Automate Your Business with AI
Creating e-commerce platforms in Laravel requires careful attention to both user experience and system robustness. Let me share practical insights from building successful online stores.
Outstanding Features of Laravel E-commerce
To be a successful e-commerce platform, it has to strike a balance between feature richness with performance and maintainability. Based on experience, I have learned that the best approach to launch your product content, discount & promo engines, and shopping cart features, then your checkout flow, your order management features, etc, all of which are core eCommerce functionality, and need to be spot on when thinking about growth. You can add more features as the business grows and based on user feedback.
The system's glue needs to hold everything together and ensure that different paths are followed, whether it be managing stock or processing the payment. Effective error handling and recovery strategies help maintain the integrity and performance of the platform during peak load times or when external services are down.
Creating Your E-commerce App
It is essential to organize the database structure and relationship models when getting started with an e-commerce initiative. Plan for scalability from day one – how will this system cope with thousands of products, hundreds of categories, and pricing rules? This is part of initial configuration such as determining the right structure for indexes or an appropriate caching mechanism to support growth in the store.
Having comprehensive logging and monitoring systems in place from the start is useful for tracking user behavior and system performance. This data is extremely useful to them for optimizing the shopping experience and addressing any potentially problematic issues before they impact sales.
Essential E-commerce Components
// app/Models/Product.php
class Product extends Model
{
protected $fillable = [
'name',
'slug',
'description',
'price',
'sale_price',
'stock_quantity',
'sku',
'status'
];
protected $casts = [
'price' => 'decimal:2',
'sale_price' => 'decimal:2'
];
public function categories()
{
return $this->belongsToMany(Category::class);
}
public function getCurrentPrice()
{
if ($this->sale_price && $this->sale_price < $this->price) {
return $this->sale_price;
}
return $this->price;
}
public function inStock()
{
return $this->stock_quantity > 0;
}
public function decrementStock($quantity)
{
return DB::transaction(function() use ($quantity) {
if ($this->stock_quantity < $quantity) {
throw new InsufficientStockException();
}
$this->decrement('stock_quantity', $quantity);
if ($this->stock_quantity <= $this->reorder_point) {
event(new LowStockEvent($this));
}
});
}
}
Code Explanation: The Product model manages basic product information and pricing, implements stock tracking with safety validations, maintains category relationships, handles price calculations including sales logic, and provides stock management with automated alert systems.
Personal Experience Note: My first approach was to save prices as integers (cents), but that led me to confusion with international currencies. Decimal casting with appropriate precision has been more reliable in a wider variety of currency systems.
Shopping Cart Implementation
// app/Services/CartService.php
class CartService
{
private $cart;
public function __construct(Cart $cart)
{
$this->cart = $cart;
}
public function addItem(Product $product, $quantity = 1, $options = [])
{
if (!$product->inStock()) {
throw new ProductOutOfStockException();
}
$cartItem = $this->cart->items()->updateOrCreate(
[
'product_id' => $product->id,
'options' => json_encode($options)
],
[
'quantity' => DB::raw("quantity + $quantity"),
'price' => $product->getCurrentPrice()
]
);
$this->updateCartTotals();
return $cartItem;
}
private function updateCartTotals()
{
$subtotal = $this->cart->items->sum(function ($item) {
return $item->price * $item->quantity;
});
$this->cart->update([
'subtotal' => $subtotal,
'tax' => $subtotal * config('shop.tax_rate'),
'total' => $subtotal * (1 + config('shop.tax_rate'))
]);
}
}
Production Tip: Always implement cart expiration and cleanup mechanisms. Old abandoned carts can bloat your database. I set up a scheduled task to clear carts older than 7 days that haven't been converted to orders.
Order Processing System
// app/Models/Order.php
class Order extends Model
{
protected $fillable = [
'user_id',
'status',
'payment_status',
'shipping_address',
'billing_address',
'shipping_method',
'payment_method',
'subtotal',
'tax',
'shipping_cost',
'total'
];
public function processPayment()
{
DB::transaction(function() {
try {
$payment = PaymentProcessor::process([
'amount' => $this->total,
'method' => $this->payment_method,
'order_id' => $this->id
]);
if ($payment->successful()) {
$this->update(['payment_status' => 'paid']);
$this->fulfillOrder();
event(new OrderPaidEvent($this));
}
} catch (PaymentException $e) {
$this->update(['status' => 'payment_failed']);
throw $e;
}
});
}
private function fulfillOrder()
{
foreach ($this->items as $item) {
$item->product->decrementStock($item->quantity);
}
$this->update(['status' => 'processing']);
event(new OrderProcessingEvent($this));
}
}
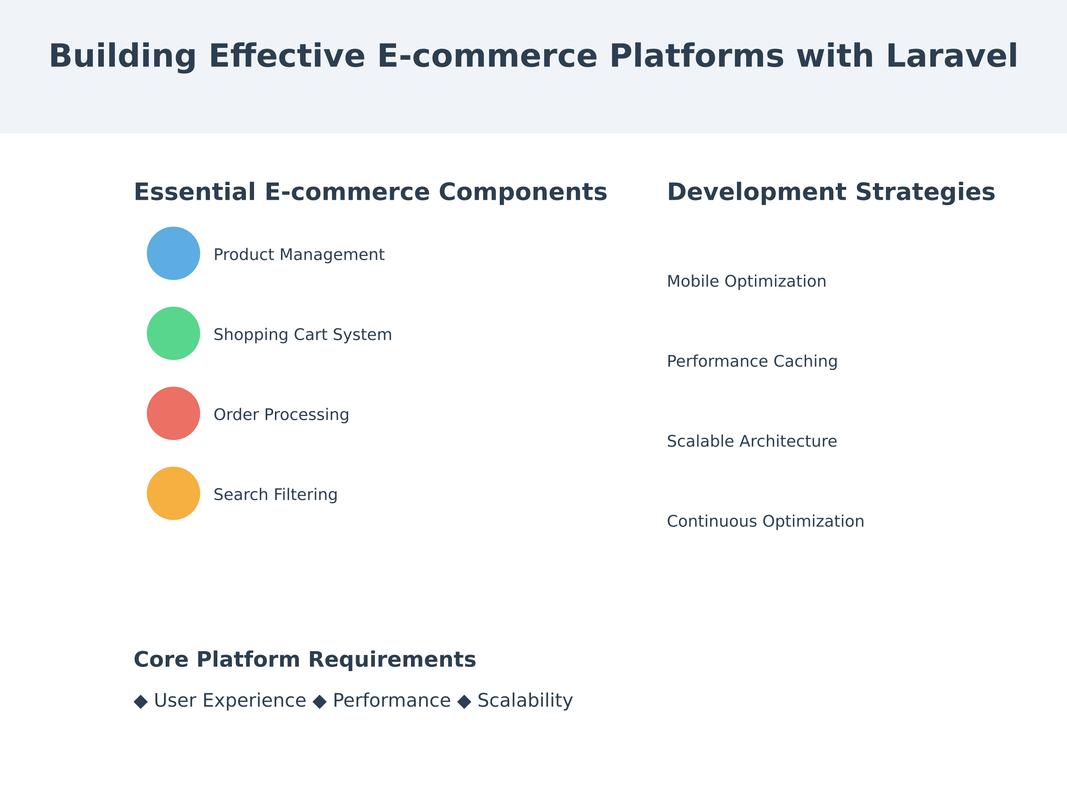
Optimizing Your Ecommerce Website for Mobile Devices
