by Lazy Sloth
Gmail Organization Invitation API
import os
import smtplib
from email.mime.multipart import MIMEMultipart
from email.mime.text import MIMEText
from fastapi import FastAPI
from pydantic import BaseModel
import uvicorn
app = FastAPI()
class Invitation(BaseModel):
recipient_email: str
inviter_email: str
organization_name: str
invitation_link: str
@app.post("/send_invitation")
def send_invitation(invitation: Invitation):
# TODO: Replace with your Gmail account details
gmail_user = os.environ['GMAIL_USER']
gmail_password = os.environ['GMAIL_PASSWORD']
msg = MIMEMultipart('alternative')
Frequently Asked Questions
How can this Gmail Organization Invitation API benefit my business?
The Gmail Organization Invitation API can streamline your onboarding process by automating invitation emails. It allows you to quickly and efficiently invite new members to your organization, saving time and ensuring consistency in your communication. This API is particularly useful for businesses with frequent new member additions or those looking to scale their operations without increasing administrative overhead.
Can I customize the invitation email content using this API?
Yes, the Gmail Organization Invitation API allows for customization of the email content. The current template includes the inviter's email, organization name, and an invitation link. You can further customize the HTML content in the send_invitation
function to include additional information, branding elements, or personalized messages to suit your organization's needs.
What security measures are in place for this API?
The Gmail Organization Invitation API uses environment variables to store sensitive information like Gmail credentials, which is a security best practice. It also uses SMTP_SSL for secure communication with Gmail's servers. However, it's important to note that you should implement additional security measures such as API authentication and rate limiting when deploying this in a production environment.
How can I add error handling to the API?
To add error handling to the Gmail Organization Invitation API, you can modify the send_invitation
function to return appropriate status codes and messages. Here's an example:
```python from fastapi import FastAPI, HTTPException
@app.post("/send_invitation") def send_invitation(invitation: Invitation): try: # Existing email sending code here return {"status": "success", "message": "Invitation sent successfully"} except Exception as e: raise HTTPException(status_code=500, detail=f"Failed to send invitation: {str(e)}") ```
This modification will return a success message when the email is sent successfully and raise an HTTP exception with a 500 status code if an error occurs.
How can I test the Gmail Organization Invitation API locally?
To test the API locally, you can use the built-in Swagger UI provided by FastAPI. Here's how:
Created: | Last Updated:
Introduction to Gmail Organization Invitation API Template
Welcome to the step-by-step guide on how to use the Gmail Organization Invitation API Template. This template allows you to send personalized invitation emails to potential members of your organization. The email is sent through a Gmail account with 2FA enabled, and the API accepts various inputs to generate the invitation. Let's get started with how to set up and use this template effectively.
Start with this Template
To begin using this template, click on the "Start with this Template" button. This will initialize the template within the Lazy platform, allowing you to customize it according to your needs.
Environment Secrets
Before you can send out invitations, you need to set up your Gmail account details as environment secrets. This is crucial for the API to access your Gmail account and send emails. Here's how to set them up:
- Locate the Environment Secrets tab within the Lazy Builder interface.
- Create two new secrets: one named 'GMAIL_USER' and another named 'GMAIL_PASSWORD'.
- For 'GMAIL_USER', enter your Gmail email address.
- For 'GMAIL_PASSWORD', enter the password for your Gmail account. If you have 2FA enabled, you will need to generate an app-specific password to use here.
Remember, these credentials are sensitive, and Lazy will keep them secure as environment secrets.
Using the Test Button
Once you have set up your environment secrets, you can test the functionality of your app. Click the "Test" button to begin the deployment of the app and launch the Lazy CLI. If the code requires any user input, you will be prompted to provide it through the Lazy CLI.
After testing, if your app uses an API, Lazy will provide you with a dedicated server link to use the API. Since this template uses FastAPI, you will also receive a link to the API documentation, which can be very helpful for understanding the available endpoints and how to interact with them.
Sample API Request and Response
To send an invitation using the API, you will make a POST request to the "/send_invitation" endpoint with the required information. Here's a sample request:
POST /send_invitation
Content-Type: application/json
{ "recipient_email": "john.doe@example.com", "inviter_email": "jane.smith@yourcompany.com", "organization_name": "Your Organization", "invitation_link": "https://www.yourorganization.com/invite" }
Upon a successful request, you will receive a confirmation in the Lazy CLI that the email has been sent. If there is an issue, you will receive an error message detailing what went wrong.
