Browser Notification System
import logging
from gunicorn.app.base import BaseApplication
from app_init import create_initialized_flask_app
# Flask app creation should be done by create_initialized_flask_app to avoid circular dependency problems.
app = create_initialized_flask_app()
# Setup logging
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
class StandaloneApplication(BaseApplication):
def __init__(self, app, options=None):
self.application = app
self.options = options or {}
super().__init__()
def load_config(self):
# Apply configuration to Gunicorn
for key, value in self.options.items():
if key in self.cfg.settings and value is not None:
self.cfg.set(key.lower(), value)
def load(self):
Frequently Asked Questions
How can this Browser Notification System be beneficial for businesses?
The Browser Notification System can be highly beneficial for businesses in several ways: - Customer Engagement: It allows companies to send real-time updates, promotions, or important information directly to users' browsers, even when they're not actively on the website. - Increased Traffic: By sending timely notifications, businesses can encourage users to return to their site, potentially increasing traffic and conversions. - Improved User Experience: For services that require timely updates (e.g., delivery status, appointment reminders), the Browser Notification System can significantly enhance the user experience by providing instant information.
What industries could benefit most from implementing this Browser Notification System?
Several industries could greatly benefit from this system: - E-commerce: Notifying customers about order status, new arrivals, or flash sales. - News and Media: Alerting readers to breaking news or important updates. - Social Media Platforms: Informing users about new messages, comments, or interactions. - Financial Services: Sending alerts for account activities, market updates, or bill reminders. - Healthcare: Reminding patients about appointments or medication schedules.
How can the Browser Notification System be customized for different business needs?
The Browser Notification System is highly customizable to suit various business needs: - Content Customization: The notification message and icon can be tailored to match the brand and convey specific information. - Timing and Frequency: Businesses can adjust when and how often notifications are sent based on user preferences and engagement patterns. - Integration: The system can be integrated with backend services to automate notifications based on specific triggers or events relevant to the business.
How can I modify the Browser Notification System to include custom icons for my business?
To include custom icons in the Browser Notification System, you can modify the sendNotification
function in the home.js
file. Here's an example of how you can do this:
javascript
function sendNotification() {
if ('Notification' in window) {
if (Notification.permission === 'granted') {
new Notification('Your Business Name', {
body: 'Your custom notification message.',
icon: '/path/to/your/custom-icon.png' // Replace with your icon path
});
} else {
alert('Please allow notifications in your browser settings.');
}
} else {
alert('Your browser does not support notifications.');
}
}
Replace 'Your Business Name'
, 'Your custom notification message.'
, and '/path/to/your/custom-icon.png'
with your specific business details and icon path.
Can the Browser Notification System be extended to support push notifications for mobile devices?
Yes, the Browser Notification System can be extended to support push notifications for mobile devices. This would require additional setup and integration with a service like Firebase Cloud Messaging (FCM). Here's a basic example of how you might modify the sendNotification
function to support both web and mobile push notifications:
javascript
function sendNotification() {
if ('serviceWorker' in navigator && 'PushManager' in window) {
navigator.serviceWorker.ready.then(function(registration) {
registration.pushManager.subscribe({userVisibleOnly: true})
.then(function(subscription) {
// Send subscription to your server
fetch('/api/push-subscription', {
method: 'POST',
body: JSON.stringify(subscription),
headers: {
'Content-Type': 'application/json'
}
});
});
});
} else if ('Notification' in window) {
// Fallback to browser notifications
if (Notification.permission === 'granted') {
new Notification('Hello!', {
body: 'This is a test notification.',
icon: 'https://via.placeholder.com/150'
});
} else {
Notification.requestPermission();
}
} else {
alert('Your browser does not support notifications.');
}
}
This code checks for service worker and push manager support (common in mobile browsers) before falling back to the standard web notifications. You would also need to set up a server-side component to handle sending push notifications to mobile devices.
Created: | Last Updated:
Here's a step-by-step guide on how to use the Browser Notification System template:
Introduction
This template provides a basic browser notification system that allows users to send test notifications with permission checks and error handling. It includes a simple web interface with a button to trigger notifications.
Getting Started
- Click "Start with this Template" to begin using the Browser Notification System template in the Lazy Builder interface.
Test the Application
-
Press the "Test" button in the Lazy Builder interface to deploy and run the application.
-
Once the deployment is complete, you will receive a dedicated server link to access the web interface.
Using the App
-
Open the provided server link in your web browser. You should see a simple page with a heading and a button labeled "Send Notification".
-
When you first visit the page, your browser may prompt you to allow notifications. Grant permission to enable the notification functionality.
-
Click the "Send Notification" button to trigger a test notification. If permissions are granted, you should see a notification appear with the message "Hello! This is a test notification."
-
If notifications are not allowed or not supported by your browser, you will see an alert message instead.
Customizing the Notification
To customize the notification content, you can modify the sendNotification
function in the home.js
file:
javascript
function sendNotification() {
if ('Notification' in window) {
if (Notification.permission === 'granted') {
new Notification('Your Custom Title', {
body: 'Your custom notification message.',
icon: 'path/to/your/icon.png' // Optional: Add a custom icon
});
} else {
alert('Please allow notifications in your browser settings.');
}
} else {
alert('Your browser does not support notifications.');
}
}
Integrating into Your Own Application
To integrate this notification system into your own application:
-
Copy the relevant JavaScript code from
home.js
into your application's JavaScript file. -
Ensure you have a button or trigger in your HTML that calls the
sendNotification()
function when clicked. -
Customize the notification content and styling to fit your application's needs.
By following these steps, you'll have a functional browser notification system that you can easily integrate into your web applications.
Template Benefits
-
Enhanced User Engagement: This template provides a simple way to implement browser notifications, allowing businesses to keep users engaged and informed even when they're not actively browsing the website. This can lead to increased user retention and more frequent site visits.
-
Improved Communication Channel: By implementing browser notifications, businesses gain an additional communication channel with their users. This can be particularly useful for time-sensitive information, updates, or promotional offers, potentially increasing conversion rates and customer satisfaction.
-
Cross-Platform Compatibility: The template includes both mobile and desktop navigation components, ensuring a consistent user experience across different devices. This responsive design approach can help businesses reach a wider audience and improve overall user satisfaction.
-
Easy Integration and Customization: With a modular structure and clear separation of concerns (HTML, CSS, JavaScript), this template can be easily integrated into existing projects or customized to fit specific business needs, potentially reducing development time and costs.
-
Scalable Backend Architecture: The inclusion of a Flask backend with database migration support and Gunicorn server configuration provides a solid foundation for building scalable web applications. This can help businesses handle growth and increased traffic without major architectural changes.
Technologies
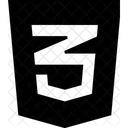
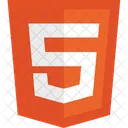