by tresson
Investment Growth Simulator
import logging
from gunicorn.app.base import BaseApplication
from app_init import create_initialized_flask_app
# Flask app creation should be done by create_initialized_flask_app to avoid circular dependency problems.
app = create_initialized_flask_app()
# Setup logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
class StandaloneApplication(BaseApplication):
def __init__(self, app, options=None):
self.application = app
self.options = options or {}
super().__init__()
def load_config(self):
# Apply configuration to Gunicorn
for key, value in self.options.items():
if key in self.cfg.settings and value is not None:
self.cfg.set(key.lower(), value)
def load(self):
Frequently Asked Questions
How can businesses benefit from using the Investment Growth Simulator?
The Investment Growth Simulator can be a valuable tool for financial institutions, investment advisors, and educational platforms. It allows businesses to demonstrate the potential growth of investments over time, helping clients visualize the impact of different interest rates and investment terms. This can aid in client education, financial planning, and marketing efforts for investment products.
Can the Investment Growth Simulator be customized for different currencies?
Yes, the Investment Growth Simulator is designed with flexibility in mind. It includes an exchange rate input, allowing users to see results in both USD and their local currency. This makes it easy for businesses to adapt the tool for international clients or to compare investment growth across different currencies.
How can the Investment Growth Simulator be integrated into a larger financial planning application?
The Investment Growth Simulator can be seamlessly integrated into a larger financial planning application as a module. Its modular design allows it to be easily incorporated alongside other tools such as retirement calculators, budget planners, or portfolio analyzers. This integration can provide a comprehensive financial planning experience for users.
How can I modify the Investment Growth Simulator to include additional investment parameters?
The Investment Growth Simulator can be easily modified to include additional parameters. For example, to add a monthly contribution option, you would need to update both the HTML form and the calculation logic. In the home.html
file, add a new input field:
```html
```
Then, in the routes.py
file, update the calculate_investment
function to include the monthly contribution in the calculations:
python
monthly_contribution = float(request.form['monthly-contribution'])
# ... existing code ...
for month in range(1, total_months + 1):
interest_earned = current_balance * monthly_interest_rate
current_balance += monthly_contribution # Add monthly contribution
# ... rest of the calculation logic ...
Can the Investment Growth Simulator handle different compounding frequencies?
Currently, the Investment Growth Simulator uses monthly compounding. To add support for different compounding frequencies, you would need to modify the calculation logic in the routes.py
file. Here's an example of how you could adjust the code to support annual, semi-annual, quarterly, and monthly compounding:
```python @app.route("/calculate", methods=['POST']) def calculate_investment(): # ... existing code ... compounding_frequency = request.form['compounding-frequency']
if compounding_frequency == 'annual':
periods_per_year = 1
elif compounding_frequency == 'semi-annual':
periods_per_year = 2
elif compounding_frequency == 'quarterly':
periods_per_year = 4
else: # monthly
periods_per_year = 12
periodic_rate = annual_interest_rate / periods_per_year
total_periods = investment_term * periods_per_year
for period in range(1, total_periods + 1):
interest_earned = current_balance * periodic_rate
# ... rest of the calculation logic ...
```
You would also need to add a dropdown in the HTML form to allow users to select the compounding frequency. This enhancement would make the Investment Growth Simulator more versatile and accurate for various investment scenarios.
Created: | Last Updated:
Introduction to the Investment Growth Simulator Template
The Investment Growth Simulator is an interactive tool designed to help users simulate the growth of their investments over time. Users can input their initial investment amount, annual interest rate, and other parameters to see a detailed monthly breakdown of their investment growth. This template includes a responsive header for both mobile and desktop views, a form for user input, and a table to display the results.
Getting Started
To get started with the Investment Growth Simulator template, follow these steps:
- Click "Start with this Template": This will load the template into the Lazy Builder interface.
Test the Application
- Press the Test Button: This will deploy the app and launch the Lazy CLI. The CLI will guide you through any required user input.
Entering Input
After pressing the Test button, you will be prompted to provide the following inputs through the Lazy CLI:
- Initial Investment Amount (USD)
- Annual Interest Rate (%)
- Investment Term (Years)
- Exchange Rate (USD to Local Currency)
These inputs are required to simulate the investment growth.
Using the App
Once the app is deployed, you can interact with it through the provided interface:
- Header: The header includes a responsive navigation menu that adapts to both mobile and desktop views.
- Form: Fill out the form with your investment details:
- Initial Investment Amount (USD)
- Annual Interest Rate (%)
- Investment Term (Years)
- Exchange Rate (USD to Local Currency)
- Calculate: Click the "Calculate" button to submit the form and see the results.
- Results: The results will be displayed in a table format, showing a monthly breakdown of your investment growth.
Integrating the App
If you need to integrate this app with other tools or services, follow these steps:
- API Endpoint: The app provides an endpoint
/calculate
to handle investment calculations. You can make a POST request to this endpoint with the required parameters to get the investment growth data.
Sample Request
bash
curl -X POST http://<your-app-url>/calculate \
-F 'initial-amount=10000' \
-F 'annual-interest-rate=5' \
-F 'investment-term=5' \
-F 'exchange-rate=1.2'
Sample Response
json
[
{
"month": 1,
"date": "Jan 2023",
"initial_balance_usd": 10000.00,
"interest_earned_usd": 41.67,
"adjustment_usd": 0.00,
"ending_balance_usd": 10041.67,
"ending_balance_local": 12050.00
},
...
]
Conclusion
The Investment Growth Simulator template is a powerful tool for visualizing the growth of investments over time. By following the steps outlined in this article, you can easily deploy and use the app to simulate various investment scenarios. If you need to integrate the app with other tools, use the provided API endpoint to fetch investment growth data programmatically.
Template Benefits
-
Financial Education Tool: This template serves as an excellent educational resource for financial institutions, helping clients visualize the potential growth of their investments over time. It can be used to demonstrate the power of compound interest and long-term investing strategies.
-
Customer Engagement: Banks and investment firms can use this interactive simulator to engage potential customers, allowing them to experiment with different investment scenarios. This hands-on approach can help convert leads into active investors.
-
Product Marketing: Financial product marketers can utilize this tool to showcase the benefits of specific investment products or services. By inputting product-specific parameters, they can illustrate the potential returns and advantages of their offerings.
-
Financial Planning Aid: Financial advisors and wealth management professionals can leverage this simulator to assist clients in setting realistic investment goals and creating personalized financial plans based on different scenarios and risk tolerances.
-
Decision Support System: For internal use, financial institutions can adapt this template to create a decision support tool for their staff, helping them quickly assess and compare different investment options or strategies for clients, leading to more informed recommendations.
Technologies
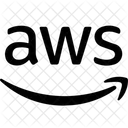