By following these steps, you can easily set up and use the Gmail Organization Invitation API Template to send out personalized invitations to join your organization. Remember to handle your Gmail credentials securely and enjoy the convenience of automating your invitation process.
Template Benefits
-
Streamlined Onboarding Process: This API simplifies the process of inviting new members to an organization, automating the invitation process and reducing manual effort.
-
Scalability for Growing Organizations: As businesses expand, this template allows for easy scaling of the invitation process, handling a large number of invitations efficiently.
-
Customizable Communication: The template enables personalized invitations, improving the recipient's experience and increasing the likelihood of acceptance.
-
Integration Capabilities: Being an API, this template can be easily integrated into existing systems or applications, enhancing overall workflow efficiency.
-
Improved Security and Compliance: By using a standardized invitation process through a secure API, organizations can maintain better control over access management and ensure compliance with data protection regulations.
Technologies
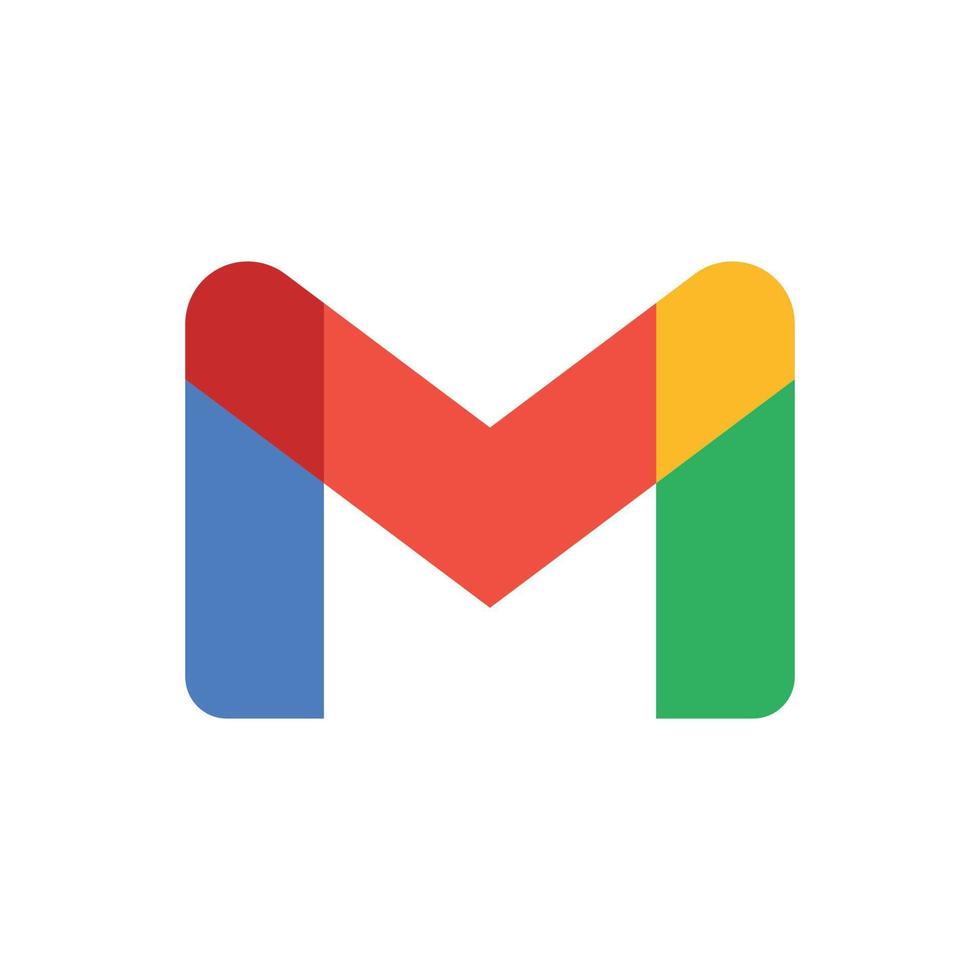