I have worked with many frameworks and libraries when developing web applications to make them visually pleasing and robust. For creating HTML templates in the Laravel ecosystem, I've found it easy and convenient to use.
Why Use HTML Templates in Laravel?
HTML templates are already-built web page formats that can be used as a base for creating your Laravel builds. Laravel developers can find many templates available to cover all the components that are used regularly. Using HTML templates, you can take advantage of several important advantages:
Fast Prototyping: HTML templates come with a rich set of pre-designed UI components and layouts that allow you to quickly prototype and put together the user interface of your application. This way, your workflow is cleaner, and you can dedicate time and effort towards just the core features and functionality of your Laravel app as opposed to writing custom HTML and CSS.
Responsive Design: A lot of HTML templates are mobile-first, giving you a powerful set of responsive utilities and grid systems. It enables developers to easily create fluid layouts that adjust themselves naturally according to screen sizes and types of devices, allowing for a consistent user experience across platforms.
Uniform Styling: Utilizing a pre-built HTML template allows you to achieve a consistent visual design across your Laravel application. It aids in creating a unified brand image and improves the user experience overall.
Comprehensive Documentation and Community: The HTML template ecosystem has a vast and active developer community, offering extensive documentation, tutorials, and resources. It simplifies the process of getting HTML templates to work with Laravel and finding solutions for common problems.
Laravel is a powerful web framework that can handle the backend functionality of web applications, while HTML templates are used to render the frontend presentation of the application.
Laravel is a PHP framework that provides an elegant syntax, built-in features, and functionalities for creating scalable web applications, while HTML templates enable you to design the user interface of your application in a user-friendly way. Laravel, owing to its rich features and MVC architecture, gives users the backend logic and structure, HTML templates give you a ready-made and responsive user interface that can be easily customized and plugged into your Laravel application.
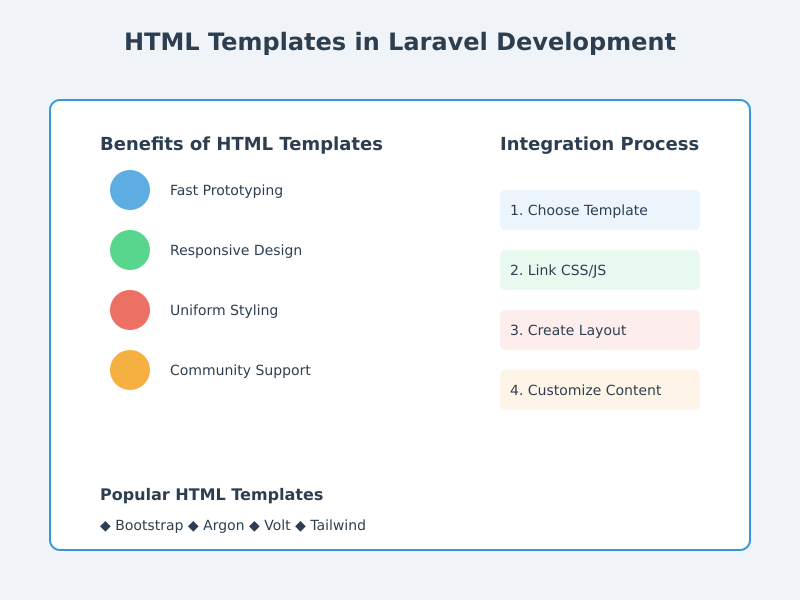
Structuring Your Laravel Project with HTML Templates
This is where using HTML templates with Laravel comes into play. A common approach is to use the HTML template as a skeleton layout within your application and let Laravel views and controllers take over for dynamic content and functionality. In any case, this abstraction lets you use the great HTML template style/pieces while benefiting from the Laravel outlines energy and flexibility
Integrating HTML Templates with Laravel
Before getting started let us be clear that embedding HTML templates in Laravel is a very simple process. In this tutorial, you’ll learn how to set up a default Laravel project and integrate an HTML template into that workflow:
<!-- resources/views/layouts/app.blade.php -->
<!DOCTYPE html>
<html lang="{{ str_replace('_', '-', app()->getLocale()) }}">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>My Laravel App</title>
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/bootstrap@5.1.3/dist/css/bootstrap.min.css">
</head>
<body>
<nav class="navbar navbar-expand-lg navbar-dark bg-primary">
<div class="container">
<a class="navbar-brand" href="#">My Laravel App</a>
<button class="navbar-toggler" type="button" data-bs-toggle="collapse" data-bs-target="#navbarNav" aria-controls="navbarNav" aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<div class="collapse navbar-collapse" id="navbarNav">
<ul class="navbar-nav">
<li class="nav-item">
<a class="nav-link" href="#">Home</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#">About</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#">Contact</a>
</li>
</ul>
</div>
</div>
</nav>
<div class="container my-5">
@yield('content')
</div>
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.1.3/dist/js/bootstrap.bundle.min.js"></script>
</body>
</html>
<!-- resources/views/home.blade.php -->
@extends('layouts.app')
@section('content')
<h1 class="mb-4">Welcome to my Laravel App</h1>
<p class="lead">This is where the main content of your application goes.</p>
<button class="btn btn-primary">Click me</button>
@endsection
In this case, we are preparing a simple Laravel application and a layout file (resources/views/layouts/app. blade. In your common layout (usually `index. It will allow using the Bootstrap components and utilities all over our application.
