To establish effective intranet systems, one must understand the organizational workflows and security needs. I want to share my experience building internal platforms that drive efficiency in organizations.
Key Features of Intranet System
The best intranet platform must find the balance between usability and security. By implementing some internal environments, I found some important features that are needed here such as single sign on; very granular audit logs and filterable workspaces by business units. That will include organization-wide tools as well as department-specific functionality.
Personal Experience Note: It is important to have flexible department structures. Organizations often reorganize, and the system needs to readily accommodate department merges, splits, and transfers, while not losing historical records.
Securing Internal Systems Implementation
This makes intranet security a multi-layered challenge. Configured additional security measures, Beyond basic authentication, managing IP restrictions, session management, and activity monitoring is done to prevent unauthorized access to the system. It is important that all sensitive operations are logged correctly and a regular security audit should be performed.
Production Tip: Enforce tough password policies, but do it gracefully Use Password Managers and SSO Solutions to balance Security and Usability
Employee Directory and Organizational Structure
A good employee directory is much more than a contact list. It needs to mirror your organizational structure so that appropriate reporting lines and department relationships are simple to follow. Integration with HR systems preserve accurate and up-to-date employee data.
Core Authentication and Authorization
// app/Models/Employee.php
class Employee extends Authenticatable
{
protected $fillable = [
'employee_id',
'department_id',
'position',
'access_level',
'reporting_to',
'office_location'
];
public function department()
{
return $this->belongsTo(Department::class);
}
public function hasAccess($permission)
{
return $this->roles()
->whereHas('permissions', function($query) use ($permission) {
$query->where('name', $permission);
})->exists();
}
public function getReportingChain()
{
$chain = collect([$this]);
$current = $this;
while ($current->reporting_to) {
$supervisor = Employee::find($current->reporting_to);
$chain->push($supervisor);
$current = $supervisor;
}
return $chain;
}
}
Code Explanation: The Employee model manages basic employee information and relationships, handles department associations, implements permission checking, and maintains organizational hierarchy management.
Personal Experience Note: I started with a very simple role-based approach, but I learned that organizations usually will want more fine-tuned permissions. For organizations with more complex structures, inheritance-based hierarchical permission systems have proved, over time, to be a more flexible solution.
Document Management System
// app/Models/Document.php
class Document extends Model
{
protected $fillable = [
'title',
'file_path',
'category_id',
'department_id',
'access_level',
'version',
'status'
];
protected static function boot()
{
parent::boot();
static::creating(function ($document) {
$document->version = 1;
$document->generateUniqueIdentifier();
});
}
public function trackAccess($employee)
{
DocumentAccess::create([
'document_id' => $this->id,
'employee_id' => $employee->id,
'action' => 'view',
'ip_address' => request()->ip()
]);
}
public function createNewVersion($file)
{
DB::transaction(function() use ($file) {
$this->version++;
$this->file_path = $file->store('documents');
$this->save();
DocumentVersion::create([
'document_id' => $this->id,
'version' => $this->version,
'file_path' => $this->file_path,
'created_by' => auth()->id()
]);
});
}
}
Production Tip: Implement thorough document versioning and access logging. This is important for regulatory, compliance, and auditing purposes. Technically then, it could also be feasible to have a cleanup system to delete all ore old document versions after a few days of inactivity to save up on storage.
Internal Communication Hub
// app/Models/Announcement.php
class Announcement extends Model
{
protected $fillable = [
'title',
'content',
'priority',
'departments',
'expires_at'
];
protected $casts = [
'departments' => 'array',
'expires_at' => 'datetime'
];
public function isRelevantFor(Employee $employee)
{
if (empty($this->departments)) {
return true;
}
return in_array($employee->department_id, $this->departments);
}
public function markAsRead(Employee $employee)
{
AnnouncementRead::firstOrCreate([
'announcement_id' => $this->id,
'employee_id' => $employee->id
]);
}
public function scopeActive($query)
{
return $query->where(function($q) {
$q->whereNull('expires_at')
->orWhere('expires_at', '>', now());
});
}
}
Document Workflow and Approval Systems
Document workflows must be strict enough to guarantee procedures, yet elastic enough to accommodate exceptions. 7600—the recommended approval limits requiring administrative tracking with delegation capabilities to strike a balance between efficiency and oversight.
Organizing Document Management and File Sharing
I have built many such intranet document systems and each time I learned that in URL file management, file storage is just the tip of the iceberg. So, the trick is how do you structure a permissioned and versioned manner of structurally organizing the lifecycle of the work to work with how your company works?
Hierarchies of documents can and should follow organizational structures, so long and wide as the flow of information exists across departments. I use a tag-based and traditional folder structure to keep documents decoupled and avoid duplication. And I want every document to know who owns it and who it is shared with, automatically inheriting from parent folders, but overrideable.
