M3 Moulin Moderne Customer Engagement Portal
import logging
from gunicorn.app.base import BaseApplication
from app_init import create_initialized_flask_app
# Flask app creation should be done by create_initialized_flask_app to avoid circular dependency problems.
app = create_initialized_flask_app()
# Setup logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
class StandaloneApplication(BaseApplication):
def __init__(self, app, options=None):
self.application = app
self.options = options or {}
super().__init__()
def load_config(self):
# Apply configuration to Gunicorn
for key, value in self.options.items():
if key in self.cfg.settings and value is not None:
self.cfg.set(key.lower(), value)
def load(self):
Frequently Asked Questions
How can the M3 Moulin Moderne Customer Engagement Portal benefit our business?
The M3 Moulin Moderne Customer Engagement Portal can significantly enhance your business by: - Showcasing your products to a wider audience - Providing easy access to company information, building trust with customers - Offering a direct communication channel through the contact form - Improving brand visibility and online presence - Facilitating customer feedback and inquiries, leading to better customer service
Can we customize the product listings on the portal?
Absolutely! The M3 Moulin Moderne Customer Engagement Portal is designed to be easily customizable. You can modify the product listings in the home.html
file. For example, to add a new product, you can insert the following code within the "Products Section":
```html

Your New Product
Description of your new product.
```
This flexibility allows you to keep your product offerings up-to-date and relevant to your customers.
How does the contact form in the M3 Moulin Moderne Customer Engagement Portal work?
The contact form in the portal is designed to collect customer inquiries and feedback. When a customer submits the form:
How can we add new pages to the M3 Moulin Moderne Customer Engagement Portal?
To add new pages to the portal, you'll need to:
What measures are in place to ensure the security of customer data submitted through the M3 Moulin Moderne Customer Engagement Portal?
The M3 Moulin Moderne Customer Engagement Portal incorporates several security measures: - HTTPS encryption (to be implemented on deployment) to protect data in transit - Server-side validation of form inputs to prevent malicious data submission - Use of Flask's built-in CSRF protection for form submissions - Proper error handling to avoid exposing sensitive information
Additionally, when implementing the email sending or database storage logic for the contact form, it's crucial to follow best practices for data protection and comply with relevant data privacy regulations.
Created: | Last Updated:
Introduction to the Template
This template is designed to create a web portal for M3 Moulin Moderne to enhance customer engagement and satisfaction. The portal features product listings, company information, and a contact form. The template includes both mobile and desktop headers, a home page with product and company information, and a contact form. It also includes the necessary backend setup using Flask.
Clicking Start with this Template
To get started with this template, click Start with this Template in the Lazy Builder interface.
Test
Press the Test button to begin the deployment of the app. The Lazy CLI will handle the deployment process and prompt you for any required user input.
Using the App
Once the app is deployed, you can use the following features:
Header
The header includes both mobile and desktop versions: - Mobile Header: A button to toggle the mobile menu. - Desktop Header: A navigation bar with links.
Home Page
The home page includes: - Welcome Section: A welcome message. - Products Section: A list of products with images and descriptions. - About Section: Information about the company. - Contact Form: A form to submit contact information.
Contact Form
The contact form allows users to submit their name, email, and message. The form data is sent to the backend, which processes the submission and returns a success message.
Integrating the App
Backend Setup
The backend is set up using Flask. The main components include: - main.py: Initializes and runs the Flask app using Gunicorn. - routes.py: Defines the routes for the home page and contact form submission. - app_init.py: Initializes the Flask app and registers routes.
External Integration Steps
- Email Sending Logic: Implement the email sending logic in the
submit_contact
route inroutes.py
. You can use an email service like SendGrid or SMTP to send emails.
Example using SendGrid: ```python import sendgrid from sendgrid.helpers.mail import Mail
def send_email(name, email, message): sg = sendgrid.SendGridAPIClient(api_key='YOUR_SENDGRID_API_KEY') email_content = f"Name: {name}\nEmail: {email}\nMessage: {message}" mail = Mail( from_email='your-email@example.com', to_emails='recipient@example.com', subject='New Contact Form Submission', plain_text_content=email_content ) response = sg.send(mail) return response.status_code ```
-
Static Files: Ensure that the static files (CSS and JS) are correctly placed in the
static
folder. -
Database Setup: If you need to store contact form submissions in a database, set up a database and configure it in
app_init.py
.
Example using SQLAlchemy: ```python from flask_sqlalchemy import SQLAlchemy
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///your_database.db' db = SQLAlchemy(app)
class ContactFormSubmission(db.Model): id = db.Column(db.Integer, primary_key=True) name = db.Column(db.String(100)) email = db.Column(db.String(100)) message = db.Column(db.Text)
db.create_all() ```
- Environment Secrets: If the code requires any environment secrets (e.g., API keys), set them up in the Environment Secrets tab within the Lazy Builder.
Conclusion
This template provides a comprehensive solution for creating a customer engagement portal for M3 Moulin Moderne. By following the steps outlined above, you can deploy and customize the app to meet your specific needs. If you need to integrate additional features or services, refer to the provided code examples and documentation links.
Here are 5 key business benefits for the M3 Moulin Moderne Customer Engagement Portal template:
Template Benefits
-
Enhanced Customer Engagement: The portal provides an interactive platform for customers to learn about products, company history, and easily contact M3 Moulin Moderne, fostering stronger customer relationships.
-
Improved Brand Visibility: With a professional and visually appealing website, M3 Moulin Moderne can enhance its online presence and brand image, potentially attracting new customers and partners.
-
Streamlined Communication: The integrated contact form allows for efficient customer inquiries and feedback collection, enabling the company to respond promptly and improve customer service.
-
Product Showcase: The dedicated products section effectively highlights M3 Moulin Moderne's offerings, potentially increasing sales and customer awareness of the full product range.
-
Mobile Responsiveness: The template's responsive design ensures a seamless experience across devices, catering to the growing number of mobile users and improving accessibility for all customers.
Technologies
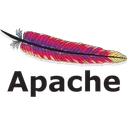