Discord Premium Access Bot
from shared import format_pass_type
from shared import calculate_end_date
import os
import discord
from discord import app_commands
from discord.ui import Button, View, Select
from abilities import apply_sqlite_migrations
from datetime import datetime, timedelta
from models import Base, engine, Purchase, Transaction, ServerSettings
from sqlalchemy.orm import Session
from sqlalchemy import func, and_
def generate_oauth_link(client_id):
base_url = "https://discord.com/api/oauth2/authorize"
redirect_uri = "http://localhost"
scope = "bot"
permissions = "8"
return f"{base_url}?client_id={client_id}&permissions={permissions}&scope={scope}"
from paypal_utils import PayPalAPI
class PaymentMethodView(View):
Frequently Asked Questions
Hobby or interest-based communities offering expert workshops or exclusive events. The bot's flexible pass types (1 day, 1 week, 1 month, and subscription) allow for versatile monetization strategies tailored to your community's needs. ### Q3: How does the financial tracking feature help in managing the business aspect of my Discord server?
The Discord Premium Access Bot includes robust financial tracking features that are crucial for managing the business side of your server. The /profit
and /profit-detailed
commands provide comprehensive analytics on your premium pass sales. These include:
Month-over-month comparisons to track growth. This data allows you to make informed decisions about pricing, promotions, and which pass types or payment methods to focus on. It also simplifies financial reporting and tax preparation for your Discord-based business. ### Q4: How can I customize the premium role assignment for my server using this bot?
The Discord Premium Access Bot allows for easy customization of premium role assignment using the /setrole
command. Here's an example of how to use it:
```python @tree.command(name="setrole") async def setrole(interaction: discord.Interaction, role: discord.Role): """Set the premium role for this server (Admin only)""" if not interaction.user.guild_permissions.administrator: await interaction.response.send_message("❌ You need administrator permissions to use this command!", ephemeral=True) return
# Check if the bot's role is lower than the premium role
if interaction.guild.me.top_role <= role:
await interaction.response.send_message("❌ The premium role must be lower than my highest role!", ephemeral=True)
return
with Session(engine) as session:
settings = session.query(ServerSettings).filter_by(server_id=str(interaction.guild.id)).first()
if settings:
settings.premium_role_id = str(role.id)
else:
settings = ServerSettings(server_id=str(interaction.guild.id), premium_role_id=str(role.id))
session.add(settings)
session.commit()
await interaction.response.send_message(f"✅ Premium role has been set to {role.mention}", ephemeral=True)
```
This command allows server administrators to set any existing role as the premium role. The bot will automatically assign this role to users when they purchase a premium pass.
Q5: How does the bot handle different payment verification processes for various payment methods?
A: The Discord Premium Access Bot implements separate verification processes for different payment methods to ensure secure and accurate premium access activation. Here's an example of how it handles CashApp verification:
```python @tree.command(name="verify-cashapp") async def verify_cashapp(interaction: discord.Interaction, payment_id: str): """Submit your CashApp payment ID to verify your payment""" with Session(engine) as session: transaction = session.query(Transaction).join(Purchase).filter( and_( Purchase.user_id == str(interaction.user.id), Transaction.payment_method == 'cashapp', Purchase.is_active == False ) ).order_by(Transaction.transaction_date.desc()).first()
if not transaction:
await interaction.response.send_message(
"❌ No pending CashApp payment found. Please start a new purchase using `/purchase`",
ephemeral=True
)
return
transaction.cashapp_payment_id = payment_id
transaction.purchase.is_active = True
session.commit()
# Add premium role if configured
settings = session.query(ServerSettings).filter_by(server_id=str(interaction.guild.id)).first()
if settings and settings.premium_role_id:
try:
role = interaction.guild.get_role(int(settings.premium_role_id))
if role:
await interaction.user.add_roles(role)
except Exception as e:
print(f"Error adding premium role: {str(e)}")
await interaction.response.send_message("✅ Your CashApp payment has been verified and your premium access is now active!", ephemeral=True)
```
This command allows users to submit their CashApp payment ID, which the bot then uses to verify and activate their premium access. Similar verification processes are implemented for other payment methods, ensuring a secure and user-friendly experience across all supported payment options.
