by davi
Web app with Google / Magic link sign in
import logging
from flask import Flask, url_for
from gunicorn.app.base import BaseApplication
from routes import routes as routes_blueprint
from models import db, User
from abilities import apply_sqlite_migrations, flask_app_authenticator
# Setup logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
def create_app():
app = Flask(__name__, static_folder='static')
app.secret_key = 'supersecretkey'
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///database.sqlite'
db.init_app(app)
with app.app_context():
apply_sqlite_migrations(db.engine, db.Model, 'migrations')
app.register_blueprint(routes_blueprint)
# Set up authentication
app.before_request(flask_app_authenticator(
Frequently Asked Questions
What types of businesses or applications would benefit most from using this Flask web app template with authentication?
This Flask web app template with authentication is ideal for a variety of businesses and applications, particularly those developing SaaS (Software as a Service) products. It's well-suited for: - Startups building user-centric web applications - Freelancers or agencies creating client portals - E-learning platforms requiring user accounts - Personal finance or budgeting tools - Health and fitness tracking apps
The template provides a solid foundation for any application where users need to manage their own data securely, making it a versatile starting point for many web-based services.
How does this template differ from an internal tool skeleton, and why might a business choose this one instead?
This Flask web app template differs from an internal tool skeleton in that it doesn't include team features. Instead, it's designed for applications where multiple users manage their own individual data. A business might choose this template over an internal tool skeleton when: - Developing a product for a broad user base rather than for internal team use - Creating a service where user privacy and data separation are crucial - Building a scalable SaaS application that needs to handle many independent user accounts - Focusing on individual user experiences rather than collaborative features
For example, if you're building a personal journal app or a individual task management tool, this template would be more appropriate than an internal tool skeleton.
What are the potential time and cost savings of using this Flask web app template for a startup?
Using this Flask web app template can provide significant time and cost savings for a startup: - Reduced development time: The authentication system is already set up, saving potentially weeks of work. - Lower initial costs: By starting with a pre-built structure, startups can allocate more budget to unique features. - Faster time-to-market: With core functionalities in place, products can launch sooner. - Reduced security risks: The template includes basic security measures, minimizing the risk of early vulnerabilities. - Scalability: The structure supports growth, preventing costly rewrites as the user base expands.
These factors can help startups conserve resources and focus on developing their core business logic and unique value propositions.
How can I customize the user model in this Flask web app template to include additional fields?
To customize the user model in this Flask web app template, you'll need to modify the User
class in the models.py
file. Here's an example of how you might add additional fields:
```python from flask_sqlalchemy import SQLAlchemy
db = SQLAlchemy()
class User(db.Model): id = db.Column(db.Integer, primary_key=True) email = db.Column(db.String(150), unique=True, nullable=False) profile_picture = db.Column(db.String(255)) # New fields first_name = db.Column(db.String(50)) last_name = db.Column(db.String(50)) date_of_birth = db.Column(db.Date) subscription_tier = db.Column(db.String(20)) ```
After modifying the model, you'll need to create and apply a new migration to update the database schema. Remember to update any related functions in database_operations.py
to handle these new fields.
How can I add a new route and template to this Flask web app?
To add a new route and template to this Flask web app, follow these steps:
Created: | Last Updated:
Here's a step-by-step guide on how to use the Login and Registration template:
Introduction
This template provides a solid foundation for building a web application with user authentication functionality. It includes features for user login, registration, and profile management using Flask, SQLAlchemy, and Google authentication.
Getting Started
-
Click "Start with this Template" to begin using this template in the Lazy Builder interface.
-
Press the "Test" button to initiate the deployment process. This will launch the Lazy CLI and start the application.
-
Once the deployment is complete, you'll receive a dedicated server link to access your application.
Using the App
After deployment, you can access your application through the provided server link. The app includes the following features:
- User authentication with Google
- User profile management
- Secure session handling
- Responsive design for both desktop and mobile devices
Home Page
The home page (/
) displays a welcome message with the user's name or email address if they're logged in. If not logged in, users will be redirected to the Google authentication page.
Logout
Users can log out by clicking the "Logout" button in the navigation bar, which will clear their session and redirect them to the home page.
Customizing the App
You can customize various aspects of the application:
- App Title: Change the
app_title
parameter in theflask_app_authenticator
function call inmain.py
:
python
app.before_request(flask_app_authenticator(
allowed_domains=None,
allowed_users=None,
logo_path=None,
app_title="Your Custom App Title",
custom_styles=None,
session_expiry=None
))
-
Logo: Update the
logo_path
parameter in the same function call to use a custom logo. -
Styling: Modify the
styles.css
file to change the app's appearance. The template uses Tailwind CSS for styling. -
Database Schema: If you need to modify the user table or add new tables, update the
models.py
file and create new migration files in themigrations
folder.
Integrating the App
This template provides a standalone web application with user authentication. To integrate it with other services or expand its functionality, you can:
-
Add new routes in the
routes.py
file to create additional pages or API endpoints. -
Extend the
User
model inmodels.py
to include more user-related data. -
Implement additional database operations in
database_operations.py
to interact with your data. -
Create new HTML templates in the
templates
folder for new pages or features.
Remember that this template handles user authentication and basic user management out of the box, allowing you to focus on building your application's unique features on top of this foundation.
Template Benefits
-
Rapid SaaS Application Development: This template provides a solid foundation for quickly building Software-as-a-Service (SaaS) applications, allowing businesses to launch new products or services faster and with less initial development overhead.
-
Secure User Authentication: The built-in authentication system ensures that user data is protected, which is crucial for maintaining customer trust and complying with data protection regulations in various industries.
-
Scalable Database Architecture: With SQLAlchemy integration and migration support, the template offers a scalable database structure that can grow with the business, accommodating increasing user numbers and data complexity over time.
-
Responsive Design Ready: The inclusion of Tailwind CSS and responsive design elements means that applications built on this template will work well on both desktop and mobile devices, catering to a wider user base and improving user experience.
-
Easy Customization and Expansion: The modular structure of the template, with separate files for routes, models, and database operations, makes it easy for developers to customize the application and add new features as business needs evolve, reducing long-term development costs.
Technologies
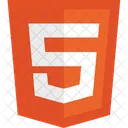