by gal
Email AI Agent Dashboard
import logging
from gunicorn.app.base import BaseApplication
from app_init import create_initialized_flask_app
# Setup logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
# Flask app creation should be done by create_initialized_flask_app to avoid circular dependency problems.
app = create_initialized_flask_app()
class StandaloneApplication(BaseApplication):
def __init__(self, app, options=None):
self.application = app
self.options = options or {}
super().__init__()
def load_config(self):
# Apply configuration to Gunicorn
for key, value in self.options.items():
if key in self.cfg.settings and value is not None:
self.cfg.set(key.lower(), value)
def load(self):
Frequently Asked Questions
Time-saving: By automating email generation and follow-ups, your team can focus on high-value tasks while maintaining consistent outreach. The Email AI Agent Dashboard combines these features to streamline your email marketing efforts and improve overall efficiency and effectiveness. ### Q2: What types of businesses would benefit most from using the Email AI Agent Dashboard?
The Email AI Agent Dashboard is versatile and can benefit various types of businesses, but it's particularly valuable for:
Real estate agencies: For nurturing leads and keeping in touch with past clients. The Email AI Agent Dashboard's ability to personalize content, automate follow-ups, and manage multiple campaigns makes it a powerful tool for any business that relies on email communication for growth and customer engagement. ### Q3: How does the Email AI Agent Dashboard ensure email deliverability and compliance with anti-spam regulations?
The Email AI Agent Dashboard incorporates several features to maintain high deliverability rates and comply with anti-spam regulations:
Follow-up limits: Users can set a maximum number of follow-ups per contact to avoid excessive emailing. By implementing these measures, the Email AI Agent Dashboard helps businesses maintain a positive sender reputation and comply with regulations like CAN-SPAM and GDPR. ### Q4: How can I integrate the Email AI Agent Dashboard with my existing CRM system?
Integrating the Email AI Agent Dashboard with your CRM system would require some custom development. Here's a high-level approach to achieve this integration:
Use the existing `Contact` model to store the CRM data. Here's an example of how you might implement the API endpoint in the `routes.py` file: ```python from flask import request, jsonify from models import db, Contact, ContactCollection @app.route('/api/crm/contacts', methods=['POST']) def crm_contact_sync(): data = request.json collection_id = data.get('collection_id') contacts = data.get('contacts', []) collection = ContactCollection.query.get(collection_id) if not collection: return jsonify({'error': 'Collection not found'}), 404 for contact_data in contacts: existing_contact = Contact.query.filter_by(email=contact_data['email'], collection_id=collection_id).first() if existing_contact: # Update existing contact existing_contact.name = contact_data.get('name') existing_contact.company_name = contact_data.get('company_name') existing_contact.company_description = contact_data.get('company_description') existing_contact.additional_info = contact_data.get('additional_info') else: # Create new contact new_contact = Contact( collection_id=collection_id, email=contact_data['email'], name=contact_data.get('name'), company_name=contact_data.get('company_name'), company_description=contact_data.get('company_description'), additional_info=contact_data.get('additional_info') ) db.session.add(new_contact) db.session.commit() return jsonify({'message': 'Contacts synced successfully'}), 200 ``` This endpoint allows your CRM to send contact data to the Email AI Agent Dashboard, keeping your contact lists in sync. ### Q5: Can you explain how the LLM model selection works in the Email AI Agent Dashboard?
The Email AI Agent Dashboard allows users to select different Language Model (LLM) options for generating email content. This feature is implemented in the settings.html
template and the settings
route in routes.py
. Here's how it works:
Created: | Last Updated:
Here's a step-by-step guide for using the Email AI Agent Dashboard template:
Introduction
The Email AI Agent Dashboard template provides a comprehensive solution for managing an AI-powered email campaign system. This template includes features such as user authentication, email configuration, LLM model selection, and email scheduling. It's designed to help you set up and manage automated email campaigns using AI-generated content.
Getting Started
To begin using this template:
- Click the "Start with this Template" button in the Lazy Builder interface.