Created: | Last Updated:
Here's a step-by-step guide for using the Discord Premium Access Bot template:
Introduction
This template provides a Discord bot for managing premium memberships with multiple payment options, automatic role assignment, financial tracking, and user management features. The bot allows server administrators to set up premium passes, handle various payment methods, and track revenue.
Getting Started
To begin using this template:
- Click the "Start with this Template" button in the Lazy Builder interface.
Initial Setup
Before running the bot, you need to set up some environment secrets:
- Go to the Discord Developer Portal and create a new application:
- Create a bot for your application
- Copy the Bot Token
-
Copy the Client ID
-
Set up the following environment secrets in the Lazy Builder's Environment Secrets tab:
BOT_TOKEN
: Paste your Discord Bot TokenCLIENT_ID
: Paste your Discord Application Client IDCASHAPP_HANDLE
: Your CashApp handle (e.g., $YourCashAppName)VENMO_HANDLE
: Your Venmo handle (e.g., @YourVenmoName)BITCOIN_WALLET
: Your Bitcoin wallet addressETH_WALLET
: Your Ethereum wallet addressLTC_WALLET
: Your Litecoin wallet addressPAYPAL_CLIENT_ID
: Your PayPal API Client IDPAYPAL_CLIENT_SECRET
: Your PayPal API Client Secret
To get the PayPal API credentials: 1. Go to the PayPal Developer Dashboard (developer.paypal.com) 2. Log in or create an account 3. Navigate to "My Apps & Credentials" 4. Under "REST API apps," click "Create App" 5. Give your app a name and click "Create App" 6. In the resulting page, you'll find your Client ID and Secret
Test
After setting up the environment secrets:
- Click the "Test" button in the Lazy Builder interface.
- The bot will start, and you'll see a message with an OAuth link to invite the bot to your Discord server.
Using the Bot
Once the bot is invited to your server:
- Use the
/setrole
command to set the premium role for your server (admin only). - Members can use
/purchase
to start the premium pass purchase process. - After payment, members use the appropriate verification command (e.g.,
/verify-cashapp
,/verify-btc
) to activate their premium access. - Members can check their status with
/status
or/time-left
. - Admins can view profit reports using
/profit
and/profit-detailed
.
Integrating the Bot
To fully integrate the bot into your Discord server:
- Set up a premium channel or category in your Discord server.
- Use Discord's built-in permissions to restrict access to these channels to users with the premium role you set using
/setrole
. - Create a channel for purchase instructions and pin a message with the
/purchase
command information. - Consider setting up webhooks in a private channel to monitor transactions and member activity.
By following these steps, you'll have a fully functional Premium Access Bot for your Discord server, allowing you to manage premium memberships and process payments through various methods.
Template Benefits
-
Monetization of Discord Communities: This bot enables server owners to create a tiered membership system, allowing them to monetize their Discord communities by offering premium access to exclusive content or features.
-
Flexible Payment Options: By supporting multiple payment methods (PayPal, CashApp, Venmo, and cryptocurrencies), the bot caters to a wider audience and increases the likelihood of successful transactions, potentially boosting revenue.
-
Automated Membership Management: The bot handles the entire process of purchasing, verifying payments, and granting access automatically, reducing administrative overhead and ensuring a smooth user experience.
-
Financial Tracking and Analytics: With built-in profit tracking and detailed analytics, server owners can gain valuable insights into their revenue streams, helping them make data-driven decisions to optimize their pricing and offerings.
-
Customizable Role Integration: The ability to automatically assign and remove roles based on premium status allows for seamless integration with existing server structures, enhancing the value proposition of premium memberships.
