by moonboy
Telegram Mini App Example
from flask import Flask, render_template, url_for, redirect
import os
import logging
import threading
from telegram import Update, WebAppInfo
import telegram
from telegram.ext import Updater, CommandHandler, MessageHandler, CallbackContext, Filters
from web_app_routes import app
from utils import get_bot_username
TELEGRAM_API_TOKEN = os.getenv('TELEGRAM_API_TOKEN')
logger = logging.getLogger(__name__)
logging.basicConfig(level=logging.INFO, format='%(asctime)s - %(name)s - %(levelname)s - %(message)s')
#DO NOT CHANGE THIS
webapp_url = f"{app.config['BASE_URL']}"
def start(update: Update, context: CallbackContext) -> None:
update.message.reply_text(
"Hi! I'm a Telegram Web App. Click the link below to open my simple web app!",
reply_markup=telegram.InlineKeyboardMarkup([[
telegram.InlineKeyboardButton("Open Web App", web_app=WebAppInfo(url=webapp_url))
]])
Created: | Last Updated:
Introduction to the Telegram Mini App Template
This template provides a foundation for creating a Telegram Mini App, which is a web application that runs inside the Telegram messenger. The template includes a basic structure with a home page and profile view, along with Telegram user authentication.
Getting Started
- Click "Start with this Template" to begin using this template in the Lazy Builder
Initial Setup
You'll need to set up your Telegram bot first:
- Go to @BotFather on Telegram
- Send the
/newbot
command - Follow the prompts to create your bot
- Copy the API token provided by BotFather
- In the Lazy Builder, go to the Environment Secrets tab
- Add a new secret with key
TELEGRAM_API_TOKEN
and paste your bot token as the value - Add another secret with key
TESTER_PASSWORD
and set any secure password you want to use for testing
Test the Application
- Click the "Test" button in the Lazy Builder
- Wait for the application to deploy
- The CLI will provide you with your bot's username and a link to start chatting with it
Using the Mini App
Once deployed, you can interact with your Mini App in two ways:
- Through Telegram:
- Open your bot in Telegram
- Send the
/start
command -
Click the "Open Web App" button that appears
-
For testing outside Telegram:
- Visit the app URL provided by the Lazy Builder
