Forest Vision: Image Response App
import logging
from gunicorn.app.base import BaseApplication
from app_init import create_initialized_flask_app
# Flask app creation should be done by create_initialized_flask_app to avoid circular dependency problems.
app = create_initialized_flask_app()
# Setup logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
class StandaloneApplication(BaseApplication):
def __init__(self, app, options=None):
self.application = app
self.options = options or {}
super().__init__()
def load_config(self):
# Apply configuration to Gunicorn
for key, value in self.options.items():
if key in self.cfg.settings and value is not None:
self.cfg.set(key.lower(), value)
def load(self):
Frequently Asked Questions
What are some potential business applications for the Forest Vision: Image Response App?
The Forest Vision: Image Response App has several potential business applications: - Tourism: Hotels or travel agencies could use it to showcase local forest scenery to potential visitors. - Education: Schools or nature centers could utilize it as an interactive tool for teaching about different forest ecosystems. - Interior Design: Designers could use it to help clients visualize nature-themed decor or wallpapers. - Meditation Apps: It could be integrated into relaxation apps to provide calming forest imagery based on user preferences. - Environmental Awareness: NGOs could use it to raise awareness about different forest types and conservation efforts.
How can the Forest Vision app be monetized?
There are several ways to monetize the Forest Vision: Image Response App: - Freemium Model: Offer basic functionality for free, with premium features (e.g., higher resolution images, more diverse forest types) available for a subscription fee. - API Access: Provide API access to the image generation capabilities for other businesses to integrate into their own applications. - Advertising: Partner with eco-friendly brands or tourism companies to display relevant ads alongside the generated images. - Custom Enterprise Solutions: Offer tailored versions of the app for specific business needs, such as for real estate companies showcasing properties with forest views. - In-app Purchases: Allow users to buy high-quality prints of their favorite generated forest scenes.
What are the potential scalability challenges for the Forest Vision app as it grows?
As the Forest Vision: Image Response App scales, it may face several challenges: - Image Processing Load: Generating or retrieving images based on descriptions can be computationally intensive. The app might need to implement load balancing and potentially use cloud services for image processing. - Database Growth: As more users interact with the app, the database of descriptions, images, and user feedback will grow. Efficient database management and potentially sharding might be necessary. - API Rate Limiting: If offering API access, implementing proper rate limiting will be crucial to prevent abuse and ensure fair usage. - Content Moderation: As the user base grows, there may be a need for more robust content moderation to ensure appropriate use of the description feature. - Scaling Infrastructure: The app might need to transition from a simple Flask setup to a more distributed architecture to handle increased traffic.
How can I modify the Forest Vision app to support multiple languages?
To support multiple languages in the Forest Vision app, you can implement internationalization (i18n). Here's a basic example using Flask-Babel:
How can I implement image generation instead of using a static image in the Forest Vision app?
To implement dynamic image generation in the Forest Vision app, you could integrate with an AI image generation service like DALL-E or Stable Diffusion. Here's a basic example using the OpenAI API:
Created: | Last Updated:
Here's a step-by-step guide for using the Forest Vision: Image Response App template:
Introduction
The Forest Vision: Image Response App is a web application that generates static images based on user-input text descriptions, starting with predefined images of nature scenes. This template provides a simple interface for users to describe forest scenes and receive matching images.
Getting Started
To begin using this template:
- Click the "Start with this Template" button in the Lazy Builder interface.
Test the Application
Once you've started with the template:
- Click the "Test" button in the Lazy Builder interface.
- The Lazy CLI will initiate the deployment process.
Using the App
After the deployment is complete, you'll receive a dedicated server link to access the web application. Here's how to use it:
- Open the provided link in your web browser.
- You'll see a simple interface with a text area and a "Show Image" button.
- Enter a description of the forest scene you'd like to see in the text area.
- Click the "Show Image" button.
- The app will display a static forest image (currently using a placeholder image).
- You can provide feedback on the image by clicking the "Like" or "Dislike" buttons.
Understanding the Code Structure
The template includes several key components:
home.html
: The main page template._header.html
,_desktop_header.html
,_mobile_header.html
: Header components for different screen sizes.header.js
: JavaScript for handling the mobile menu.home.js
: JavaScript for handling form submission and feedback buttons.styles.css
: Custom styles for the application.main.py
: The main Python file that sets up the Flask application with Gunicorn.routes.py
: Defines the application routes.app_init.py
: Initializes the Flask app and database.database.py
: Handles database operations and migrations.
Customization Opportunities
To enhance the application, consider the following customizations:
- Implement actual image generation based on user descriptions.
- Store and analyze user feedback.
- Expand the database schema to include image metadata and user interactions.
- Add more pages or features to the application.
Remember, all changes and deployments are handled through the Lazy platform, so you don't need to worry about server setup or environment configuration.
Template Benefits
-
Rapid Prototyping for AI Image Generation: This template provides a quick starting point for businesses looking to develop or demonstrate AI-powered image generation capabilities, particularly for nature or forest-related imagery. It allows for fast proof-of-concept development without the need for complex AI integration initially.
-
Enhanced User Engagement: The interactive nature of the application, where users can input descriptions and receive immediate visual feedback, can significantly boost user engagement. This is particularly valuable for businesses in the tourism, environmental, or educational sectors looking to create immersive experiences.
-
Feedback Collection and Product Improvement: The built-in feedback mechanism (thumbs up/down) allows businesses to gather valuable user data. This can inform future improvements in image selection algorithms or AI model training, leading to better product offerings and increased customer satisfaction.
-
Scalable Web Application Architecture: The template demonstrates a well-structured, scalable web application using Flask, Gunicorn, and SQLAlchemy. This architecture can serve as a foundation for businesses looking to build robust web applications that can handle growth and increased user load.
-
Cross-Platform Compatibility: With its responsive design (mobile and desktop layouts) and use of modern web technologies, the template ensures that the application is accessible across various devices. This broad accessibility can help businesses reach a wider audience and improve overall user experience.
Technologies
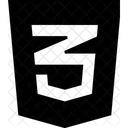
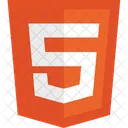