Technologies
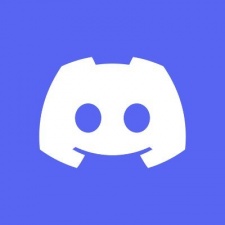
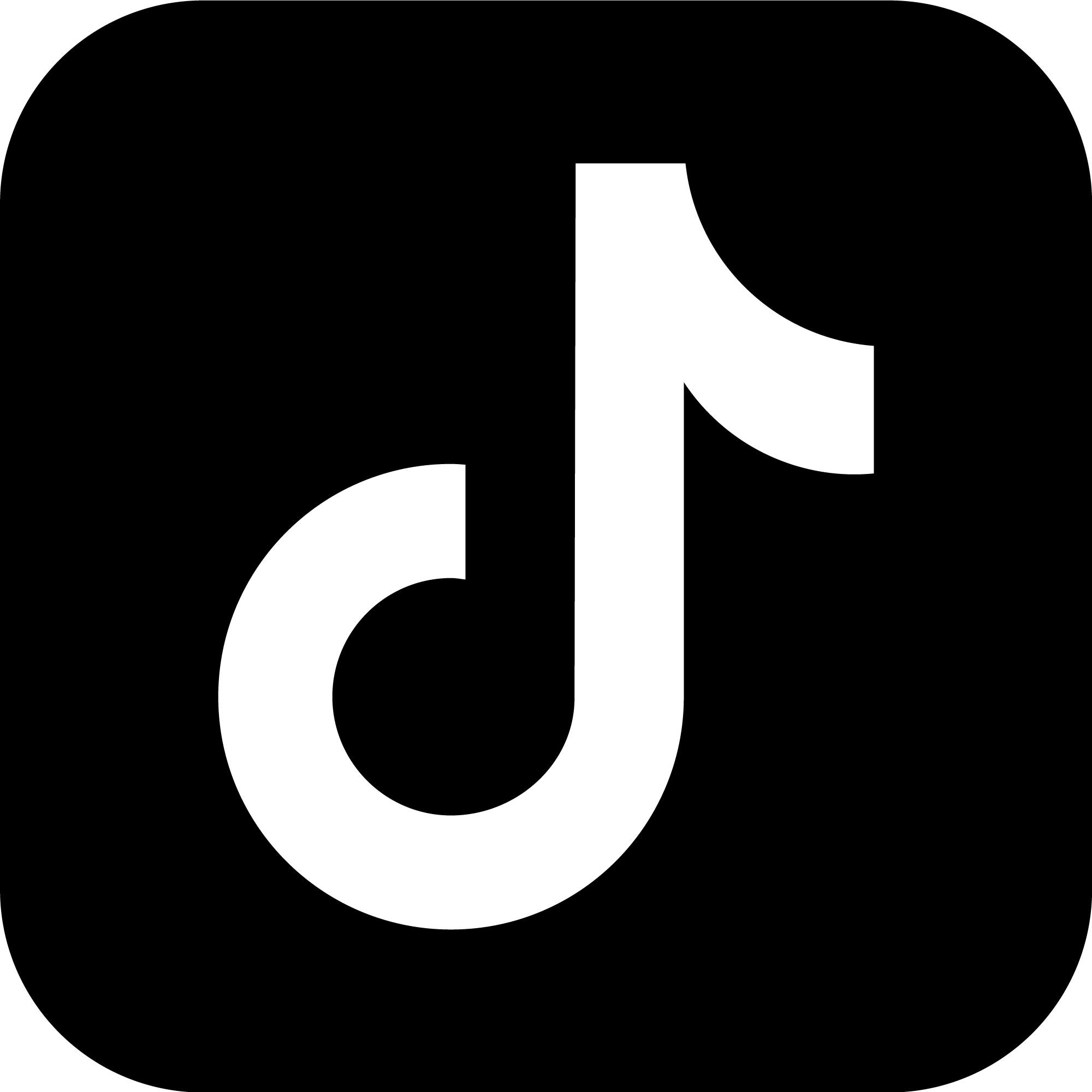
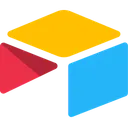
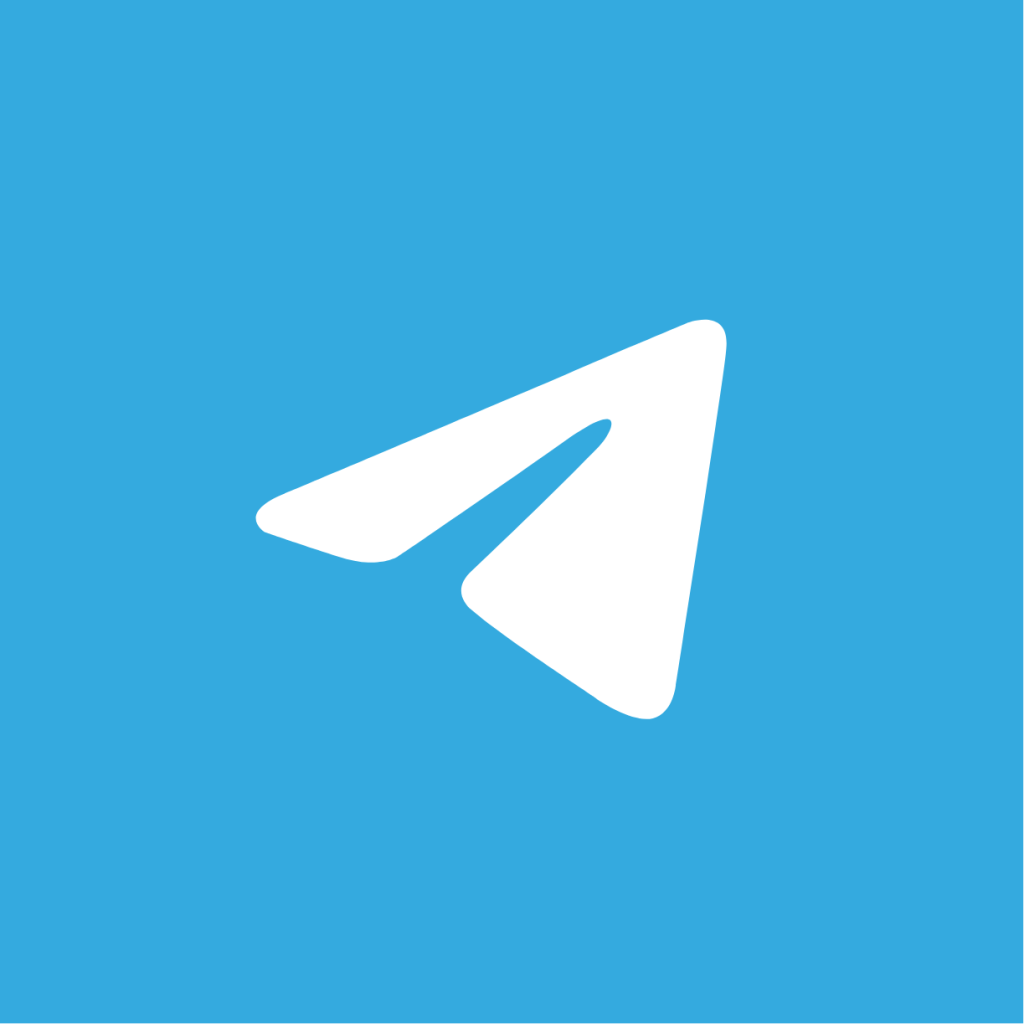
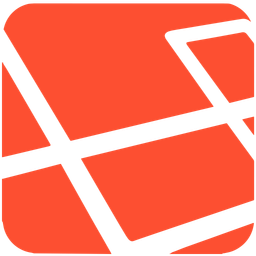
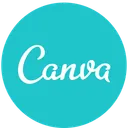
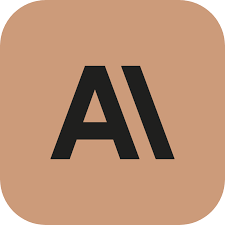
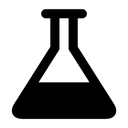
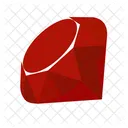
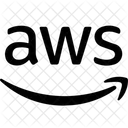