- Use the tester password you set earlier to access the app
The Mini App includes: * A home page with customizable content * A profile page showing the user's Telegram information * Bottom navigation for easy access to both pages
Customizing the Template
To modify the Mini App, focus on editing the index.html
file. The main content of your app should go in the body section:
```html
```
Note: The template automatically handles the base URL configuration through Flask, so you don't need to set it manually.
To change the app name, modify the title in both index.html
and other template files where it appears.
Template Benefits
- Rapid Customer Engagement Platform
- Instantly deploy interactive customer-facing applications within Telegram
- Leverage Telegram's massive user base without requiring customers to download separate apps
-
Create seamless engagement experiences where customers already spend their time
-
Cost-Effective Business Solution
- Eliminate the need for expensive native mobile app development
- Reduce maintenance costs by maintaining a single web-based application
-
Minimize infrastructure expenses through lightweight implementation
-
Secure User Authentication System
- Built-in Telegram authentication removes the need for complex login systems
- Leverage Telegram's security features for user verification
-
Maintain user privacy while accessing profile information securely
-
Flexible Testing Environment
- Includes a tester mode for development and debugging
- Allows testing without Telegram integration during development
-
Supports smooth transition between testing and production environments
-
Scalable Business Integration Framework
- Easy integration with existing business systems through Flask backend
- Extensible architecture for adding custom features and functionality
- Support for database operations and API integrations for business growth
Technologies

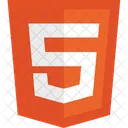

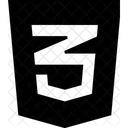
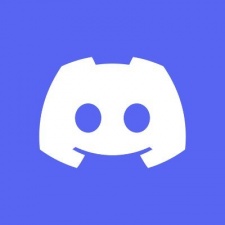
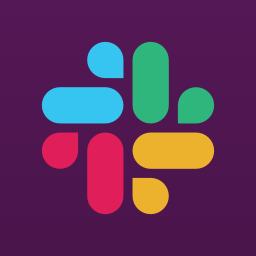
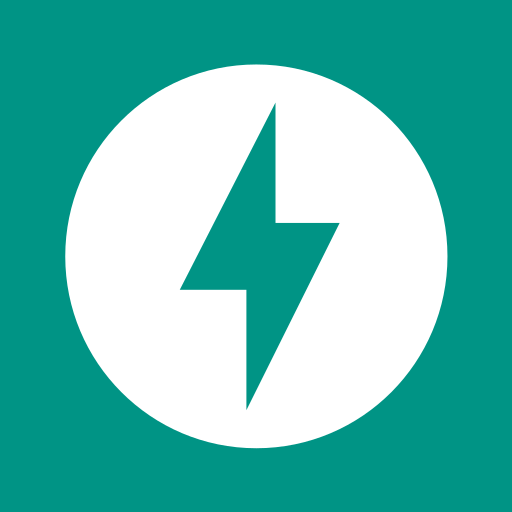
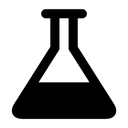
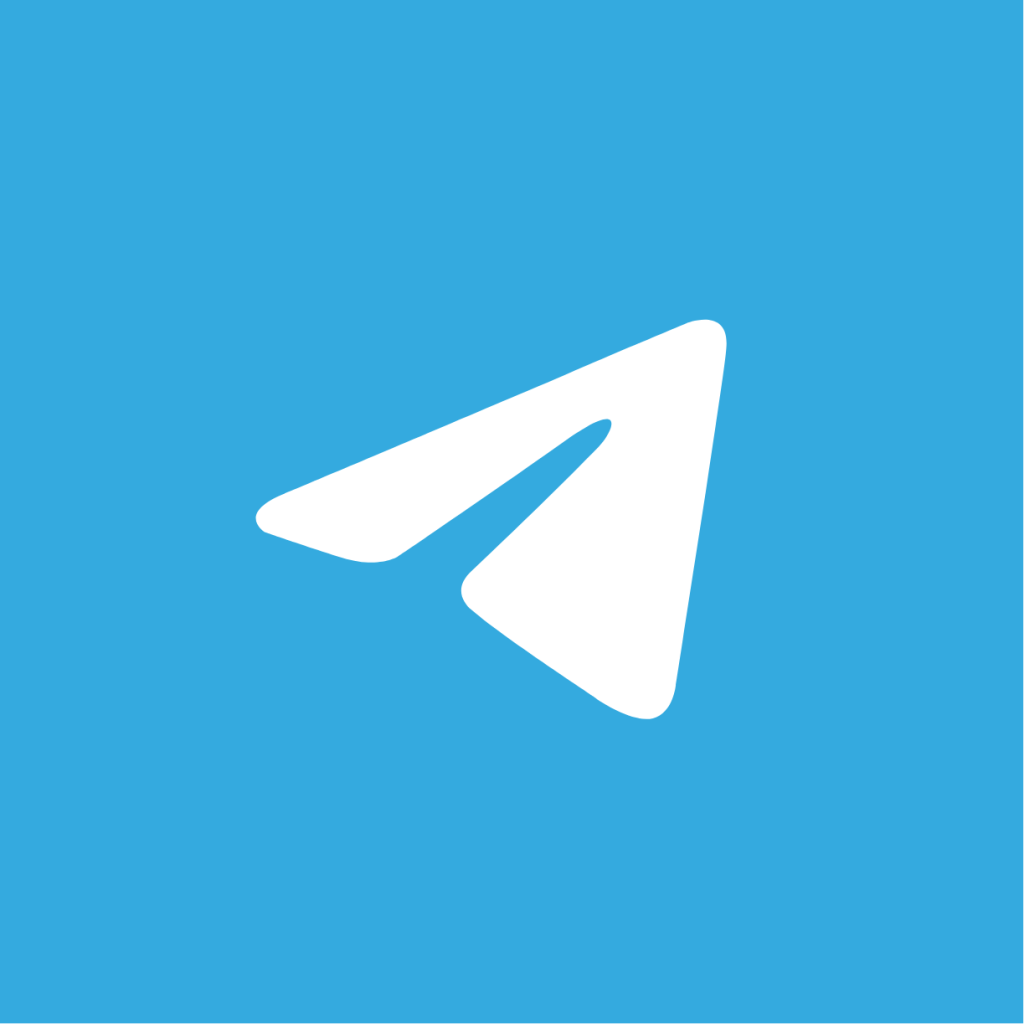
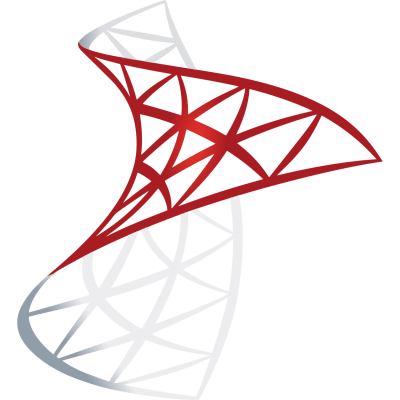