Automate Your Business with AI
I have worked on many projects as a web developer including brochure style websites, SaaS(Software-as-a-Service) applications, and many more. Laravel and Its Powerful Template Engines — A Preferred Option for Building Scalable SaaS Platforms In this post, we'll share some tips from Laravel and template engines that will help you harness the power of Laravel, alongside template engines, to create a winning SaaS solution.
So Why Laravel for Your SaaS App?
Laravel is a popular used PHP framework, and it is a powerful and flexible foundation for building modern web applications, including SaaS platforms. Here are several key advantages of using Laravel for SaaS development:
Strong MVC Architecture: Laravel's Model-View-Controller(MVC) architecture allows you to keep a clear separation of concerns in your code, which makes scaling your application and implementing complex business logic much simpler.
Rich Ecosystem: Laravel has a rich ecosystem with a large community behind it that offers various packages, libraries, and tools to speed up your development.
Intuitive Routing and Authentication: The built-in routing and authentication systems within Laravel simplify the process of managing complex user flows and access control mechanisms that are critical to the functioning of SaaS applications.
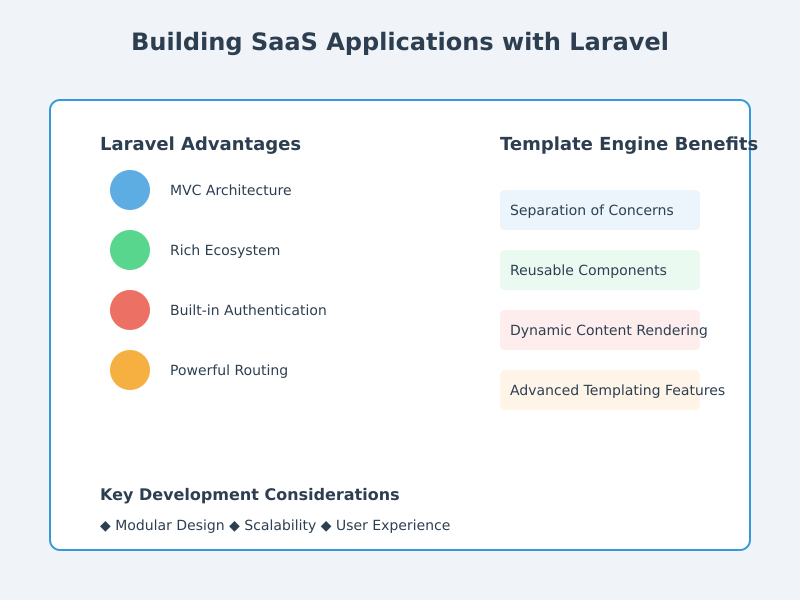
Leveraging Template Engines with Laravel
Laravel itself offers a strong foundation for constructing SaaS applications, but adding an empire of the template can elevate your UI and UX. Template engines, like Blade (the built-in engine for Laravel) or Twig, can provide a number of advantages for SaaS development:
Separation of Concerns: Template engines allow you to cleanly separate your application's presentation from its logic, making your codebase more modular and easier to maintain.
Reusable Templates: The use of template engines allows you to define reusable UI components, which can be easily integrated into different areas of your SaaS application, ensuring consistency and reducing development effort.
Dynamic Content Rendering: Within your UI, template engines allow you to easily incorporate dynamic data, giving your SaaS application the ability to provide real-time information to users.
Additional Templating Features: Many template engines such as Blade and Twig have advanced features that enable auxiliary rendering, loops, and custom helper functions to build complex and sophisticated user interfaces.
SaaS Template Integration in Laravel
For example, let's show you how to integrate a SaaS template with Laravel using the well known Volt SaaS template. Using a SaaS-focused HTML template such as Volt allows you to construct an attractive and functional user interface for your Laravel-based SaaS application in no time.
