by moonboy
Internal tool (web app) with Google sign in and team management
import logging
from gunicorn.app.base import BaseApplication
from app_init import create_initialized_flask_app
# Flask app creation should be done by create_initialized_flask_app to avoid circular dependency problems.
app = create_initialized_flask_app()
# Setup logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
class StandaloneApplication(BaseApplication):
def __init__(self, app, options=None):
self.application = app
self.options = options or {}
super().__init__()
def load_config(self):
# Apply configuration to Gunicorn
for key, value in self.options.items():
if key in self.cfg.settings and value is not None:
self.cfg.set(key.lower(), value)
def load(self):
Created: | Last Updated:
Internal Tool Template with Google Sign-in and Team Management
This template provides a ready-to-use internal tool with Google authentication and team management features. It's perfect for creating internal dashboards and tools where access needs to be restricted to specific team members.
Getting Started
- Click "Start with this Template" to begin using this template in the Lazy Builder interface
Test the Application
- Click the "Test" button in the Lazy Builder interface
- The application will deploy and provide you with a server link to access your internal tool
Using the Application
The internal tool comes with several built-in features:
- Google Sign-in Authentication: Users will be prompted to sign in with their Google account
- Team Management Dashboard: Access the team management page by clicking "Team" in the sidebar
- Admin Controls:
- Add individual admin users by email
- Allow entire email domains (e.g., "company.com")
- Block/unblock specific admin users
- View last login times for all users
- Delete admin access
The main dashboard (home page) is currently a placeholder that you can customize for your specific internal tool needs.
Managing Team Access
To manage who can access your internal tool:
- Sign in as the first admin
- Navigate to the Team page using the sidebar
- Add access in one of two ways:
- Click "Add New Admin" to grant access to individual email addresses
- Click "Add New Domain" to allow anyone with a specific email domain
Blocking and Unblocking Users
To manage user access:
- Click the "Block" button next to any user to prevent their access
- Click "Unblock" to restore access for blocked users
- Click "Delete" to completely remove admin access
The template provides a clean, responsive interface that works well on both desktop and mobile devices. The sidebar can be collapsed for more screen space, and all management functions are accessible through an intuitive interface.
Template Benefits
-
Secure Internal Access Control
Provides enterprise-grade authentication through Google Sign-In and granular access management, allowing organizations to strictly control who can access internal tools based on email domains or individual permissions. -
Rapid Internal Tool Development
Accelerates the development of company-specific web applications by providing a pre-built foundation with authentication, user management, and responsive UI components - reducing development time from weeks to days. -
Team Management Efficiency
Offers comprehensive team administration features including the ability to add/remove admins, block users, and manage domain-wide access - making it ideal for IT administrators to maintain organizational security and compliance. -
Professional User Experience
Delivers a polished, modern interface with responsive design, dark/light theme support, and mobile optimization - ensuring a consistent and professional experience across all devices for internal staff. -
Flexible Integration Foundation
Serves as a robust starting point for building custom internal applications, with a modular structure that makes it easy to add new features and integrate with existing business systems and workflows.
Technologies
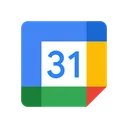
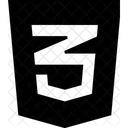
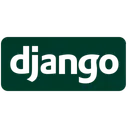
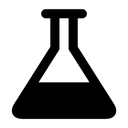
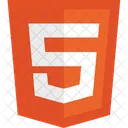
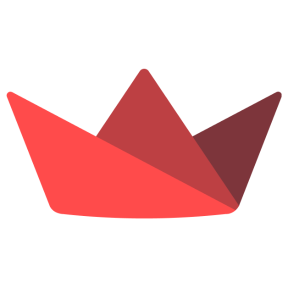

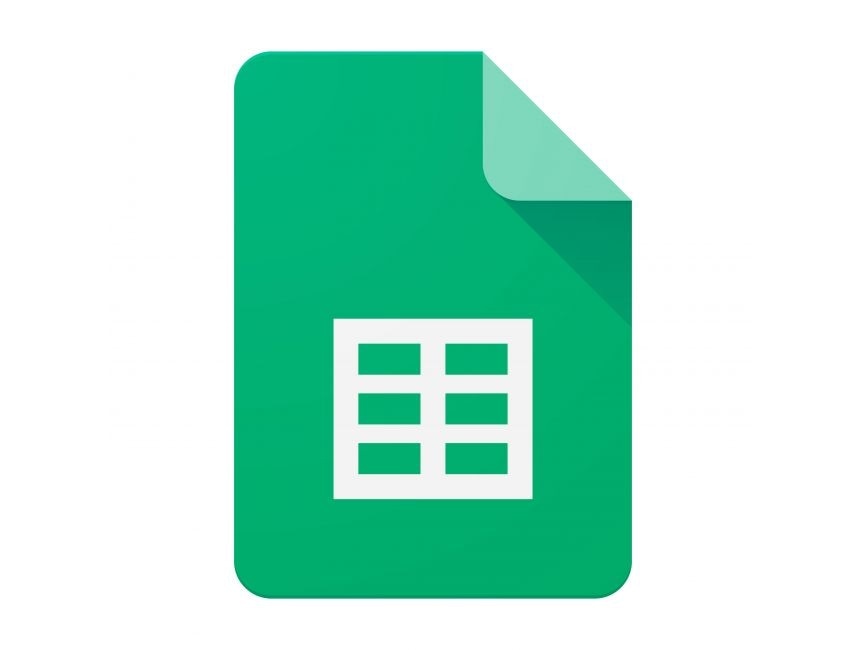

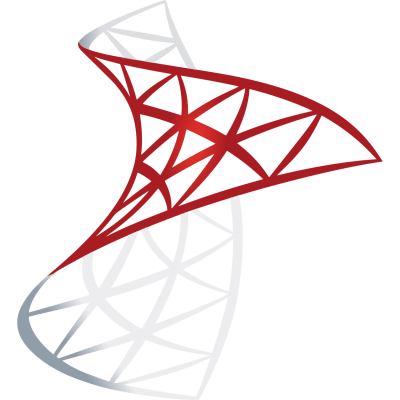