Claude AI Quickstart Chat API
import logging
import os
from fastapi import FastAPI, HTTPException
from pydantic import BaseModel
from typing import Optional
import anthropic
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
app = FastAPI()
client = anthropic.Anthropic(
api_key=os.environ.get("ANTHROPIC_API_KEY")
)
class ChatRequest(BaseModel):
system_prompt: Optional[str] = "You are a helpful assistant. Respond to the following question/statement."
user_prompt: str
model: Optional[str] = "claude-3-opus-20240229"
temperature: Optional[float] = 1.0
max_tokens: Optional[int] = 1000
@app.post("/ask-claude/")
Frequently Asked Questions
What are some potential business applications for this Claude 3 Quickstart Chat API?
The Claude 3 Quickstart Chat API offers numerous business applications across various industries. Some potential use cases include: - Customer support chatbots that can handle complex queries - Content generation for marketing materials - Automated research assistants for data analysis and report writing - Personalized product recommendations in e-commerce - Language translation services for international businesses
By leveraging Claude 3's advanced capabilities, businesses can quickly integrate AI-powered solutions into their existing workflows and applications.
How can this API improve productivity in a business setting?
The Claude 3 Quickstart Chat API can significantly boost productivity by: - Automating repetitive tasks and queries - Providing instant access to vast amounts of information - Offering 24/7 availability for customer inquiries - Assisting employees with research and data analysis - Generating draft content for various business needs
By implementing this API, businesses can free up human resources for more complex tasks while ensuring consistent and rapid responses to routine inquiries.
What advantages does using Claude 3 through this API offer compared to other AI solutions?
The Claude 3 Quickstart Chat API provides several advantages: - Access to one of the most advanced AI models available (Claude 3 Opus) - Easy integration with existing applications and systems - Customizable system prompts for tailored responses - Adjustable parameters like temperature and max tokens for fine-tuned outputs - Scalability to handle varying workloads
These features make the Claude 3 Quickstart Chat API a versatile and powerful tool for businesses looking to leverage cutting-edge AI capabilities.
How can I customize the system prompt in the API request?
To customize the system prompt in your API request, you can modify the system_prompt
field when sending a POST request to the /ask-claude/
endpoint. Here's an example using Python's requests
library:
```python import requests import json
url = "http://your-api-url/ask-claude/" headers = {"Content-Type": "application/json"} data = { "system_prompt": "You are a financial advisor. Provide investment advice based on the following question.", "user_prompt": "What are some low-risk investment options for beginners?", "model": "claude-3-opus-20240229", "temperature": 0.7, "max_tokens": 500 }
response = requests.post(url, headers=headers, data=json.dumps(data)) print(response.json()) ```
By changing the system_prompt
value, you can guide Claude 3 to respond in different contexts or with specific expertise.
How can I handle errors or exceptions when using the Claude 3 Quickstart Chat API?
The API includes error handling that returns a 500 status code with a generic error message for any exceptions. To implement more detailed error handling in your application, you can wrap the API call in a try-except block and check the status code. Here's an example:
```python import requests import json
def chat_with_claude(user_prompt): url = "http://your-api-url/ask-claude/" headers = {"Content-Type": "application/json"} data = { "user_prompt": user_prompt }
try:
response = requests.post(url, headers=headers, data=json.dumps(data))
response.raise_for_status() # Raises an HTTPError for bad responses
return response.json()['message']
except requests.exceptions.HTTPError as http_err:
print(f"HTTP error occurred: {http_err}")
except requests.exceptions.RequestException as err:
print(f"An error occurred: {err}")
except json.JSONDecodeError:
print("Failed to decode JSON response")
return None
```
This approach allows you to handle different types of errors that may occur when using the Claude 3 Quickstart Chat API, providing more informative feedback to your users or logging for troubleshooting.
Created: | Last Updated:
Introduction to the Template
Welcome to the Claude 3 Quickstart Chat API template! This template allows you to integrate the largest anthropic model, Claude Opus, into any application you're building with just your API key. The /ask-claude
endpoint enables you to send requests to Claude 3 and receive responses, making it a powerful tool for various applications.
Getting Started
To get started with this template, click Start with this Template.
Test
After starting with the template, press the Test button. This will begin the deployment of the app and launch the Lazy CLI. The Lazy CLI will guide you through any required user input.
Entering Input
The code does not require any user input through the CLI, so you can skip this section.
Using the App
Once the app is deployed, you can use the /ask-claude
endpoint to communicate with the AI. Here’s how you can interact with the API:
- Endpoint:
/ask-claude/
- Method: POST
-
Request Body:
json { "system_prompt": "You are a helpful assistant. Respond to the following question/statement.", "user_prompt": "Your question or statement here", "model": "claude-3-opus-20240229", "temperature": 1.0, "max_tokens": 1000 }
-
Sample Request:
bash curl -X POST "http://<your-server-link>/ask-claude/" -H "Content-Type: application/json" -d '{ "system_prompt": "You are a helpful assistant. Respond to the following question/statement.", "user_prompt": "What is the capital of France?", "model": "claude-3-opus-20240229", "temperature": 1.0, "max_tokens": 1000 }'
-
Sample Response:
json { "message": "The capital of France is Paris." }
Integrating the App
To integrate this app into your service or frontend, follow these steps:
- Get the Server Link: After pressing the Test button, the Lazy CLI will provide you with a dedicated server link to use the API.
- API Documentation: You will also receive a link to the FastAPI documentation, which you can use to explore and test the endpoints.
Example Integration
If you are integrating this API into another service, you might need to add the server link and endpoints to your external tool. Here’s an example of how to do this:
- External Tool: Postman
- Open Postman and create a new request.
- Set the request method to POST.
- Enter the server link followed by
/ask-claude/
in the request URL. - In the Body tab, select
raw
and set the type toJSON
. - Paste the sample request body into the input field.
- Click Send to test the request and view the response.
By following these steps, you can easily integrate the Claude 3 Quickstart Chat API into your application and start leveraging the power of the Claude Opus model.
Template Benefits
-
Rapid AI Integration: This template allows businesses to quickly integrate Claude 3, Anthropic's most advanced AI model, into their existing applications or services with minimal setup, accelerating time-to-market for AI-enhanced products.
-
Scalable Microservice Architecture: Built on FastAPI, this template provides a foundation for creating scalable, high-performance microservices that can handle a large number of AI requests efficiently, supporting business growth and increased demand.
-
Customizable AI Interactions: The template offers flexibility in customizing system prompts, user prompts, and model parameters, allowing businesses to tailor AI responses to their specific use cases and brand voice.
-
Error Handling and Logging: With built-in error handling and logging, the template helps businesses maintain robust and reliable AI services, reducing downtime and improving the overall user experience.
-
API-First Design: The template's API-first approach enables easy integration with various front-end applications, mobile apps, or other services, fostering innovation and allowing businesses to leverage AI capabilities across multiple platforms and products.
Technologies
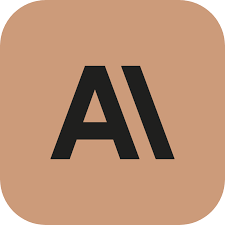
