by davi
Web App Without Login
import logging
from gunicorn.app.base import BaseApplication
from app_init import create_initialized_flask_app
# Setup logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
# Flask app creation should be done by create_initialized_flask_app to avoid circular dependency problems.
app = create_initialized_flask_app()
class StandaloneApplication(BaseApplication):
def __init__(self, app, options=None):
self.application = app
self.options = options or {}
super().__init__()
def load_config(self):
# Apply configuration to Gunicorn
for key, value in self.options.items():
if key in self.cfg.settings and value is not None:
self.cfg.set(key.lower(), value)
def load(self):
Frequently Asked Questions
What types of businesses would benefit most from using the FALLBACK template?
The FALLBACK template is versatile and can benefit a wide range of businesses. It's particularly well-suited for startups, small to medium-sized enterprises, and freelancers who need a professional-looking website quickly. Its clean design and responsive layout make it ideal for service-based businesses, portfolios, and simple e-commerce sites. The template's flexibility allows it to be easily customized for various industries, from tech companies to creative agencies.
How can I customize the color scheme of the FALLBACK template to match my brand?
The FALLBACK template uses CSS variables for easy color customization. To change the color scheme, you can modify the :root
section in the styles.css
file. For example, to change the background color to a light blue and the text color to dark gray, you would update the CSS like this:
css
:root {
--bg-color: #e6f2ff;
--text-color: #333333;
--header-bg: #4a90e2;
--nav-link-bg: #3498db;
--nav-link-hover: #2980b9;
}
Remember to choose colors that complement your brand and ensure good contrast for readability.
Can the FALLBACK template handle dynamic content, and how would I integrate it with a content management system?
Yes, the FALLBACK template is built with Flask, which makes it easy to handle dynamic content. To integrate it with a content management system (CMS), you would typically:
How scalable is the FALLBACK template for growing businesses?
The FALLBACK template is designed with scalability in mind. It uses Flask, which can handle a significant amount of traffic when properly configured. The template also includes Gunicorn as a WSGI HTTP Server, which is known for its performance and ability to handle concurrent requests. As your business grows, you can easily add more routes, integrate with databases, and implement caching strategies. The use of Tailwind CSS also allows for rapid UI development as you add new features and pages to your site.
How can I add authentication to the FALLBACK template for secure areas of my website?
Adding authentication to the FALLBACK template is straightforward using Flask extensions like Flask-Login. Here's a basic example of how you could implement user login:
First, install Flask-Login:
pip install flask-login
Then, in your app_init.py
, add:
```python from flask_login import LoginManager
login_manager = LoginManager()
def create_initialized_flask_app(): # ... existing code ... login_manager.init_app(app) # ... rest of the function ... ```
Create a login route in routes.py
:
```python from flask import request, redirect, url_for from flask_login import login_user, login_required, logout_user from werkzeug.security import check_password_hash from models import User
@app.route('/login', methods=['GET', 'POST']) def login(): if request.method == 'POST': user = User.query.filter_by(username=request.form['username']).first() if user and check_password_hash(user.password, request.form['password']): login_user(user) return redirect(url_for('home_route')) return render_template('login.html')
@app.route('/logout') @login_required def logout(): logout_user() return redirect(url_for('home_route')) ```
This basic setup allows you to add @login_required
decorators to routes that need authentication in your FALLBACK template.
Created: | Last Updated:
Here's a step-by-step guide on how to use the provided Flask, HTML, JS, and Tailwind-based website template:
Introduction
This template provides a solid starting point for building a styled website using Flask, HTML, JavaScript, and Tailwind CSS. It includes a header, responsive navigation, and a basic structure for adding content. The template is pre-configured with Tailwind CSS and Flowbite for easy styling and component creation.
Getting Started
-
Click "Start with this Template" to begin using this template in the Lazy Builder interface.
-
Press the "Test" button to initiate the deployment of the app and launch the Lazy CLI.
Customizing the Template
To customize the template for your specific needs, follow these steps:
- Update the app title:
- Open the
_header.html
file - Locate the
<span class="app-title">App Name</span>
line -
Replace "App Name" with your desired application name
-
Modify the color scheme:
- Open the
styles.css
file - Find the
:root
section - Adjust the color variables to match your app's theme:
css
:root {
--bg-color: #your-background-color;
--text-color: #your-text-color;
--header-bg: #your-header-background-color;
--nav-link-bg: #your-nav-link-background-color;
--nav-link-hover: #your-nav-link-hover-color;
}
- Add content to the home page:
- Open the
home.html
file - Locate the
<div class="text-center py-10">
section -
Add your content within this div
-
Create additional pages:
- Duplicate the
home.html
file and rename it (e.g.,about.html
) - Update the content in the new file
- Add a new route in the
routes.py
file:
python
@app.route("/about")
def about_route():
return render_template("about.html")
- Update navigation:
- Open both
_mobile_header.html
and_desktop_header.html
- Add new navigation links to match your added pages
Using the App
After customizing the template and deploying it:
- You'll receive a server link through the Lazy builder CLI.
- Open this link in a web browser to view your website.
- Test the responsive design by resizing your browser window or using mobile device emulation in your browser's developer tools.
The template provides a clean, responsive layout that you can build upon. The mobile menu functionality is already implemented, allowing for a seamless experience on smaller screens.
Remember to leverage Tailwind CSS classes for styling your content, and refer to the Flowbite documentation for additional pre-built components you can use in your project.
Template Benefits
-
Rapid Development: This template provides a pre-configured Flask application with HTML, JavaScript, and Tailwind CSS, allowing developers to quickly start building web applications without spending time on initial setup.
-
Responsive Design: The template includes both mobile and desktop navigation components, ensuring a seamless user experience across different devices and screen sizes.
-
Scalability: With a modular structure (separate files for routes, models, and app initialization) and database integration using SQLAlchemy, the template supports easy scaling as the application grows.
-
Enhanced Security: The template includes basic security measures such as CSRF protection (Flask's built-in secret key) and database best practices (foreign key enforcement in SQLite).
-
Deployment Ready: The inclusion of Gunicorn configuration and a production-ready server setup makes it easy to deploy the application to various hosting platforms with minimal additional configuration.
Technologies
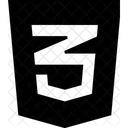
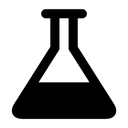
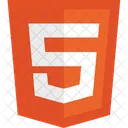

