by Lazy Sloth
Stripe page for Wordpress
import os
import logging
from fastapi import FastAPI, HTTPException
from fastapi.middleware.cors import CORSMiddleware
from pydantic import BaseModel, Field
import stripe
import uvicorn
# Constants
STRIPE_SECRET_KEY = os.environ['STRIPE_SECRET_KEY']
YOUR_DOMAIN = os.environ['YOUR_DOMAIN']
# Configure Stripe API key
stripe.api_key = STRIPE_SECRET_KEY
# FastAPI app initialization
app = FastAPI()
# CORS configuration
origins = ["*"]
app.add_middleware(
CORSMiddleware,
allow_origins=origins,
allow_credentials=True,
Created: | Last Updated:
Introduction to the Stripe Integration Template for WordPress
This template is designed to help you integrate Stripe payment processing into a WordPress site. It provides a backend service using FastAPI to create and manage Stripe checkout sessions, and frontend code to embed the Stripe checkout experience into your WordPress page. This guide will walk you through the steps to set up and use this template on the Lazy platform.
Getting Started
To begin using this template, click "Start with this Template" on the Lazy platform. This will pre-populate the code in the Lazy Builder interface, so you won't need to copy or paste any code manually.
Initial Setup
Before testing the app, you'll need to set up some environment secrets within the Lazy Builder:
- STRIPE_SECRET_KEY: Your Stripe secret API key. You can find this in your Stripe dashboard under Developers > API keys.
- YOUR_DOMAIN: The domain of your website where the Stripe checkout will be redirected after payment. For example, "https://www.yourwebsite.com".
These values are not stored in your operating system but are set in the Environment Secrets tab within the Lazy Builder.
Test: Pressing the Test Button
Once you have set up the environment secrets, press the "Test" button in the Lazy Builder. This will deploy the app and launch the Lazy CLI. If the code requires any user input, you will be prompted to provide it through the Lazy CLI.
Entering Input
If the code requires user input, you will be prompted for it after pressing the "Test" button. Follow the instructions in the Lazy CLI to enter the necessary information.
Using the App
After deployment, Lazy will provide you with a dedicated server link to use the API. If you're using FastAPI, Lazy will also provide a link to the API documentation.
Integrating the App into WordPress
To integrate the backend service into your WordPress site, you'll need to add the provided frontend code to your WordPress payment page. Here's how:
- Log in to your WordPress dashboard and navigate to the page where you want to add the payment functionality.
- Edit the page and insert the provided frontend script just before the
</body>
tag. - Replace
"PUBLISHABLE STRIPE API KEY"
with your actual publishable API key from Stripe. - Replace
"LAZY SERVER LINK"
with the endpoint URL of your published app that you received after pressing the "Test" button. - Replace
"PRICE_ID"
with the actual price ID you want to use for the transaction. You can find this in your Stripe dashboard under Products.
Here is the script you need to add to your WordPress page:
`
` After adding this script to your WordPress page, your custom Stripe payment page will be ready to accept payments.
Remember to test the payment flow thoroughly to ensure everything is working as expected before going live with your new payment page.
Technologies
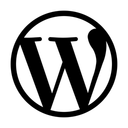
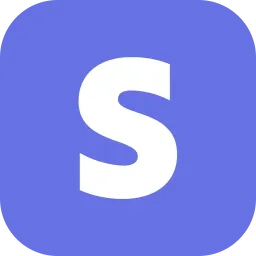
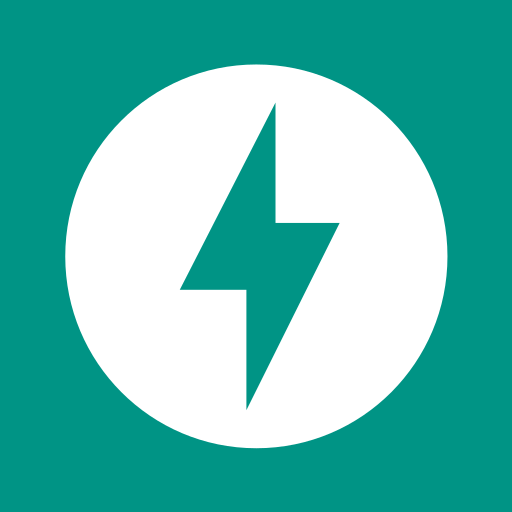