Setting Up the Dashboard
After starting with the template, follow these steps to set up and use the Email AI Agent Dashboard:
-
Click the "Test" button in the Lazy Builder interface to deploy the application.
-
Once deployed, you'll receive a server link through the Lazy CLI. This link will allow you to access the Email AI Agent Dashboard.
-
Open the provided link in your web browser to access the login page.
-
On the login page, enter your email address to log in. This template uses a simplified authentication system for demonstration purposes.
Configuring Email Settings
After logging in, you'll need to configure your email settings:
-
Navigate to the "Settings" page in the dashboard.
-
Fill in the following SMTP and IMAP details:
- SMTP Host (e.g., smtp.gmail.com)
- SMTP Port (e.g., 587)
- SMTP Username (your email address)
- SMTP Password (your email password or app-specific password)
- IMAP Host (e.g., imap.gmail.com)
- IMAP Port (e.g., 993)
- IMAP Username (your email address)
-
IMAP Password (your email password or app-specific password)
-
Choose whether to use TLS (recommended for security).
-
Select your default LLM model from the dropdown menu.
-
Click "Save Settings" to store your email configuration.
-
After saving, you can click "Test Email Integration" to verify your settings.
Creating Contact Collections
Before setting up campaigns, you need to create contact collections:
-
Go to the "Contacts" page in the dashboard.
-
Click on "Create a New Contact Collection" and provide a name and description.
-
After creating a collection, click "Manage Contacts" to add individual contacts to the collection.
-
For each contact, provide their name, email, company name, and any additional information.
Setting Up Campaigns
To create and manage email campaigns:
-
Navigate to the "Campaigns" page in the dashboard.
-
Click on "Create a New Campaign" and fill in the following details:
- Title
- Prompt (instructions for the AI to generate email content)
- LLM Model
- Follow-up frequency (in days)
- Whether to stop upon receiving a reply
-
Select the contact collection to use
-
Choose whether to activate the campaign immediately.
-
After creating a campaign, you can edit its details or test it by sending a sample email to yourself.
Using the Dashboard
The Email AI Agent Dashboard provides several features:
- Campaigns: View, create, edit, and manage your email campaigns.
- Contacts: Manage your contact collections and individual contacts.
- Settings: Configure your email settings and default LLM model.
- Templates: (This feature is mentioned in the code but not fully implemented in the provided template)
The system will automatically handle sending initial emails, checking for replies, and sending follow-up emails based on your campaign settings.
Important Notes
- This template uses a simplified authentication system. In a production environment, you should implement a more secure authentication method.
- The template includes a background process (
process_campaigns.py
) that handles checking for replies and sending follow-up emails. This process runs independently of the web interface. - Ensure that your email provider allows SMTP and IMAP access. For Gmail, you may need to use an app-specific password and enable less secure app access.
- The LLM models mentioned in the template (e.g., GPT-4o, Claude 3 Haiku) are placeholders. Ensure you have access to the AI models you plan to use.
By following these steps, you'll have a fully functional Email AI Agent Dashboard that can manage contacts, create AI-powered email campaigns, and handle follow-ups automatically.
Here are 5 key business benefits for this Email AI Agent Dashboard template:
Template Benefits
-
Automated Email Outreach: Enables businesses to set up and manage automated email campaigns powered by AI, allowing for personalized outreach at scale without manual effort for each message.
-
Intelligent Follow-ups: The system can automatically generate and send follow-up emails based on predefined schedules and rules, improving engagement rates and reducing the need for manual follow-up management.
-
Centralized Contact Management: Provides a centralized platform to organize and manage contact lists, making it easy to segment audiences and target specific groups for email campaigns.
-
Customizable AI Models: Allows users to select and configure different AI language models for email generation, enabling businesses to tailor the tone and style of communications to their brand and audience.
-
Performance Tracking: Includes features for tracking email campaign performance, such as monitoring reply rates and email thread progression, helping businesses optimize their outreach strategies over time.
Technologies
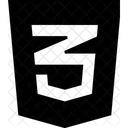
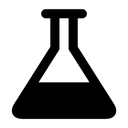
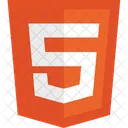