<!-- resources/views/layouts/app.blade.php -->
<!DOCTYPE html>
<html lang="{{ str_replace('_', '-', app()->getLocale()) }}">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<title>My SaaS App</title>
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/@fortawesome/fontawesome-free@5.15.3/css/all.min.css">
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/volt-bootstrap-5@1.0.1/dist/css/volt.min.css">
</head>
<body>
<nav class="navbar navbar-dark navbar-theme-primary px-4 col-12 d-lg-flex d-none">
<a class="navbar-brand me-lg-5" href="/">
<img class="navbar-brand-image" src="/img/brand.svg" alt="Logo">
</a>
<div class="d-none d-lg-flex">
<ul class="navbar-nav navbar-nav-hover align-items-lg-center">
<li class="nav-item dropdown">
<a href="#" class="nav-link dropdown-toggle" data-bs-toggle="dropdown" aria-expanded="false">Demos</a>
<div class="dropdown-menu">
<a class="dropdown-item" href="#">Dashboard</a>
<a class="dropdown-item" href="#">Transactions</a>
</div>
</li>
</ul>
</div>
<div class="d-flex align-items-center">
<button class="navbar-toggler d-lg-none collapsed" type="button" data-bs-toggle="collapse" data-bs-target="#sidebarMenu" aria-controls="sidebarMenu" aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
</div>
</nav>
<div class="container-fluid bg-gray-800 pb-4">
@yield('content')
</div>
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.1.3/dist/js/bootstrap.bundle.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/volt-bootstrap-5@1.0.1/dist/js/volt.min.js"></script>
</body>
</html>
In this example, we're using the Volt SaaS template as the base layout for our Laravel application. We've included the necessary CSS and JavaScript files from the Volt template in the `app.blade.php` layout file, making the Volt components available throughout our application.
Using Blade Templates in Laravel
Laravel's built-in Blade templating engine is another powerful tool for building SaaS applications. Here's an example of how you can use Blade templates to create a dynamic user dashboard:
<!-- resources/views/dashboard.blade.php -->
@extends('layouts.app')
@section('content')
<div class="row">
<div class="col-md-6">
<h2>Welcome, {{ auth()->user()->name }}!</h2>
<p>Here's a summary of your account activity:</p>
<ul>
@foreach ($recentActivity as $activity)
<li>{{ $activity->description }} on {{ $activity->created_at->format('Y-m-d') }}</li>
@endforeach
</ul>
</div>
<div class="col-md-6">
<h2>Your Subscription</h2>
<p>You are currently subscribed to the {{ auth()->user()->subscription->plan->name }} plan.</p>
<a href="{{ route('subscription.manage') }}" class="btn btn-primary">Manage Subscription</a>
</div>
</div>
@endsection
By using the Blade template inheritance and control structures in this specific example, we can dynamically create user dashboards. We are showing the user's name, active now and its subscription status, which are all fetched from the application data models.
Production note: Template engines/SaaS Templates have everything regarding styling, but don't forget that you can also build your styles when needed! Like in all templated base classes, do not over-rely on their classes. Refrain from this and instead make your own reusable components that use the building blocks from the template, meaning you can keep your code clean and maintainable.
FAQs
Can I use Laravel and template engines on other PHP frameworks other than simple applications like SaaS?
Absolutely! Laravel and template engines or in our case — Blade or Twig are framework-agnostic; they will work with any other PHP framework or even standalone PHP projects. The incoming integration might be a little different but the principles are still the same.
It is common for people to utilize Laravel and template engines in large-scale SaaS applications.
Yes, Good for large scale SaaS ( when you have Laravel with any of the template engines) You may need to invest some time in the beginning for setting it up, but you will be glad you did when the development speed, maintainability, and scalability pay off, especially in complex SaaS apps. A modular aspect of Laravel combined with the customizable concept of template engines makes it a perfect option for robust, enterprise-grade SaaST applications.
Final Thoughts
Barometric always had its quality pillar in Laravel and using one of the most powerful template engine gives us the specific advantage for building a SaaS applications. With the powerful features of Laravel, as well as the flexibility of a separate template engine, it's been easy to build SaaS platforms that are modern, responsive, and immensely scalable. With the tips and examples here you can start to improve your own SaaS app, and provide your customers an incredible UX!