Web app with landing page and log in
import logging
from flask import Flask, url_for, request, session
from gunicorn.app.base import BaseApplication
from routes import routes as routes_blueprint
from authentication import auth, auth_required
from models import db, User
from abilities import apply_sqlite_migrations
# Setup logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
def create_app():
app = Flask(__name__, static_folder='static')
app.secret_key = 'supersecretkey'
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:///database.sqlite'
db.init_app(app)
with app.app_context():
apply_sqlite_migrations(db.engine, db.Model, 'migrations')
app.register_blueprint(routes_blueprint)
app.register_blueprint(auth)
Created: | Last Updated:
Web App Template with Landing Page and Login
This template provides a starting point for building a web application with both public-facing pages and authenticated sections. It includes a landing page for public access and a protected home page that requires authentication.
Getting Started
- Click "Start with this Template" to begin using this template in the Lazy Builder
Testing the App
- Click the "Test" button in the Lazy Builder
- Once deployed, you'll receive a server link to access your web application
Using the App
The template provides two main sections:
- A public landing page accessible to all visitors
- A protected home page that requires authentication
The landing page includes: * Navigation header with logo * Hero section with a "Get Started" button * Mobile-responsive design
The authenticated section includes: * Sidebar navigation with user profile * Logout functionality * Protected routes that require login
To customize the app:
- Update the app title in
app_init.py
by changing theAPP_TITLE
value - Modify the theme by changing the
THEME
value inapp_init.py
to any DaisyUI theme - Add your own logo by placing a
logo.png
file in the static folder - Build out the content of
home.html
based on your app's specific needs
The template uses Google authentication by default and will automatically create user profiles in the database when users log in.
Next Steps
To build your application:
* Add your specific content and functionality to the home.html
template
* Customize the landing page content in landing.html
* Add additional routes in routes.py
as needed
* Extend the User model in models.py
with any additional fields your app requires
The template provides a solid foundation for building a web application with both public and authenticated sections, suitable for SaaS applications or any service requiring user authentication.
Template Benefits
- Rapid SaaS Platform Development
- Perfect foundation for building subscription-based web applications
- Includes essential authentication and user management features
-
Reduces time-to-market for new SaaS products
-
Professional Customer-Facing Portal
- Clean, modern landing page design for attracting potential customers
- Seamless transition between public and private areas
-
Professional user interface with responsive design
-
Secure User Management System
- Built-in authentication and session management
- Database structure for user profiles and credentials
-
Protected routes for sensitive content
-
Scalable Architecture
- Configured with Gunicorn for production-grade deployment
- Multi-worker setup for handling concurrent users
-
Organized codebase structure for easy maintenance and scaling
-
Customizable Branding Integration
- Dynamic theme support through DaisyUI
- Easy logo and brand color customization
- Consistent styling across public and private pages
Technologies
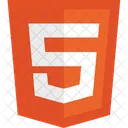

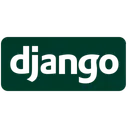
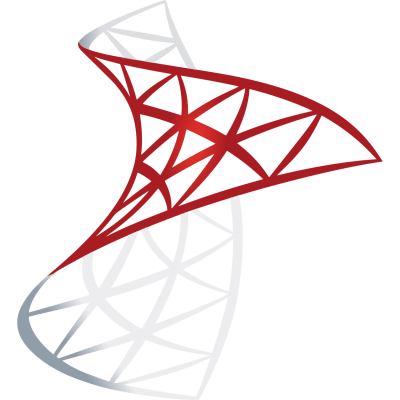
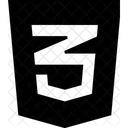

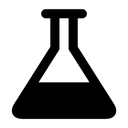