Mobile optimization for e-commerce has become imperative. From many implementations, I have learned that mobile optimization is more than any responsive design. It takes a profound insight into mobile user behavior and shopping trends. The trick is to make sure the experience feels like a mobile version, not just a shrink-wrapped version of the desktop site.
Mobile UI must pay particular care to navigation patterns. For simpler, touch-optimized mega-menus, I create expandable and collapsible category listings. Product filters, and search functions need to be easily accessible yet unobtrusive. One of the more powerful patterns I incorporated was that of the floating filter button that expands to be a full-width overlay when clicked, allowing users to easily filter their products.
Note from Personal Experience: I was once at the stage where my multi-level category navigation looked cool in desktop, but was not at its best in mobile. I now design mobile navigation first and then scale up to desktop to create a smooth experience for all devices.
On mobile devices, image optimization is all the more important. Device capabilities and connection speed determine the size of the images loaded. This includes serving images with the appropriate size and utilizing lazy loading for improved performance.” Initiative for touch-optimized product galleries with swipe gestures and right zoom functionalities.
Mobile users need special consideration when it comes to the checkout process. Form Fields are Mobile Optimized: Input types are set for different fields (email, phone numbers, etc.) Auto-fill support and address validation minimize user friction. I always make sure error messages are un-scrollable.
Production Tip: Always use real payment gateways to test your mobile checkout! Desktop and mobile payment forms can behave very differently, particularly when using third-party payment providers.
Enabling Product Search and Filtering
They need to have a streamlined search and filter functionality to allow customers to find products as quickly as possible. Having worked on multiple e-commerce platforms, it became clear to me how the nuances around search should be both powerful and user-centric. As well as handling misspellings, synonyms, and partial matches, the search system needs to return responses quickly.
Such filtering systems have to be adaptive and contextual. Instead of putting all possible filters at once, I use smart filters where update dynamically according to selected categories or search results. For instance, size filters will only show up for apparel, but technical specifications will appear for electronics, and so on.
A Word of Personal Experience: I started with all filters in the form of straight checkboxes. The fact is - there are different types of attributes that require different types of interfaces – price ranges are best represented by a slider, colors by swatches, and sizes by buttons. Having the UI dynamically change based on the filter type massively boosts user-friendliness.
Search and filtering implementations will be reliant on performance. For filter options and search result sets I use aggressive caching, and invalidate the cache when products change. For large catalogs, using elastic search or similar search engines is highly effective in keeping response times lower.
How the presentation of search results is carefully controlled Hyperlinks to Websites. Users need to easily and quickly scan results, so I use clear product cards with the important information up front — image/title/price/key features. Sorting options should be accessible to the users and remember the user's choice in the session.
Production Tip: Tracking search queries and filters applied. Follow public profiles or accounts on how to implement this. This data is useful for gaining insight into user behavior and optimizing your product catalog. When searching for your product returns no results, but still has a decent amount of volume, it often indicates that there may be opportunities for new products or new content.
The search for multilingual stores: Multi-language stores should focus on managing translation for search functionality. I enable search over every language that we support so that all users have access to the products they need. Which means managing character variations and accents correctly.
Frequently Asked Questions
How should I handle product variations?
Designing a flexible and scalable attribute-based system Use JSON columns if the product you need to implement has dynamic attributes, and set correct validation rules for each product type.
What's the best way to manage shipping options?
Design a modular shipping system that plugs into various carriers. Utilize caching for shipping rate computations, and use zone-oriented shipping regulations.
How can I optimize the checkout process?
First one-step checkout with validation on all the steps. User preferences for repeat customers, and guest checkout options
Final Thoughts
This when done successfully will take a proper e-commerce approach, where you focus on technical side of things as well as a user perspective. Build honest, scalable systems with a seamless shopping experience.
Keep in mind that e-commerce platforms are never done – they need constant monitoring, updates and optimizations according to user behavior and business priorities.