In the home. blade. php view Л Back to the layouts -- we extend layouts. The app layout is done using Bootstrap classes to style the content. So, we can use classes like mb-4 for the bottom margin on the heading, lead for the paragraph text style, and for the button we can use btn, btn-primary classes.
Using an HTML template with its pre-defined navigation, hero section, and other components will enable you to quickly build attractive user interfaces for your Laravel applications. It ensures you can design a consistent and responsive layout across devices and screen sizes while allowing you to concentrate on the core purpose of the app.
<!-- resources/views/layouts/app.blade.php -->
<!DOCTYPE html>
<html lang="{{ str_replace('_', '-', app()->getLocale()) }}">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<title>My Laravel App</title>
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/argon-design-system-free@1.2.0/assets/css/argon.min.css">
</head>
<body>
<nav class="navbar navbar-expand-lg navbar-dark bg-primary">
<div class="container">
<a class="navbar-brand" href="#">My Laravel App</a>
<button class="navbar-toggler" type="button" data-bs-toggle="collapse" data-bs-target="#navbarNav" aria-controls="navbarNav" aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<div class="collapse navbar-collapse" id="navbarNav">
<ul class="navbar-nav">
<li class="nav-item">
<a class="nav-link" href="#">Home</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#">About</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#">Contact</a>
</li>
</ul>
</div>
</div>
</nav>
<div class="container my-5">
@yield('content')
</div>
<script src="https://cdn.jsdelivr.net/npm/argon-design-system-free@1.2.0/assets/js/argon.min.js"></script>
</body>
</html>
<!-- resources/views/home.blade.php -->
@extends('layouts.app')
@section('content')
<div class="row justify-content-center">
<div class="col-lg-6 col-md-8">
<div class="card bg-secondary shadow border-0">
<div class="card-body px-lg-5 py-lg-5">
<div class="text-center text-muted mb-4">
<small>Welcome to my Laravel App</small>
</div>
<form role="form">
<div class="form-group mb-3">
<div class="input-group input-group-alternative">
<div class="input-group-prepend">
<span class="input-group-text"><i class="ni ni-email-83"></i></span>
</div>
<input class="form-control" placeholder="Email" type="email">
</div>
</div>
<div class="form-group">
<div class="input-group input-group-alternative">
<div class="input-group-prepend">
<span class="input-group-text"><i class="ni ni-lock-circle-open"></i></span>
</div>
<input class="form-control" placeholder="Password" type="password">
</div>
</div>
<div class="text-center">
<button type="button" class="btn btn-primary my-4">Sign in</button>
</div>
</form>
</div>
</div>
</div>
</div>
@endsection
In this case, we use the Argon Dashboard HTML template as a base layout for our Laravel application. So we’ve added the CSS and JavaScript files from the Argon template to the app.blade. php layout file so that the Argon components would be available all through our application.
In the home. blade. For the php view, we are extending the layouts. app layout, and styling the content with the Argon template classes. Using the card, bg-secondary, shadow, and border-0 classes to style the login form which is based on a card, and the input-group, input-group-alternative, and input-group-prepend classes to though the style of the form inputs.
By using an HTML framework (like Argon), you decorate your page with user interface elements with a few code lines on Laravel as it prevents you from writing lots of custom HTML and CSS. With this approach, you can spend more time on the functionality of your app, while being able to have a uniform app design that works on a range of devices and screen sizes.
Production advice: I mentioned HTML templates. HTML templates have lots of prebuilt components that span nearly every styling option, you'll want to decide when to use those versus making your own styles. But do not use classes provided by the template extensively because it will cause a messy and rigid codebase. You can create your own reusable components over that HTML template, instead and keep your code clean and maintainable.
FAQs
Can I use HTML templates for Laravel in other PHP frameworks?
Absolutely! HTML templates can be used in any PHP framework as well as with standalone PHP projects. Though the integration process might vary slightly, the key tenets are still the same.
Are HTML templates bad for larger-size projects?
HTML templates are a good use case for scalability. So while it may require a bit of a setup upfront, the dividends later in terms of rapid development, consistent styles, and responsive design would make them well worth it ᅳ, especially for more complicated apps. Irrespective of project size, HTML templates treat web applications (template, module) as modular, making them scalable: Modular, Maintainable web applications.
Final Thoughts
In my case, the HTML template integrating with travel is perfect. Thanks to this powerful features of Laravel which can be matched with HTML template designs, I can develop up-to-date responsive web applications. With the various tips and examples provided in this article, you are on the way to starting your clean and efficient projects with Laravel. That is a very basic look at what you can do with HTML templates in Laravel, but is enough to understand that looking for HTML templates is one of the best things you can do when building your web applications.