symbol
Setting Up Your Telegram Bot
- Open Telegram and message @BotFather
- Send
/newbot
to create a new bot - Choose a name and username for your bot
- Copy the API token provided by BotFather
- In BotFather, configure your bot:
- Send
/setdomain
and select your bot - After deployment, paste your app's domain URL to enable Telegram login
Testing the App
- Click the "Test" button to deploy your application
- Lazy will provide you with a server URL for your app
Using the Casino App
The casino app includes:
- Telegram login integration
- Slot machine game with various symbols and rewards
- Account management system
- TON wallet connection capability
- Deposit and withdrawal functionality (coming soon)
Players can:
- Login with their Telegram account
- Play the slot machine with different symbol combinations
- View their balance and transaction history
- Connect their TON wallet
- Access their account dashboard
Game Features
The slot machine includes:
- Multiple symbols with different values
- Special combinations for higher rewards
- Initial balance of 100 USDT
- Fixed bet amount of 10 USDT per spin
Winning combinations: * Three matching symbols * Special combinations with 💎 and 🍀 symbols * Multipliers based on symbol values
The template provides a complete casino gaming experience with Telegram authentication and blockchain wallet integration capabilities.
Template Benefits
- Secure Authentication Integration
- Implements robust Telegram authentication system with hash validation
- Provides secure user session management and data protection
- Reduces fraud risk through verified user identities
-
Enables seamless login/logout functionality
-
Monetization Ready Platform
- Built-in wallet and balance management system
- Ready-to-implement deposit and withdrawal functionality
- Supports cryptocurrency transactions (TON wallet integration)
-
Multiple revenue stream opportunities through in-game purchases
-
User Engagement & Retention
- Interactive slot machine gameplay mechanics
- Reward system with daily streaks and special combinations
- Social sharing features to drive organic growth
-
Progress tracking and statistics for user engagement
-
Scalable Architecture
- Modular codebase with clear separation of concerns
- Database migration system for easy updates
- Responsive design that works across devices
-
Well-structured frontend and backend integration
-
Cross-Platform Integration
- Seamless integration with Telegram's ecosystem
- TON blockchain compatibility for crypto transactions
- Web-based interface accessible from any browser
- Easy expansion to additional platforms and services
Technologies
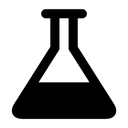

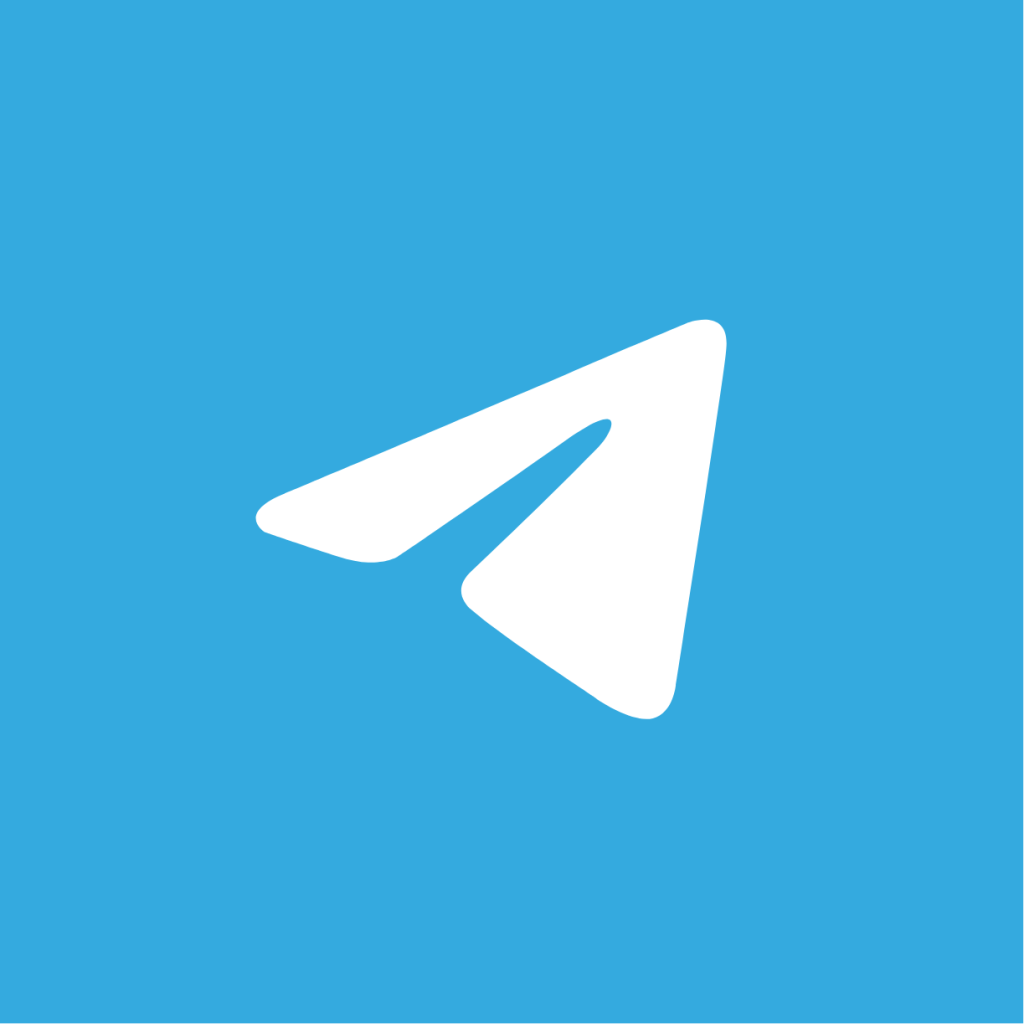
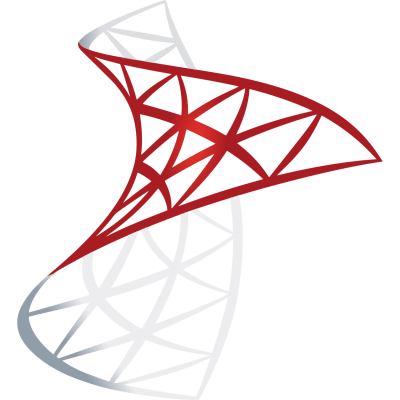