Note: Back in the early stages of my development life, I had designed a strict folder-based structure which started to become difficult to manage when the group expanded. I now use a hybrid approach where I use both folders and tags, as well as powerful search capabilities so users can find their documents in multiple ways and quickly.
And being able to version control things is huge in keeping documents. The system should go a step further and also capture who made the changes, when, and why, instead of simple different iterations. Critical documents are subjected to a check-out system to avoid simultaneous edits, and automatic notifications go out to interested parties when new revisions are uploaded.
Production Tip: Always keep a good backup and recovery process set up for the documents. I find it useful to retain detailed information about response patterns for every document so that you can configure the most useful documents to receive frequent backups and the least useful ones infrequent backups, and so forth.
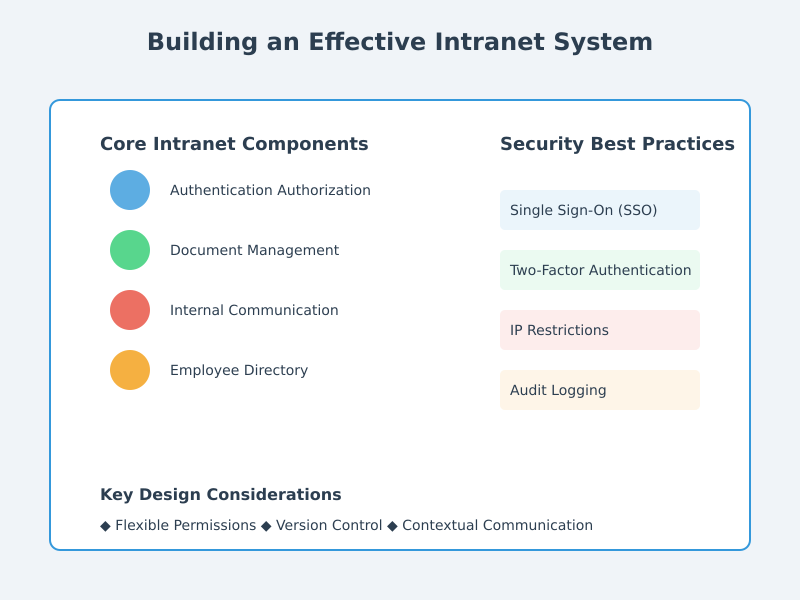
Implementing Internal Messaging and Communication Tools
If there is one thing that every intranet system must have, it has to be great internal communication. In doing so, I have found that successful communication tools are a balance between immediately available and properly organized and searchable communication history.
It should handle direct messages and group communications, as well as smart notification management to avoid information overload. I assign priority levels to messages so that urgent communications can pop out and routine discussions can stay tidy. It should also hook into email notifications for users who aren’t logged into the system actively, but there should be some smart throttling so people don’t get so many notifications that they’re tuned out.
One of the learnings was, communication channel contextuality (more on that in the personal experience note). I no longer have one company-wide chat, but instead have channels that add relevant team members based on projects, departments, or topics, and so people outside of that channel can subscribe manually if they wish.
As conversations scale, managing threads becomes critical. The system should preserve distinct conversational threads while permitting branching discussions where appropriate. Other chatbot uses its own swirling set of smart threading devices, merging related conversations and splitting off-topic conversations into new threads, allowing broad, yet easy-to-follow discussions.
Communication tools contain a lot of data that requires additional care with research — Analysis Find the discussion history, shared files, decisions are easy for the user. I perform full-text search on all messages, with filters for date ranges, participants, or content types. The system remembers context when it displays search results, showing the entire thread of conversation surrounding each matched message.
Production Tip: Archive messages for different types and importance for different lengths of time. Essential communications could be preserved in perpetuity, while routine conversations can be archived after a set time frame. It also ensures that system resources are not utilized unnecessarily, in keeping with data retention regulations.
Integration with other aspects of the intranet is essential - the communication system should integrate smoothly with document management, task tracking and employee directories. If a document comes up in a discussion, the system should be able to pull and check who has access and provide hypertextual links to request access, if needed.
Frequently Asked Questions
How should I handle department-specific content?
Design a generic permission mechanism to accommodate department level as well as role-based permission system. Think about content tagging and dynamic visibility rules.
What's the best way to implement document search?
Full-text search is made with a proper indexation of metadata. Use OCR for scanned documents and version tracking.
How can I ensure system security?
Add layers of security such as SSO, 2FA, IP restrictions, and audit logging. You need to run regular security reviews
Final Thoughts
Creating an efficient intranet involves several technical and organizational aspects. Concentrate on establishing secure and scalable solutions that streamline organizational communication and workflow efficiency.